Exploring Next.js API Routes for Server-side Operations
- User Experience
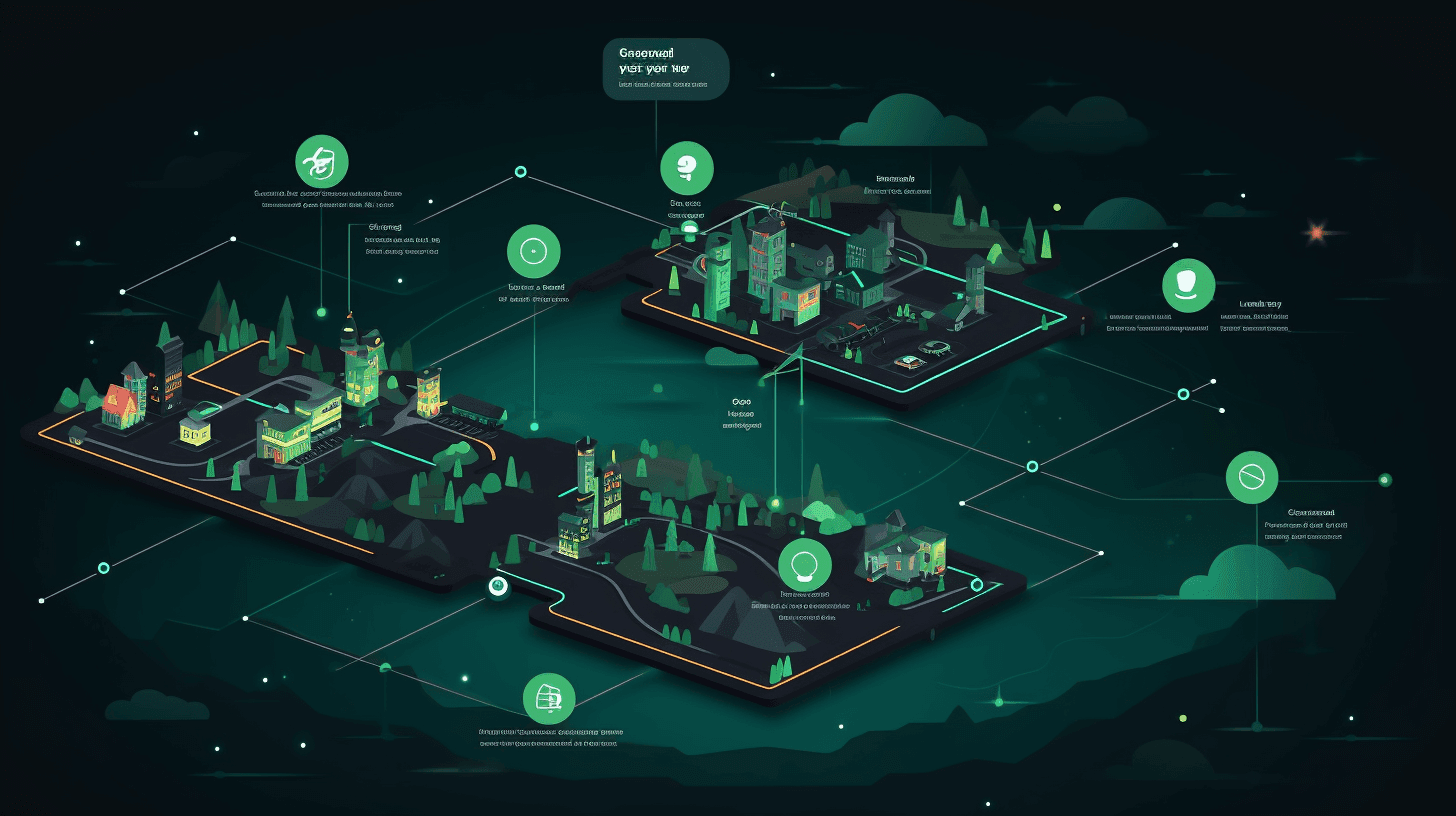
Next.js has rapidly become one of the most popular frameworks for React development thanks to its simplicity, flexibility, and performance. Among its many features, Next.js API routes are a powerful tool for developers looking to handle server-side operations directly within their Next.js applications. This guide from Kapsys will explore the capabilities of Next.js API routes, offering insights and examples to help you fully utilize this feature for your server-side needs.
What are Next.js API Routes?
Next.js API routes allow you to create RESTful API endpoints for your Next.js application. These routes reside in the pages/api
directory, where each file corresponds to an API endpoint. This integration simplifies the development process by allowing you to manage your frontend and API within a single project, eliminating the need for a separate server for backend operations.
Key Features
- Serverless Functionality: Next.js API routes are serverless functions, which means they scale automatically and are only invoked when needed, leading to efficient resource utilization.
- Built-in Middleware Support: Customize API behavior with built-in middleware, enabling tasks like request parsing, CORS handling, and more.
- Easy to Set Up: With no additional configuration required, setting up API routes is straightforward, making it accessible for developers of all skill levels.
Creating and Using Next.js API Routes
Basic Setup
To get started with Next.js API routes, create a file in the pages/api
directory. The file name becomes the endpoint's path. For example, a file named hello.js
would create the route /api/hello
.
// pages/api/hello.js
export default function handler(req, res) {
res.status(200).json({ message: 'Hello World' });
}
This simple function responds to requests to /api/hello
with a JSON object containing a greeting message.
Handling Different HTTP Methods
You can handle different HTTP methods (GET, POST, etc.) within the same API route by checking the req.method
property.
// pages/api/message.js
export default function handler(req, res) {
switch (req.method) {
case 'GET':
res.status(200).json({ message: 'This is a GET request' });
break;
case 'POST':
// Process POST request
res.status(200).json({ message: 'This is a POST request' });
break;
default:
res.setHeader('Allow', ['GET', 'POST']);
res.status(405).end(`Method ${req.method} Not Allowed`);
}
}
Leveraging the Next.js App Router API for Advanced Operations
The Next.js app router API enhances API routes by providing advanced routing capabilities, middleware support, and more. This allows for complex server-side operations, such as user authentication, data processing, and dynamic route handling, directly within your Next.js application.
Best Practices for Using Next.js API Routes
Next.js API routes offer a robust solution for integrating server-side logic directly into your Next.js applications. Following best practices, developers can ensure their API routes are efficient, secure, and maintainable. Here are some key best practices for using Next.js API routes:
1. Organize Your API Files Logically
- Directory Structure: Place your API route files within the
pages/api
directory. Use a logical directory structure to group related endpoints, which makes your API easier to navigate and maintain. - Naming Conventions: Use clear and descriptive names for your API route files. This helps in understanding the purpose of each endpoint at a glance.
2. Handle Errors Gracefully
- Consistent Error Responses: Implement a consistent structure for error responses. This should include a standardized error message and, where applicable, a status code that accurately reflects the nature of the error.
- Try/Catch Blocks: Use try/catch blocks to catch exceptions and respond with appropriate error messages and status codes.
- Validation Errors: Validate request data and provide detailed error messages for validation failures, helping clients correct their requests.
3. Secure Your API
- Authentication and Authorization: Implement authentication and authorization checks to protect sensitive endpoints. Consider using JWT (JSON Web Tokens) or session-based authentication mechanisms.
- CORS (Cross-Origin Resource Sharing): Configure CORS policies as needed to restrict which domains can access your API, preventing unauthorized access from other domains.
- Rate Limiting: Prevent abuse and ensure the availability of your API by implementing rate limiting. This limits the number of requests a user can make within a certain timeframe.
- Input Validation: Validate all incoming data to protect against SQL injection, cross-site scripting (XSS), and other common web vulnerabilities. Use libraries like Joi or Yup for schema validation.
4. Optimize for Performance
- Minimize External API Calls: Cache external API responses where possible and avoid unnecessary calls within your API routes to improve response times.
- Database Interactions: Optimize database queries to reduce response times. Use indexes appropriately and avoid N+1 query problems.
- Serverless Considerations: Since Next.js API routes can run as serverless functions, be mindful of cold starts and optimize your code for quick execution.
5. Use Environment Variables for Configuration
- Securely Store Secrets: Use environment variables to securely store API keys, database connection strings, and other sensitive information. Next.js supports
.env
files for environment-specific configurations.
6. Leverage Middleware for Common Tasks
- Reusable Logic: Use middleware for common tasks across multiple API routes, such as logging, authentication, and custom headers setting. Next.js 12 and later versions offer built-in support for middleware, enhancing API routes with additional capabilities.
7. Implement Efficient Data Handling
- Pagination: For endpoints that return large datasets, implement pagination to limit the data sent in a single response. This improves the performance of your API and the experience for end-users.
- Data Caching: Cache data that doesn't change often at the server level to reduce database load and improve response times for frequently accessed endpoints.
8. Document Your API
- Clear Documentation: Provide comprehensive documentation for your API, including endpoints, request parameters, response objects, and status codes. Tools like Swagger or Redoc can help automatically generate interactive API documentation.
Real-world Example: Building a CRUD API with Next.js
Let's create a simple CRUD (Create, Read, Update, Delete) API for managing articles in a Next.js application. We'll use a hypothetical database utility (db.js
) for data operations, illustrating how to perform server-side operations with Next.js API routes.
// pages/api/articles/[id].js
import { findArticle, updateArticle, deleteArticle } from '../../../db';
export default async function handler(req, res) {
const { id } = req.query;
switch (req.method) {
case 'GET':
const article = await findArticle(id);
res.status(200).json(article);
break;
case 'PUT':
const updatedArticle = await updateArticle(id, req.body);
res.status(200).json(updatedArticle);
break;
case 'DELETE':
await deleteArticle(id);
res.status(204).end();
break;
default:
res.setHeader('Allow', ['GET', 'PUT', 'DELETE']);
res.status(405).end(`Method ${req.method} Not Allowed`);
}
}
This example demonstrates handling different HTTP methods to perform CRUD operations on articles. It showcases the versatility and power of Next.js API routes for server-side processing.
Conclusion
Next.js API routes offer a seamless way to integrate server-side logic into your Next.js applications. By leveraging this feature, developers can create efficient, scalable, and secure server-side operations without the need for a separate backend service. As you explore the potential of Next.js API routes, remember to follow best practices for structuring, security, and performance to build robust and maintainable APIs.