How to Build a Basic API with Strapi: A Complete Guide to CRUD Operations
- User Experience
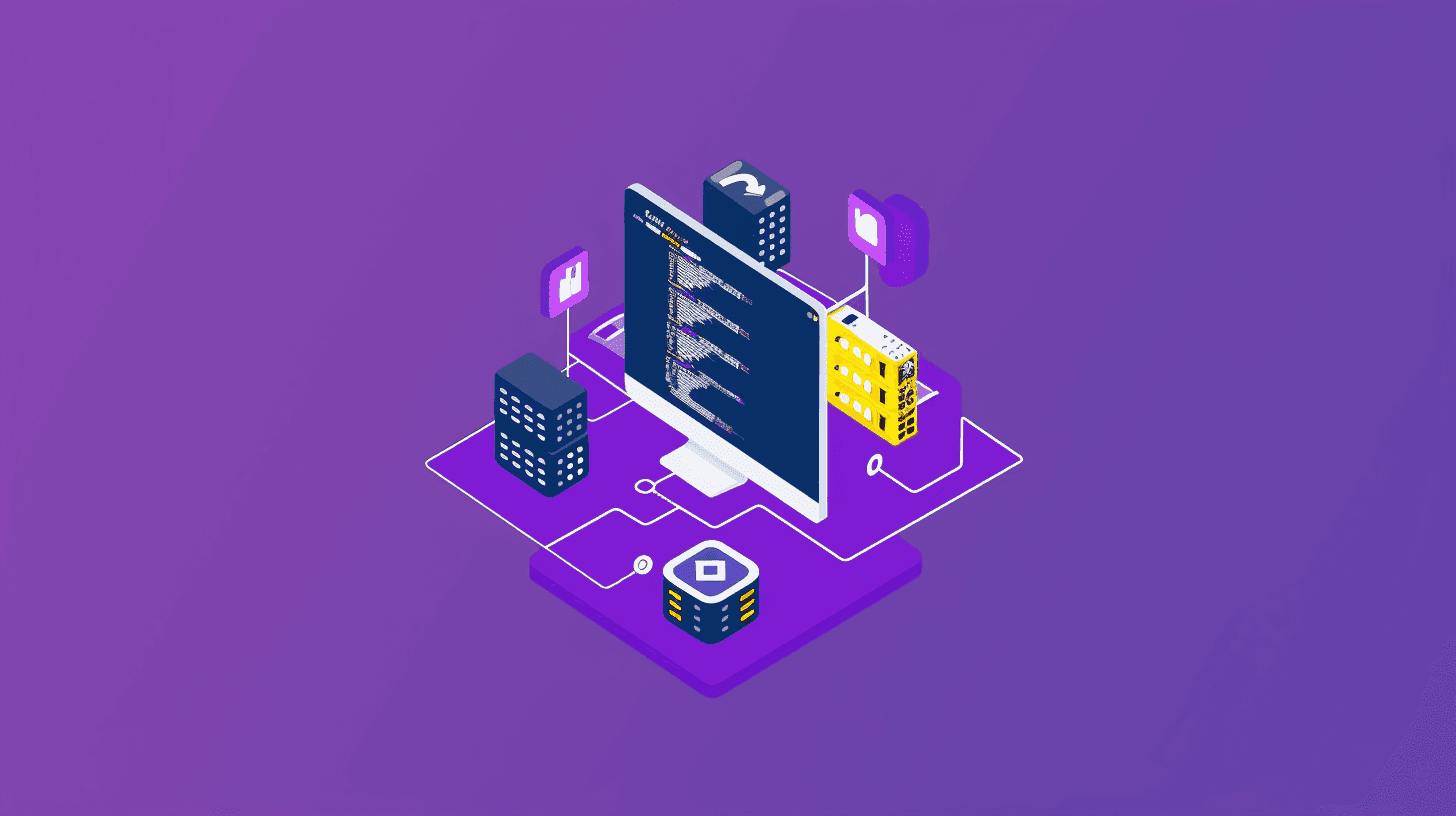
In today's fast-paced digital world, the ability to rapidly develop and manage web content is crucial.
Content Management Systems (CMS) have evolved to meet these needs, and with the rise of headless CMS options, developers have unprecedented flexibility in how they deliver content across platforms.
Strapi, a leading open-source headless CMS, allows developers to build customizable APIs easily.
This article from Kapsys will explore how to set up a basic API with Strapi and perform Create, Read, Update, and Delete (CRUD) operations using React JS.
Understanding CRUD Operations
What are CRUD Operations?
CRUD stands for Create, Read, Update, and Delete. These are the four basic operations that can be performed on data in a database. In the context of a CMS like Strapi:
- Create: Add new data.
- Read: Retrieve data.
- Update: Modify existing data.
- Delete: Remove data.
Understanding CRUD is essential for developing dynamic applications, allowing developers to design efficient data interaction methods.
Why Use Strapi for CRUD Operations?
Strapi provides a flexible, easy-to-use platform for managing and distributing content via APIs. Being a headless CMS, developers can use front-end tools (like React JS) to deliver content. This is especially useful for building CRUD apps where timely and specific data manipulation is crucial.
Setting Up Strapi
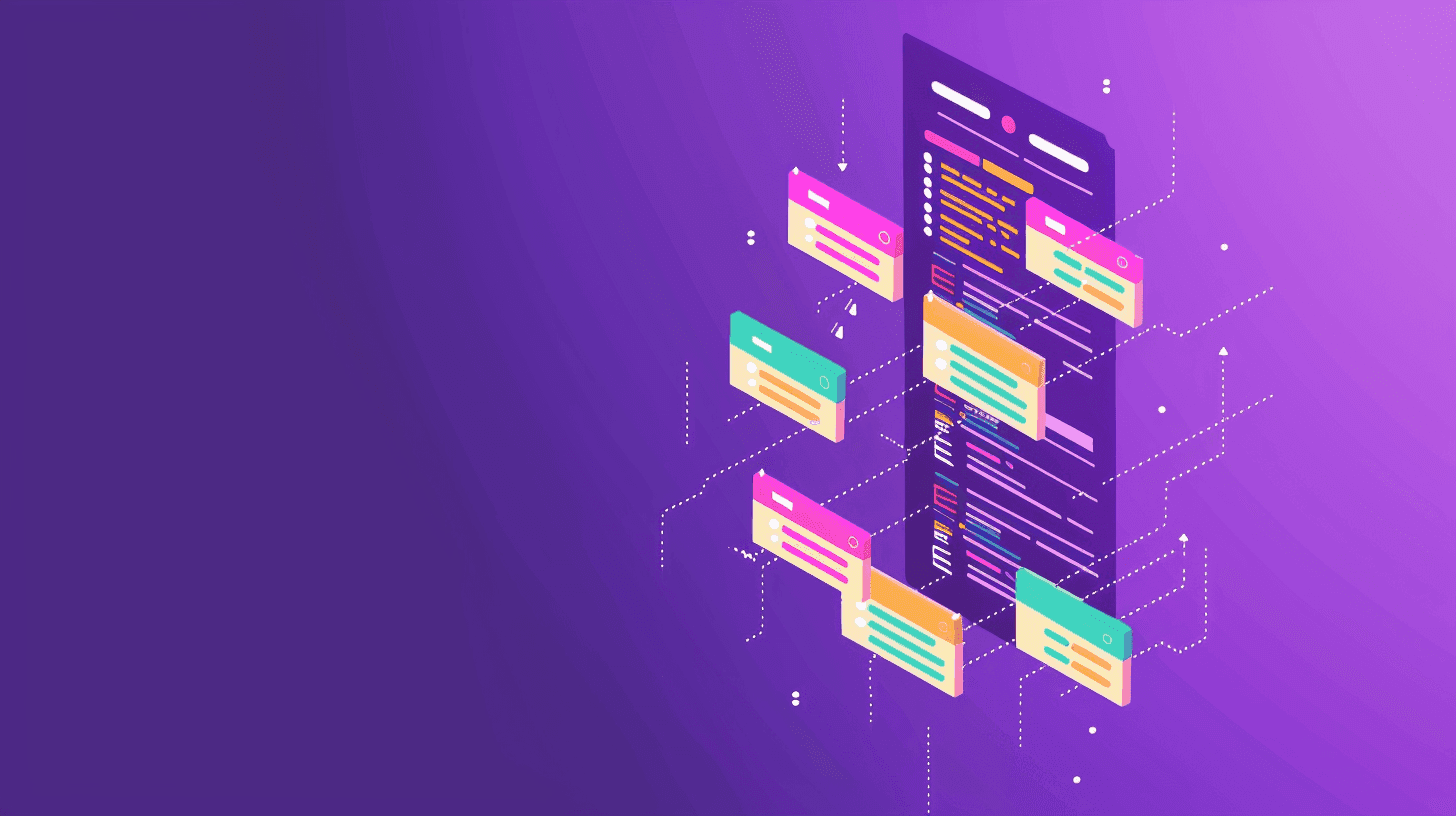
Installation
First, you need to install Strapi. You can do this by running the following command in your terminal:
npx create-strapi-app my-project --quickstart
This command creates a new Strapi project with a default SQLite database and starts the Strapi server.
Creating Your First Content Type
Once Strapi is up and running, navigate to the admin panel (usually at http://localhost:1337/admin
). Here, you can create your first content type:
- Click on "Content-Types Builder" in the sidebar.
- Click "Create new collection type".
- Name your content type (e.g.,
Article
) and add fields (e.g.,title
,body
,published_at
).
After configuring the fields, save and publish your content type.
Integrating Strapi with React JS
Setting Up React Application
To use Strapi with React JS, start by creating a new React application:
npx create-react-app my-react-app
cd my-react-app
Fetching Data from Strapi
To fetch data from your Strapi API, you can use the fetch
API or libraries like Axios. Here's an example using fetch
:
fetch('http://localhost:1337/articles')
.then(response => response.json())
.then(data => console.log(data));
This code retrieves all articles from the articles
content type in Strapi.
Performing CRUD Operations
Create (POST)
To add a new article through your React app:
const article = { title: 'New Article', body: 'Content of the new article.' };
fetch('http://localhost:1337/articles', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(article)
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Read (GET)
We already saw how to read data. To fetch a single article by ID:
fetch('http://localhost:1337/articles/1')
.then(response => response.json())
.then(article => console.log(article));
Update (PUT)
To update an existing article:
const articleUpdate = { title: 'Updated Title', body: 'Updated content of the article.' };
fetch('http://localhost:1337/articles/1', {
method: 'PUT',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(articleUpdate)
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Delete (DELETE)
To delete an article:
fetch('http://localhost:1337/articles/1', {
method: 'DELETE'
})
.then(() => console.log('Deleted'))
.catch(error => console.error('Error:', error));
Conclusion
Strapi offers a powerful platform for developers to build flexible CRUD applications using headless CMS architecture. By integrating Strapi with React JS, developers can efficiently manage and present dynamic content.
Whether you're building a blog, a complex web application, or anything else, understanding how to perform CRUD operations with Strapi and React will significantly enhance your project's capabilities.