Best Practices for Scalable Next.js Applications
- User Experience
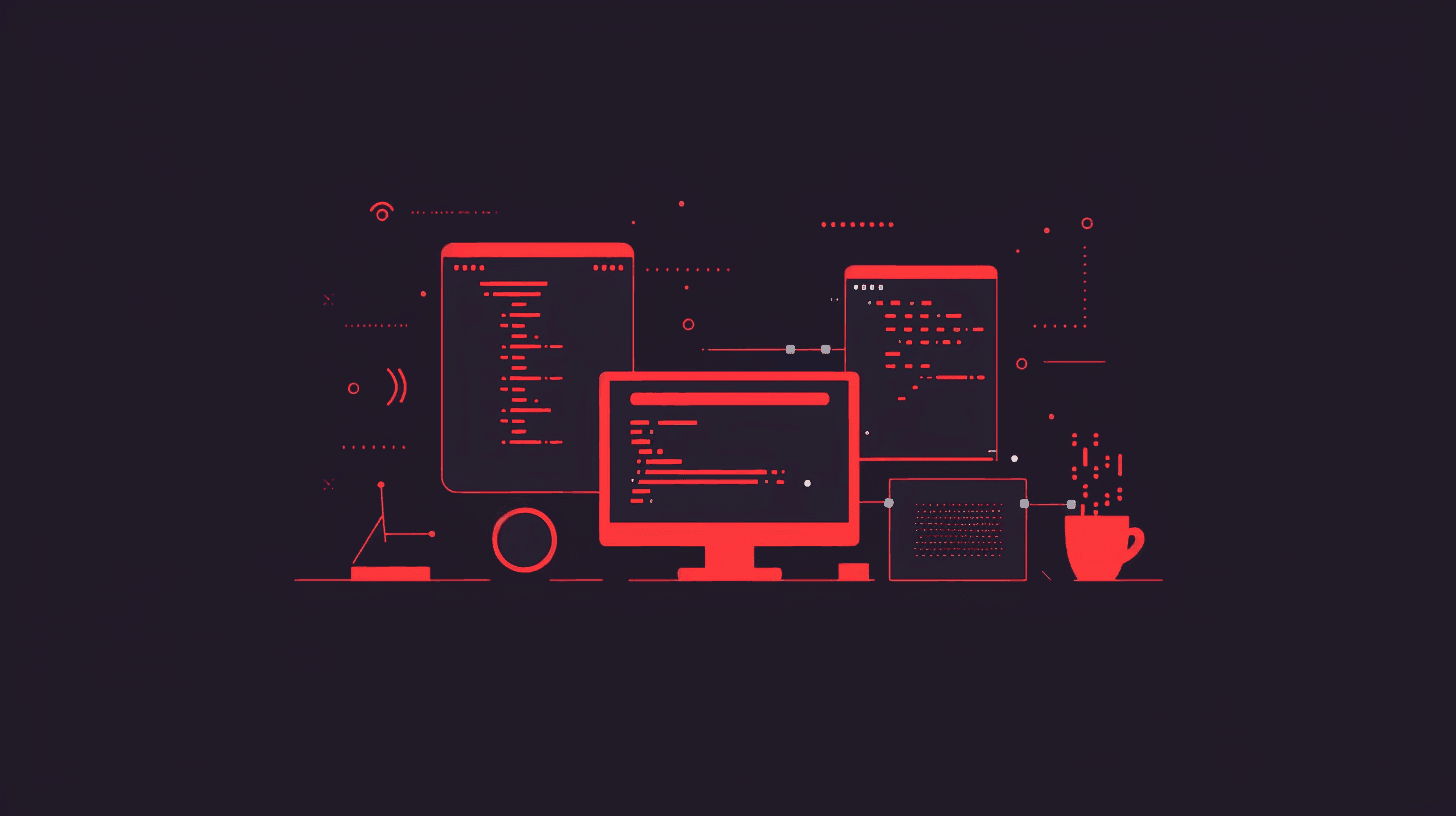
Creating a scalable Next.js web application requires more than a good understanding of React; it necessitates a thorough design, development, and deployment approach.
This article from Kapsys will guide you through the essential practices to ensure your Next js web application meets current needs and is prepared for future growth. We'll explore application insights, effective routing, and how to structure your project for scalability.
Understanding Next js Web Application for Scalability
Before diving into the practices, it's crucial to understand why Next.js is chosen for scalable web applications.
Next.js offers server-side rendering (SSR), static site generation (SSG), and the capability to incrementally regenerate pages (ISR), making it a versatile framework for any next js web application.
Its seamless integration with various backends and ability to prerender pages for performance is unparalleled.
Key Features for Scalability
- Automatic Code Splitting: Load only the necessary modules for each page, reducing the initial load time.
- Hybrid Rendering: Choose the most appropriate rendering strategy (SSR, SSG, ISR) based on the page's needs.
- API Routes: Easily build API endpoints within your Next.js web application to facilitate server-side operations or as a gateway to other services.
Best Practices for Development with Next.js
Developing scalable web applications with Next.js involves understanding its core features, adhering to best practices in coding and architecture, and leveraging the ecosystem's tools effectively. Here are essential development practices to ensure your Next.js projects are scalable, maintainable, and efficient.
Embrace the Framework's Conventions
- File-based Routing: Utilize Next.js's file-based routing to structure your pages. It simplifies navigation within your next js web application and makes it more accessible to developers new to the project.
- API Routes: Leverage Next.js API routes for building your backend API within the same project. This keeps your next js web application consolidated and simplifies deployment and scaling.
Optimize Performance from the Start
- Server-side Rendering (SSR) & Static Generation (SSG): Use SSR for pages that need real-time data and SSG for pages that can be pre-rendered at build time. This significantly improves load times and SEO.
- Image Optimization: Use the
next/image
component for image optimization. It automatically optimizes image loading for different devices and supports lazy loading out of the box. - Code Splitting: Next.js automatically splits your code into manageable chunks. However, be mindful of how you import modules and components to ensure you're not unnecessarily loading large libraries on initial page loads.
Develop with Components and Hooks
- Reusable Components: Break down UI elements into reusable components. This not only makes your application more maintainable but also helps in ensuring consistency across the UI.
- Custom Hooks: Create custom React hooks for complex stateful logic and data fetching. This keeps your functional components clean and promotes code reuse.
State Management and Data Fetching
- Context API/Redux/Zustand: Choose a state management library that fits the size and complexity of your next js web application. For simpler apps, React's Context API might suffice. For more complex state management, consider using Redux or Zustand.
- SWR/React Query: For data fetching, caching, and synchronization, libraries like SWR or React Query can simplify handling asynchronous data and improve user experience by reducing loading times and avoiding waterfalls.
Testing and Quality Assurance
- Unit Testing: Implement unit tests for your components and utility functions. Libraries like Jest and React Testing Library provide a robust testing framework that integrates well with Next.js.
- End-to-End Testing: Use frameworks like Cypress or Playwright to test user flows and interactions. These tests can catch issues that unit tests might miss and ensure your application works as expected from a user's perspective.
Code Quality and Maintenance
- ESLint and Prettier: Integrate ESLint and Prettier into your development workflow for consistent code styling and to catch common errors early in development.
- Type Safety with TypeScript: Adopt TypeScript to add type safety to your project. It can significantly reduce runtime errors and improve the developer experience through better autocompletion and code navigation.
Scalable Project Structure
- Folder Organization: Organize your project files into folders based on features or functionality. This modular approach makes managing and scaling your codebase easier as your application grows.
- Environment Variables: Use environment variables for configuration settings that vary between environments (development, testing, production). This is crucial for securing sensitive information and for flexibility in deployment.
Continuous Integration and Deployment
- Automate Testing and Deployment: Set up continuous integration (CI) pipelines to run tests automatically on each commit. Continuous deployment (CD) pipelines can automate the deployment process, ensuring your anext js web application is always up to date and reducing manual errors.
Advanced Routing Techniques in Next.js
Next.js offers a powerful and intuitive routing system designed to support applications of any scale with simplicity and efficiency. Beyond the basic static and dynamic routes, Next.js enables developers to implement advanced routing techniques to enhance UX, optimize performance, and improve the overall structure of the application. Here are some advanced routing techniques you can leverage in your Next.js projects.
Custom Server and Rewrites
- Custom Server: By implementing a custom Node.js server, you gain full control over the routing logic. This is particularly useful for handling legacy routes, server-side computations before rendering, or custom proxy behavior. However, note that using a custom server might limit the benefits of static optimization provided by Next.js.
- Rewrites: Next.js allows you to rewrite paths to different destinations without changing the URL shown to the user. This feature is handy for creating user-friendly URLs or routing to external resources as if they were part of the next js web application.
Middleware for Route Handling
Introduced in Next.js 12, middleware enables you to run code before a request is completed directly within Next.js. You can use middleware for:
- Authentication: Check if a user is authenticated and redirect unauthenticated users before rendering the page.
- Logging and Analytics: Implement custom logging for analytics or debugging purposes.
- Custom Headers and Redirects: Modify response headers or perform redirects based on the request path or other criteria.
Middleware supports Edge Functions, running at the edge closer to your users, significantly improving performance and scalability.
Incremental Static Regeneration (ISR)
ISR allows you to update static content after deployment without rebuilding the entire site.
This technique is particularly effective for applications that require frequent updates to content but still want to benefit from the performance of static generation.
With ISR, you can specify a revalidation time to update the cached content, ensuring your users always see the most up-to-date information.
Dynamic Imports for Components
Dynamic imports enable you to split your code into separate bundles that can be loaded on demand. This can significantly reduce the initial load time of your pages. In Next.js, you can use dynamic imports for components like this:
import dynamic from 'next/dynamic';
const DynamicComponent = dynamic(() => import('../components/MyComponent'));
function HomePage() {
return (
<div>
<h1>Welcome to the Home Page</h1>
<DynamicComponent />
</div>
);
}
This technique is especially useful for loading components that are not immediately required on page load, such as modals or off-screen content.
Catch-all Routes and Optional Catch-all Routes
Catch-all routes allow you to capture paths that do not correspond to your static or dynamic routes. This is useful for building applications with many pages that aren't known at build time or for handling user-generated content with unique slugs.
Optional catch-all routes extend this functionality by making the catch-all segment optional, enabling a single route to capture specific paths and a broader pattern.
Custom 404 and Error Handling
Customizing the 404 page or handling errors at the route level can enhance the user experience and maintain consistency in your application's design. Next.js provides a simple way to create a custom 404 page by adding a 404.js
file in the pages directory. For more complex error handling, you can use the _error.js
file to catch and display errors for server-side rendering and static generation.
Localization and Internationalized Routing
Next.js supports automatic internationalized (i18n) routing. You can configure locales next.config.js
and structure your pages to serve different versions based on the user's region or language preference. This native support simplifies creating multi-language applications, handling locale detection, and loading the appropriate content based on the user's preferences.
your application's design consistency
Conclusion
Building a scalable Next.js web application involves a comprehensive approach encompassing project structure, performance optimization, application insights, advanced routing, and strategic deployment.
By following these best practices, you'll be well-equipped to develop a Next js web application that performs excellently and scales efficiently to meet the demands of your growing user base.
As Next.js continues to evolve, staying updated with the latest features and practices will ensure your application remains competitive and scalable.