Dynamic Routing in Next.js: Tips and Tricks
- User Experience
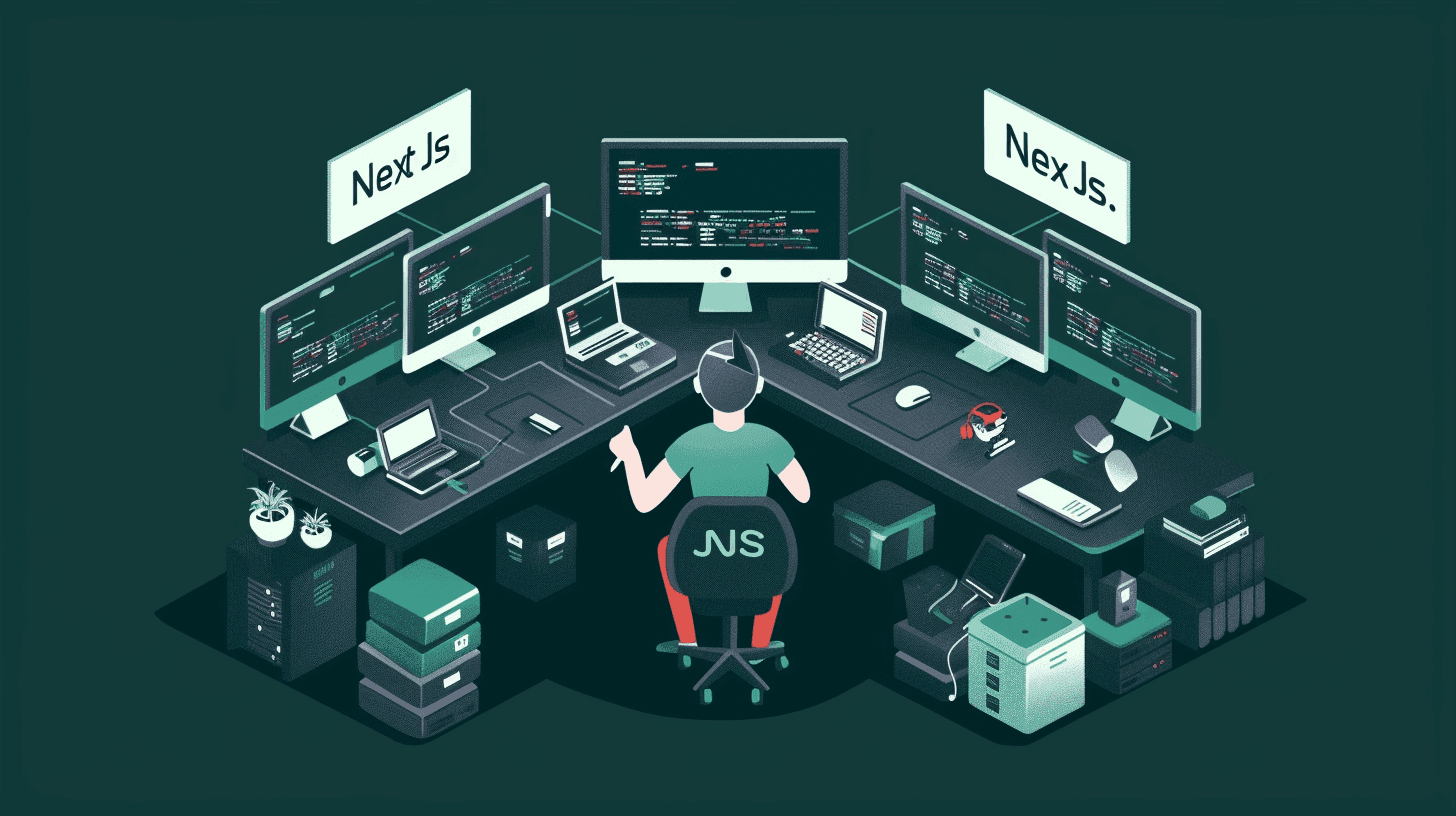
Next.js has revolutionized how web applications are built, offering developers a robust framework that simplifies the creation of fast, scalable projects. Among its many features, dynamic routing stands out as a powerful tool for building complex, user-friendly applications. This article from Kapsys delves into dynamic routing in Next.js, offering tips and tricks to harness its full potential.
What is Next.js?
Before diving into dynamic routing, it's crucial to understand what Next.js is. Next.js is a React-based framework that enables developers to build server-side rendered (SSR) and statically generated web applications. It's designed to make React apps more efficient and easier to scale, providing features like automatic code splitting, optimized prefetching, and more.
What are Dynamic Routes in Next.js?
Dynamic routes allow Next.js developers to create pages that can adapt to changing data, making it possible to generate routes based on user input or external data sources. This feature is particularly useful for applications that require content to be fetched from a database or API, such as e-commerce sites, blogs, or portfolios.
Implementing Dynamic Routing in Next.js
Implementing dynamic routing in Next.js involves a few key steps that allow developers to create flexible and complex applications. Dynamic routes are essential for applications that require content to be fetched based on user input or external data. Here’s a detailed guide on how to implement dynamic routing in Next.js:
Step 1: Creating Dynamic Pages
- File Naming Convention: To create a dynamic page, you need to use square brackets
[]
in the file name to specify a dynamic segment. For example, if you want to create a dynamic route for blog posts, you would create a file named[slug].js
inside thepages/posts
directory. Theslug
part is a variable that will be matched by the path in the URL. - Accessing URL Parameters: Inside your dynamic page, you can access the dynamic parts of the URL using the
useRouter
hook fromnext/router
. For instance, to get theslug
value from the URL, you can use the following code snippet:
import { useRouter } from 'next/router';
const Post = () => {
const router = useRouter();
const { slug } = router.query;
return <div>Post: {slug}</div>;
};
export default Post;
Step 2: Fetching Data for Dynamic Pages
To fetch data based on the dynamic segment, you can use Next.js's data fetching methods like getStaticProps
and getStaticPaths
, or getServerSideProps
depending on your needs.
Using getStaticProps
and getStaticPaths
getStaticProps
: This function fetches data at build time. It receives parameters that include path parameters for dynamic routes.
export async function getStaticProps({ params }) {
const postData = await getPostData(params.slug); // Fetch your data here
return {
props: {
postData,
},
};
}
getStaticPaths
: This function specifies which paths should be pre-rendered. For dynamic pages, you need to define a list of paths that have to be generated at build time.
export async function getStaticPaths() {
const paths = await getAllPostSlugs(); // Fetch or define your paths here
return {
paths,
fallback: false, // Can be set to 'blocking' or 'true' for incremental static regeneration
};
}
Using getServerSideProps
getServerSideProps
: For pages that require fresh data for each request (e.g., user-specific pages), usegetServerSideProps
. This function runs on each request and can fetch data that is passed to the page as props.
export async function getServerSideProps({ params }) {
const userData = await getUserData(params.id); // Fetch your data here
return {
props: {
userData,
},
};
}
Step 3: Linking to Dynamic Routes
To link to dynamic routes within your Next.js application, use the Link
component from next/link
and pass the dynamic path as the href
property:
import Link from 'next/link';
const HomePage = () => (
<ul>
<li>
<Link href="/posts/[slug]" as="/posts/hello-world">
<a>Hello World Post</a>
</Link>
</li>
</ul>
);
export default HomePage;
Tips and Tricks for Dynamic Routing
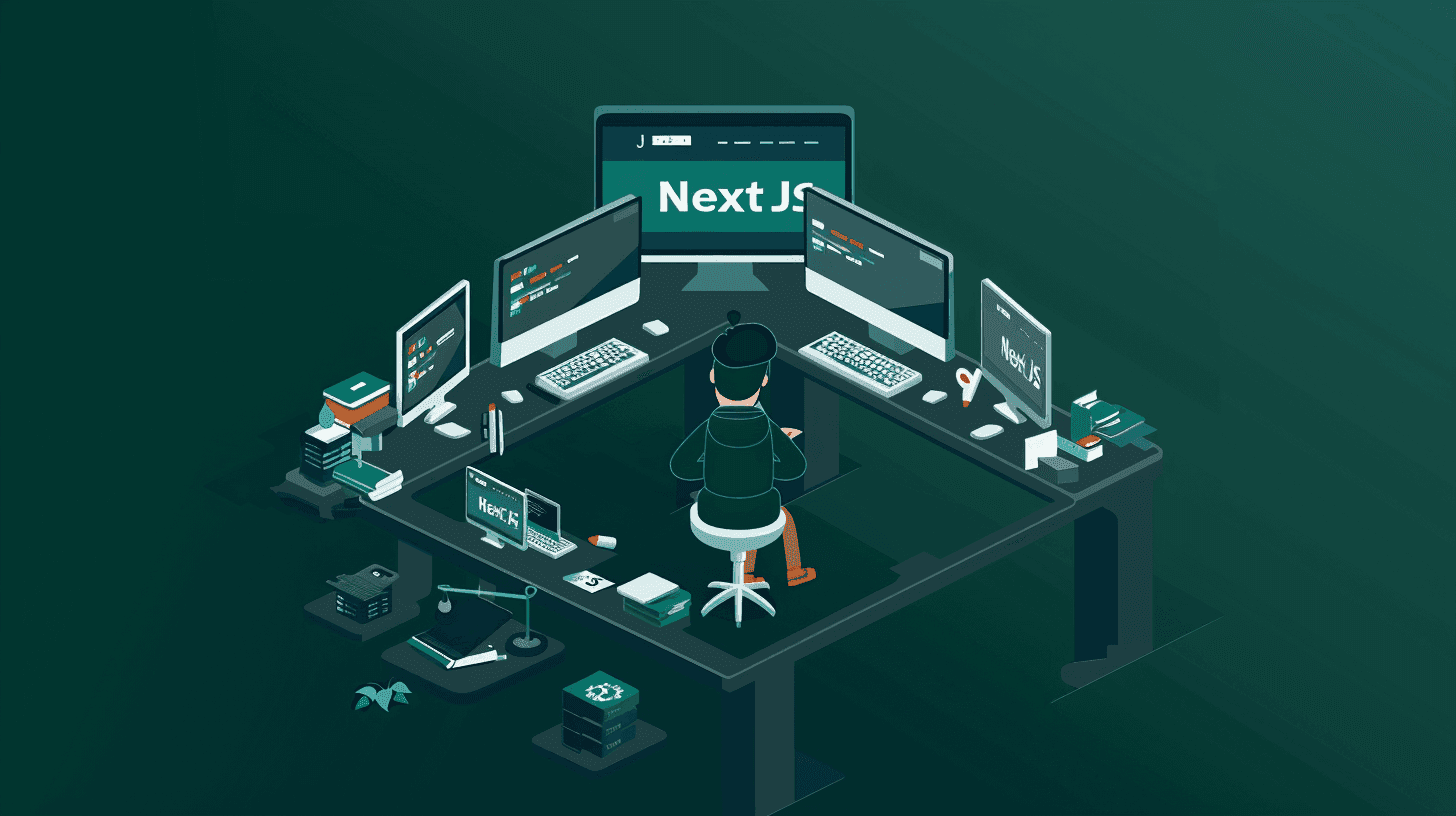
Tip #1: Use getStaticPaths
for Static Generation
For statically generated sites, getStaticPaths
works alongside getStaticProps
to specify which paths should be pre-rendered. This method is essential for dynamic routes, allowing you to define a list of paths that have their HTML generated at build time.
Tip #2: Leverage getServerSideProps
for Server-side Rendering
If your application requires real-time data, getServerSideProps
is the way to go. It renders your page on each request, ensuring that the data is always up to date. This is particularly useful for pages that display user-specific information or results from a database query.
Tip #3: Optimize Dynamic Routes with Automatic Static Optimization
Next.js automatically optimizes pages by statically generating them whenever possible. However, for dynamic routes, consider manually specifying fallback versions or leveraging Incremental Static Regeneration (ISR) to enhance performance without sacrificing dynamic capabilities.
Tip #4: Utilize Rewrites for Cleaner URLs
Next.js's rewrites
feature allows you to map dynamic routes to cleaner, more user-friendly URLs. This can be particularly useful for SEO and improving the user experience by hiding complex query parameters or IDs.
Advanced Techniques
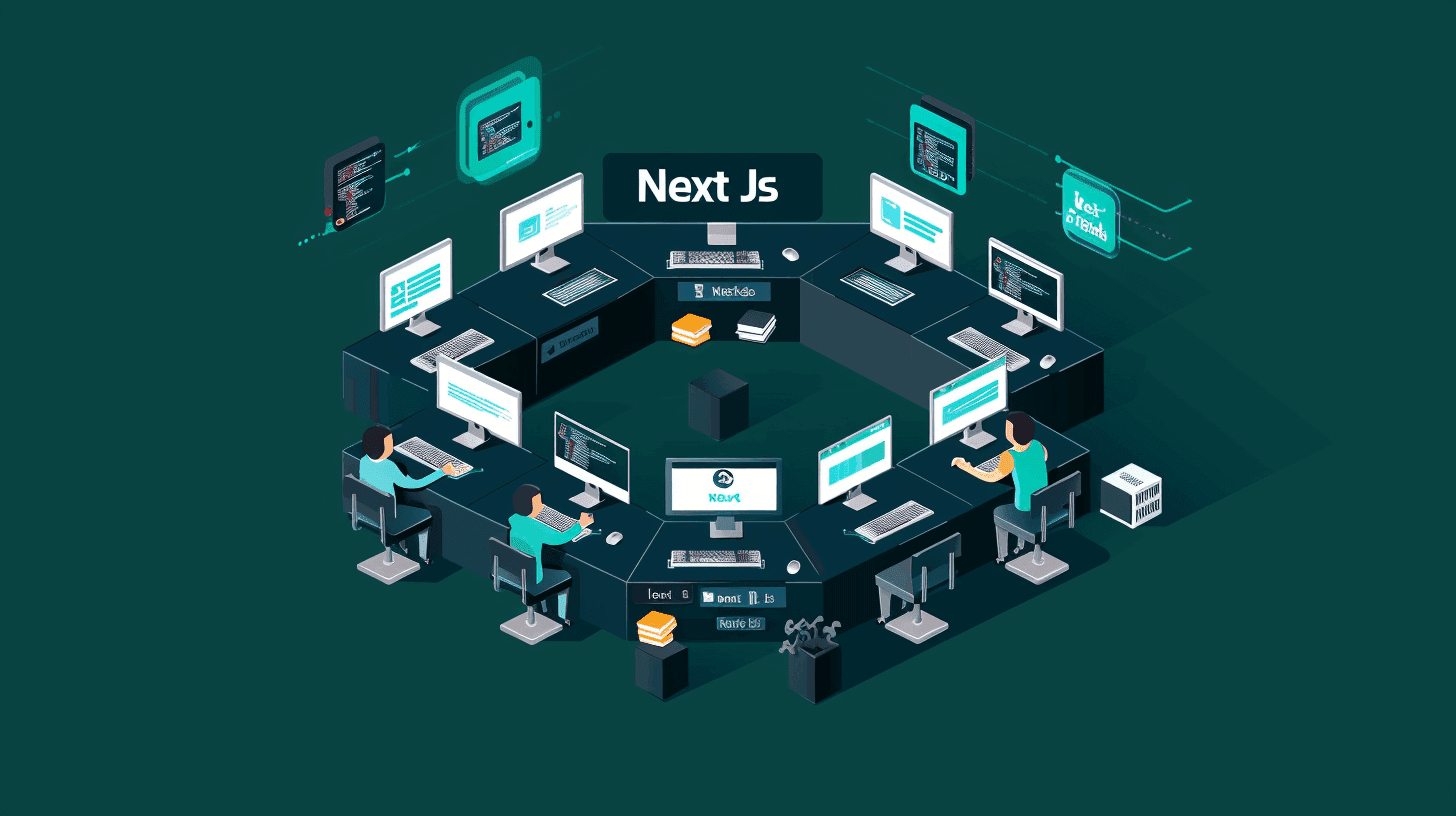
Customizing the 404 Page for Dynamic Routes
Dynamic routes can sometimes lead to user navigation errors. Customizing the 404 page to handle such cases gracefully can improve the user experience significantly.
Dynamic API Routes
Next.js also supports dynamic API routes, allowing you to create API endpoints with dynamic parameters. This can be incredibly useful for building backend functionality directly within your Next.js application.
Conclusion
Dynamic routing in Next.js opens up possibilities for building sophisticated, data-driven web applications. Developers can fully leverage active routes to create more interactive, personalized user experiences by understanding and implementing the tips and tricks discussed in this article. Whether optimizing performance, fetching data efficiently, or creating more user-friendly URLs, the power of dynamic routing in Next.js is a game-changer for modern web development.