A Guide To Building a Next.js App with Kontent.ai and Deploying on Vercel
- User Experience
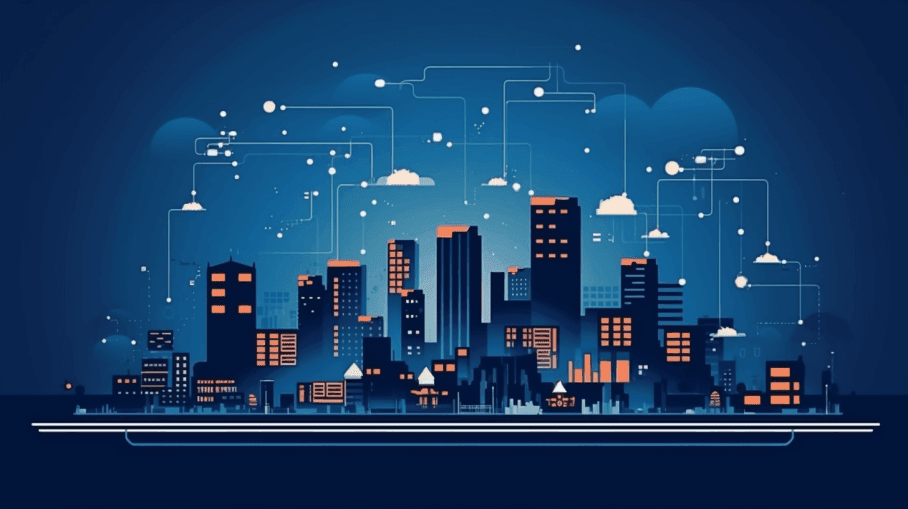
Are you ready to embark on a journey to create a Next.js app that leverages the power of Kontent.ai and deploys it effortlessly on Vercel? Look no further! This comprehensive guide will walk you through the entire process, from setting up your development environment to deploying your Next.js app on Vercel.
Join Kapsys in this guide to build something unique!
Why Next.js?
Before we get into the nitty-gritty of building and deploying our Next.js app, let's briefly explore why Next.js is an excellent choice for web development. Next.js is a React framework that offers numerous advantages, including:
Server-Side Rendering (SSR): Next.js makes SSR a breeze, providing faster page loads and improved SEO.
Efficient Routing: Its file-based routing system simplifies navigation within your app.
Automatic Code Splitting: Optimizes performance by only loading JavaScript when needed.
Vast Ecosystem: Access to a rich ecosystem of packages and plugins for enhanced functionality.
Now that you understand the benefits of Next.js let's dive into the steps to build your Next.js app with Kontent.ai and deploy it on Vercel.
Read: Building a Blog with Next.js and Strapi: End-To-End Guide For A Modern Web Application
Step 1: Setting Up Your Development Environment
The first step is always the most important. So, take your time and set properly your development environment, following these easy pointers:
Install Node.js and npm
Ensure you have Node.js and npm (Node Package Manager) installed on your machine. You can download them from the official website.
Create a new Next.js project
Let's start by creating a new Next.js project. Open your terminal and run the following commands:
npx create-next-app my-nextjs-app
cd my-nextjs-app
This will scaffold a basic Next.js app for you.
Step 2: Integrating Kontent.ai
Kontent.ai integration is a very straightforward process that requires just a few steps:
Sign up for Kontent.ai
If you haven't already, sign up for a Kontent.ai account. Kontent.ai is a headless content management system (CMS) that will help you manage your content seamlessly.
Install the Kontent delivery SDK
To interact with Kontent.ai from your Next.js app, you'll need to install the Kontent Delivery SDK. Run the following command:
npm install @kentico/kontent-delivery
Configure Kontent.ai
You'll need to configure Kontent.ai to connect to your project. Retrieve your Project ID and API Key from your Kontent.ai project settings and create a configuration file like so:
// kontentConfig.js
module.exports = {
projectId: 'YOUR_PROJECT_ID',
apiKey: 'YOUR_API_KEY',
};
Now, you can use this configuration to fetch content from Kontent.ai in your Next.js app.
Step 3: Building Your Next.js App
With Kontent.ai integrated into your Next.js project, it's time to build your app. Create React components and pages, and use the Kontent Delivery SDK to fetch content dynamically.
Here's a simple example of fetching content:
// pages/index.js
import React from 'react';
import { DeliveryClient } from '@kentico/kontent-delivery';
import kontentConfig from '../kontentConfig';
const client = new DeliveryClient(kontentConfig);
export async function getStaticProps() {
const response = await client.items().type('your_content_type').toPromise();
const content = response.items;
return {
props: {
content,
},
};
}
function Home({ content }) {
return (
<div>
{/* Render your content here */}
</div>
);
}
export default Home;
Customize the above code to suit your content types and requirements.
Step 4: Testing Locally
Before deploying to Vercel, testing your Next.js app locally is essential. Run the following command to start your development server:
npm run dev
Open your browser and navigate to http://localhost:3000 to see your app in action.
Step 5: Deploying Your Next.js App on Vercel
Now, let's deploy your Next.js app to Vercel, a popular platform for hosting web applications. Before you begin any deployment, you need to understand that it's required to have an established Vercel account. So, take your time to either login or sign up for Vercel.
Install Vercel CLI
Install the Vercel CLI globally by running the following:
vercel
Follow the prompts to link your Vercel account, choose a name for your project, and configure deployment settings. Vercel will take care of building and deploying your app.
Read: Best Practices For Safety And Privacy Vercel Deployment
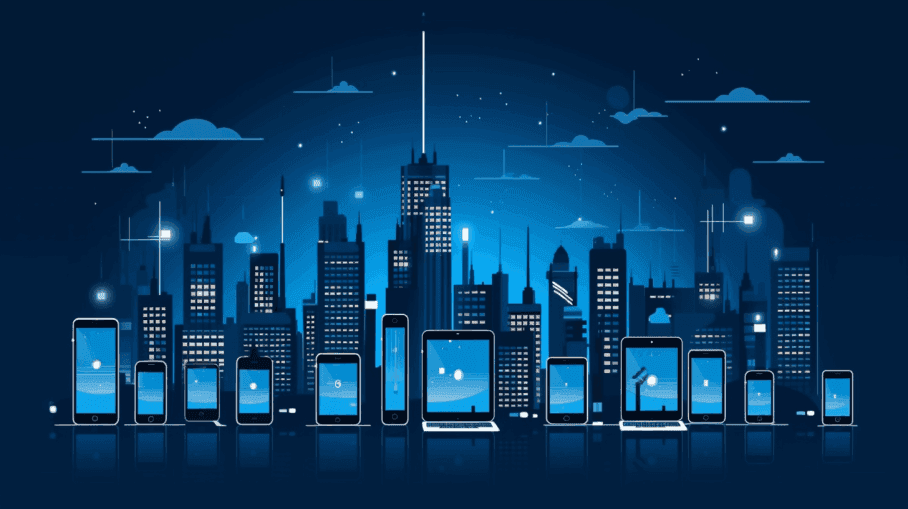
Troubleshooting Common Issues
While building and deploying your Next.js app with Kontent.ai and Vercel is a relatively straightforward process, you may encounter some common issues. Let's take a look at how to troubleshoot these problems effectively.
Deployment errors on Vercel
Issue: If you encounter errors during the deployment process on Vercel and your app fails to go live.
Solution:
Check Your Configuration: Ensure that your Vercel configuration is correctly set up. Double-check the project settings, including environment variables, deployment branches, and build settings.
Review Error Logs: Vercel provides detailed error logs during the deployment process. Review these logs to identify the issues that caused the deployment to fail. Look for any error messages or stack traces.
Dependencies: Ensure your project's dependencies are correctly listed in your package.json file. It's possible that a missing/ outdated dependency could be causing issues.
Content not loading from Kontent.ai
Issue: Your Next.js app is not fetching content from Kontent.ai as expected.
Solution:
Check API Key and Project ID: Verify that your Kontent.ai API key and project ID are correctly configured in your kontentConfig.js file. Typos or incorrect values can prevent successful communication with Kontent.ai.
Content Type Configuration: Ensure that your content types in Kontent.ai match the style you're trying to retrieve in your Next.js app. Mismatched content types can lead to empty or missing content.
Rate Limiting: Kontent.ai may have rate limits in place. If you're making many API requests in a short period, you could encounter rate-limiting issues. Consider implementing caching or optimizing your requests to reduce the load on the Kontent.ai API.
Performance issues
Issue: Your Next.js app needs to load faster or experience performance issues.
Solution:
Code Splitting: Ensure you use code splitting effectively in your Next.js app. Only load JavaScript that is necessary for the current page to improve performance.
Optimize Images: Images can significantly impact page load times. Use optimized images and consider lazy loading or responsive image techniques to reduce the initial load.
Vercel Build Settings: Review your Vercel build settings and ensure your app is assembled and deployed efficiently. Utilize serverless functions for backend tasks to improve performance.
Local development issues
Issue: You need help with testing your Next.js app locally.
Solution:
Check Dependencies: Ensure all dependencies are installed correctly by running npm install or yarn install in your project directory.
Environment Variables: If you use environment variables, ensure they are set correctly for local development. You can use tools like dotenv to manage these variables locally.
Check for Port Conflicts: Ensure that the port your local development server is using (usually 3000 by default) is not in use by another application. You can change the port in your Next.js configuration if necessary.
SEO and metadata
Issue: Your Next.js app must display the correct metadata or SEO information.
Solution:
Check Head Tags: Verify that you have set up the appropriate meta tags for SEO in your Next.js components. Ensure you use the correct React Helmet or Next.js Head components to manage these tags.
Cache and CDN: If you've recently updated your metadata, it may take some time for changes to propagate, especially if you're using a Content Delivery Network (CDN). Clear any caches and wait for the changes to reflect.
Structured Data: Consider implementing structured data markup using JSON-LD or other formats to improve SEO and search engine visibility.
Remember that troubleshooting often involves a process of elimination. Identify the specific issue, review your configurations, and consult the relevant documentation for Next.js, Kontent.ai, and Vercel.
Feel free to seek help from developer communities or support forums if you're stuck on a particular problem. With patience and persistence, you can overcome challenges and build a robust Next.js app that leverages Kontent.ai and deploys smoothly on Vercel.
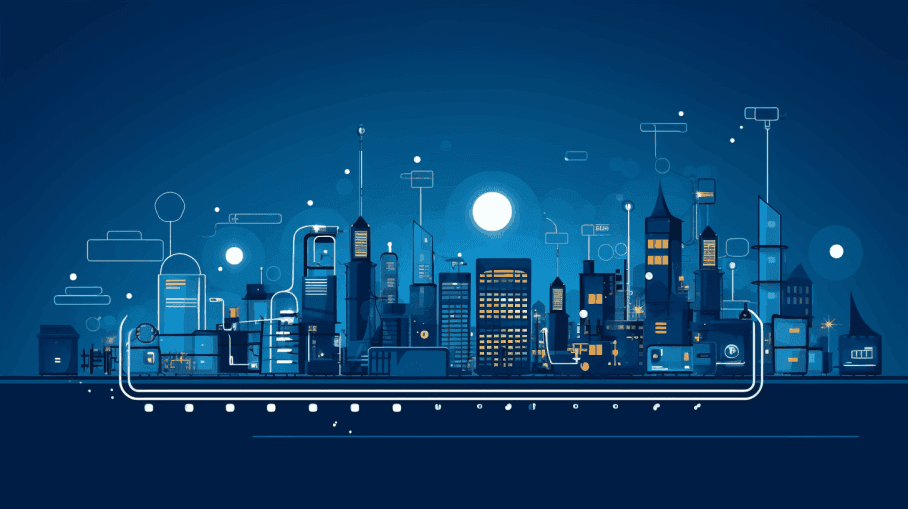
Conclusion
This comprehensive guide has walked you through building a Next.js app, integrating Kontent.ai for content management, and deploying your app seamlessly on Vercel.
With these powerful tools and the knowledge you've gained, you're well-equipped to create dynamic, performant web applications. Explore and experiment with Next.js, Kontent.ai, and Vercel to bring your unique project to life!
Stay ahead in cutting-edge technology and innovation by subscribing to the Kapsys blog – your source for the latest updates, insights, and tutorials!