Best Practices For Performance Optimization React.js
- Software Development
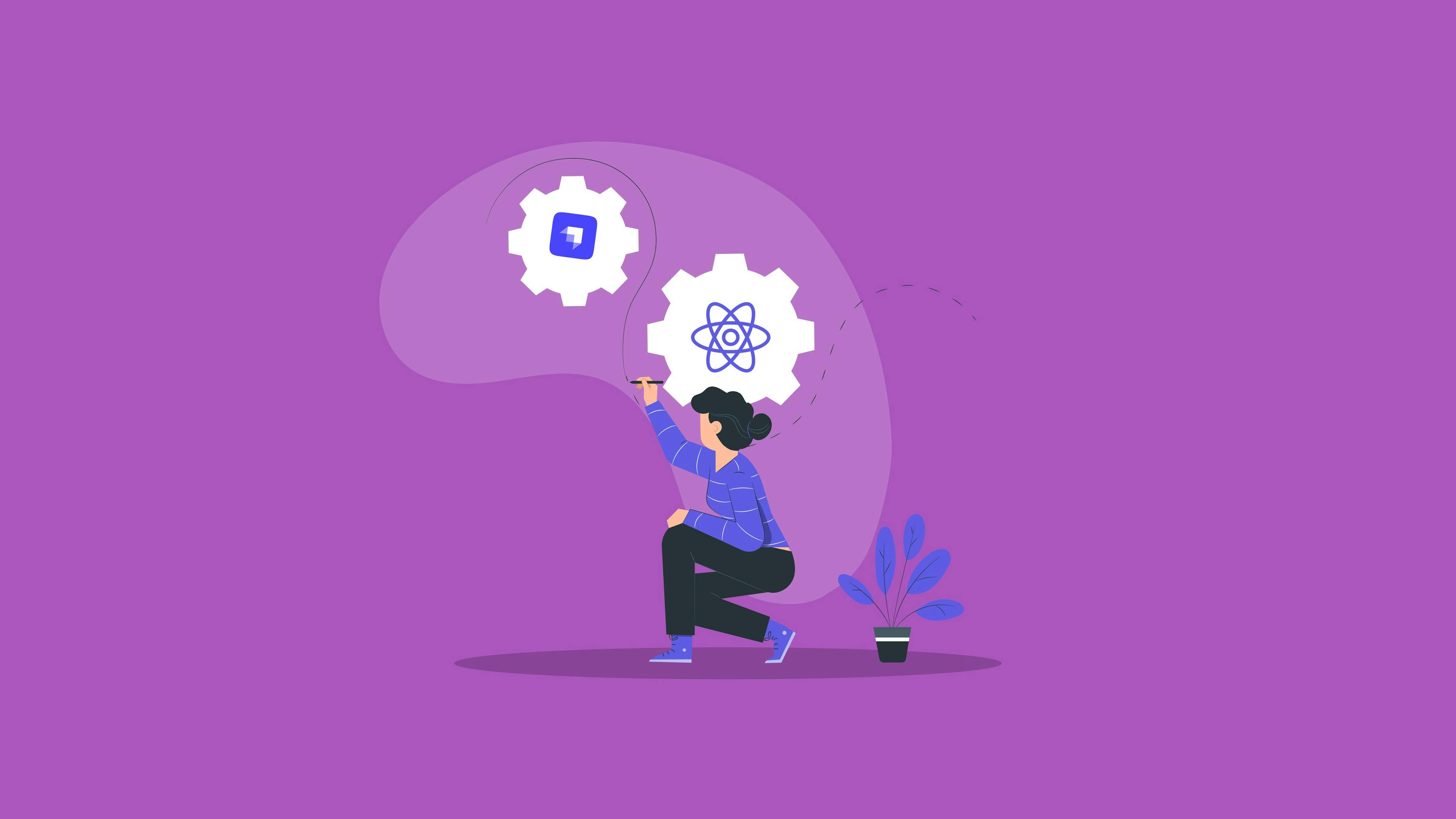
Regarding building performant and efficient web applications, React.js has become a popular choice among developers. However, as applications grow in complexity, it becomes crucial to optimize their performance to ensure a smooth user experience. In this article, Kapsys will delve into the best practices for performance optimization in React.js, focusing on techniques that improve speed, reduce unnecessary re-renders, and enhance overall efficiency.
Understanding how React Updates its UI
React updates its UI using reconciliation, which involves comparing the previous state of the UI with the new state and determining the minimal changes needed to bring the UI to the desired state. Here's an overview of how React updates its UI:
- Virtual DOM Representation: React maintains a virtual representation of the DOM called the Virtual DOM. It's a lightweight copy of the actual DOM that React uses to perform calculations and comparisons efficiently.
- Component Rendering: React components are responsible for rendering UI elements. When a component's state or props change, React invokes its render method to generate a new Virtual DOM representation of the component and its child components.
- Diffing: Once the new Virtual DOM is generated, React compares it with the previous Virtual DOM snapshot to identify the differences between the two. This process is known as diffing or reconciliation.
- Update Strategy: React employs a reconciliation algorithm to determine the most efficient way to update the actual DOM based on the identified differences. It attempts to minimize the number of DOM manipulations needed by applying updates only to the changed parts of the UI.
- Reconciliation: React creates a list of changes or updates to be applied to the actual DOM during reconciliation. It optimizes the process by grouping related changes together and performing batch updates.
- DOM Updates: Once the list of updates is ready, React applies the changes to the actual DOM, efficiently modifying only the necessary parts. This process typically uses "diffing" or "patching" the real DOM.
- Component Lifecycle Methods: Throughout this process, React provides a set of lifecycle methods that allow developers to hook into different stages of the update process. These methods, such as componentDidUpdate, shouldComponentUpdate, and componentWillUnmount, enable developers to customize the behavior of their components during updates.
By leveraging the Virtual DOM and a smart reconciliation algorithm, React minimizes the number of DOM manipulations needed and provides a highly efficient way to update the UI in response to changes in the application state.
Examining Diffing and Re-rendering
Diffing and re-rendering are techniques used in web development to efficiently update the user interface (UI) when changes occur in an application's underlying data or state. These techniques are commonly employed in frameworks like React, Vue.js, and Angular to optimize UI updates and improve performance.
Diffing:
Diffing, short for "difference checking," involves comparing the previous and current states of the UI to determine the minimal set of changes required to update the UI efficiently. Instead of re-rendering the entire UI from scratch, the diffing algorithm only identifies specific elements or components that have changed and applies targeted updates to those parts.
The diffing process typically follows these steps:
- Generate a virtual representation of the UI (often called a virtual DOM or VDOM) based on the current state of the application.
- Compare the previous and current virtual DOM trees to identify differences between them.
- Calculate the minimum set of operations needed to transform the previous virtual DOM into the current one.
- Apply the necessary updates to the real DOM to reflect the changes.
By performing a fine-grained comparison and updating only the necessary elements, diffing minimizes the amount of work required to update the UI, resulting in improved performance and responsiveness.
Re-rendering:
Re-rendering refers to updating the UI by rendering the entire component or section whenever a change occurs in the underlying data or state. Unlike diffing, re-rendering doesn't involve analyzing the differences between previous and current states. Instead, it replaces the existing UI with a fresh rendering based on the new data.
Re-rendering can be less efficient than diffing because it discards the existing UI and rebuilds it entirely, even if only a small portion of the UI needs to change. However, re-rendering may still be suitable for smaller applications or cases where the overhead of diffing outweighs the benefits.
Frameworks like React use a combination of both techniques. They perform virtual DOM diffing to identify granular changes within components and minimize updates to the real DOM. However, if the diffing process becomes computationally expensive or the component tree is simple, React may re-render the entire component.
Both diffing and re-rendering have their trade-offs, and the choice between them depends on factors like the complexity of the UI, the size of the component tree, the frequency of updates, and the application's performance requirements.
Profiling The React App To Locate Bottlenecks
Profiling a React app can help you identify performance bottlenecks and optimize your application. Here's a general approach to profiling a React app:
- Set up a development environment: Ensure you have a development environment with the necessary tools and dependencies. You may want to use tools like Node.js, npm (or yarn), and a code editor.
- Enable production mode: Build your React app in production mode to enable optimizations and better performance. You can run the appropriate build command for your setup (e.g., npm run build).
- Use performance profiling tools: React provides built-in performance profiling tools that can help identify performance bottlenecks. The main tool is the React DevTools Profiler, which integrates with the browser's developer tools. Install the React DevTools extension for your preferred browser.
- Identify components to profile: Determine which components or sections of your app you want to profile. Start with areas you suspect may have performance issues or render frequently.
- Instrument the components: Wrap the components you want to profile with the Profiler component provided by React. The Profiler component accepts a id prop to identify the component and a onRender callback triggered whenever the component is rendered. Inside the onRender callback, you can log or capture performance data.
Example usage of Profiler:
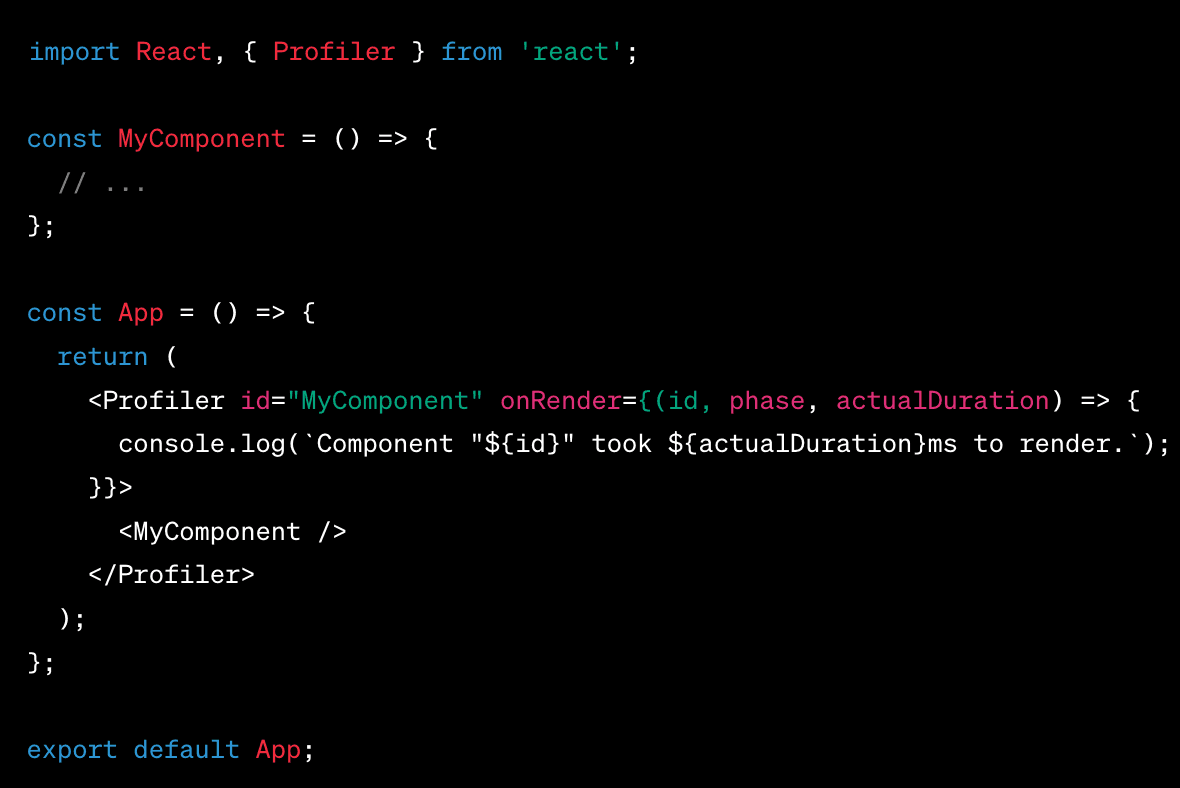
- Analyze the profiling data: Use the React DevTools Profiler to inspect the recorded performance data. Open the browser's developer tools, navigate to the "Profiler" tab, and start recording. Interact with your app to trigger the rendering of the instrumented components. Once you've collected enough data, stop recording and analyze the results.
- Identify bottlenecks: Look for components with significant rendering times or rendered more frequently than expected. Pay attention to the "Actual Duration" metric, which indicates the time to render the component and its descendants.
- Optimize performance: Once you've identified the performance bottlenecks, optimize those components or sections of your app. Consider techniques like memoization, lazy loading, component splitting, or using shouldComponentUpdate/React.memo to prevent unnecessary renders. Make incremental changes and profile again to evaluate the impact of optimizations.
Remember to profile your app in different scenarios, such as loading data, handling large lists, or complex user interactions. This will help you identify performance issues and ensure a smooth user experience.
You can also consider using other performance profiling tools like the Chrome Performance tab or third-party libraries like the React Profiler API, which offer more advanced profiling capabilities.
React Performance Optimization Techniques
Optimizing the performance of a React application is crucial for delivering a smooth user experience. Here are some performance optimization techniques you can employ:
Use React.memo or shouldComponentUpdate:
- React.memo: Wrap your functional components with React.memo to memoize the component and prevent re-rendering when the component's props haven't changed. This is particularly useful for components that receive the same props but don't need to update.
- shouldComponentUpdate: For class components, implement the shouldComponentUpdate lifecycle method to determine whether a component should re-render based on changes in props or state. By customizing this method, you can prevent unnecessary renders and improve performance.
Employ PureComponent or React.memo for children:
- PureComponent: Use PureComponent for class components to automatically perform shallow prop and state comparisons and prevent re-renders when no changes occur. PureComponent is a subclass of Component that implements shouldComponentUpdate with shallow comparisons for props and state.
- React.memo: Wrap child functional components with React.memo to achieve the same effect as PureComponent. This prevents unnecessary re-renders when the child component receives the same props.
Optimize expensive renders with useCallback and useMemo:
- useCallback: Use useCallback to memoize expensive callback functions to prevent unnecessary re-creations on each render. This is particularly useful when passing callbacks as props to child components.
- useMemo: Utilize useMemo to memoize expensive computations or values derived from props or states. It ensures that the computation is performed only when necessary, preventing redundant calculations.
Lazy load components and routes:
- React.lazy: Use React.lazy to load components, allowing you to split your application into smaller chunks and load them on demand. This can improve the initial load time of your application by deferring the loading of less critical components until they are needed.
- React Router's dynamic import: For code-splitting in React Router, you can use the dynamic import syntax to load routes dynamically when the user navigates to them. This reduces the initial bundle size and speeds up the application's startup time.
Virtualize long lists or large data sets:
- Use libraries like react-window or react-virtualized to virtualize long lists or large data sets. These libraries render only the visible portion of the list, significantly reducing the number of DOM nodes and improving rendering performance.
Analyze and optimize component rendering:
- Use React DevTools Profiler to identify components that are causing performance issues. Look for components with high rendering times or excessive re-renders.
- Optimize expensive rendering operations within components, such as unnecessary calculations or inefficient looping.
- Consider breaking down large components into smaller, more manageable ones, reducing the rendering and update overhead.
Ensure efficient data fetching and management:
- Optimize data fetching by reducing unnecessary requests and optimizing the server-side responses.
- Use pagination or infinite scrolling to limit the data fetched at once.
- Implement data caching and intelligent data management techniques to avoid redundant requests.
Enable production optimizations:
- Build your application in production mode to enable React's production optimizations, eliminating development-specific checks and warnings.
- Minify and compress your JavaScript, CSS, and assets to reduce their size and improve loading times.
Remember to profile and measure the impact of each optimization technique to ensure that it positively affects your application's performance. It's often beneficial to prioritize optimizations based on your application's specific needs and usage patterns.
Conclusion
By implementing these best practices for performance optimization in React.js, you can ensure that your applications deliver a seamless user experience with improved speed and efficiency. Understanding how React updates its UI, profiling for bottlenecks, and employing techniques such as local component state, memoization, code-splitting, virtualization, and lazy loading, will help your React applications perform at their best. Stay vigilant in monitoring and optimizing performance, and continuously update your knowledge to adapt to evolving best practices.tools to