How To Integrate Next.js with Headless CMS
- User Experience
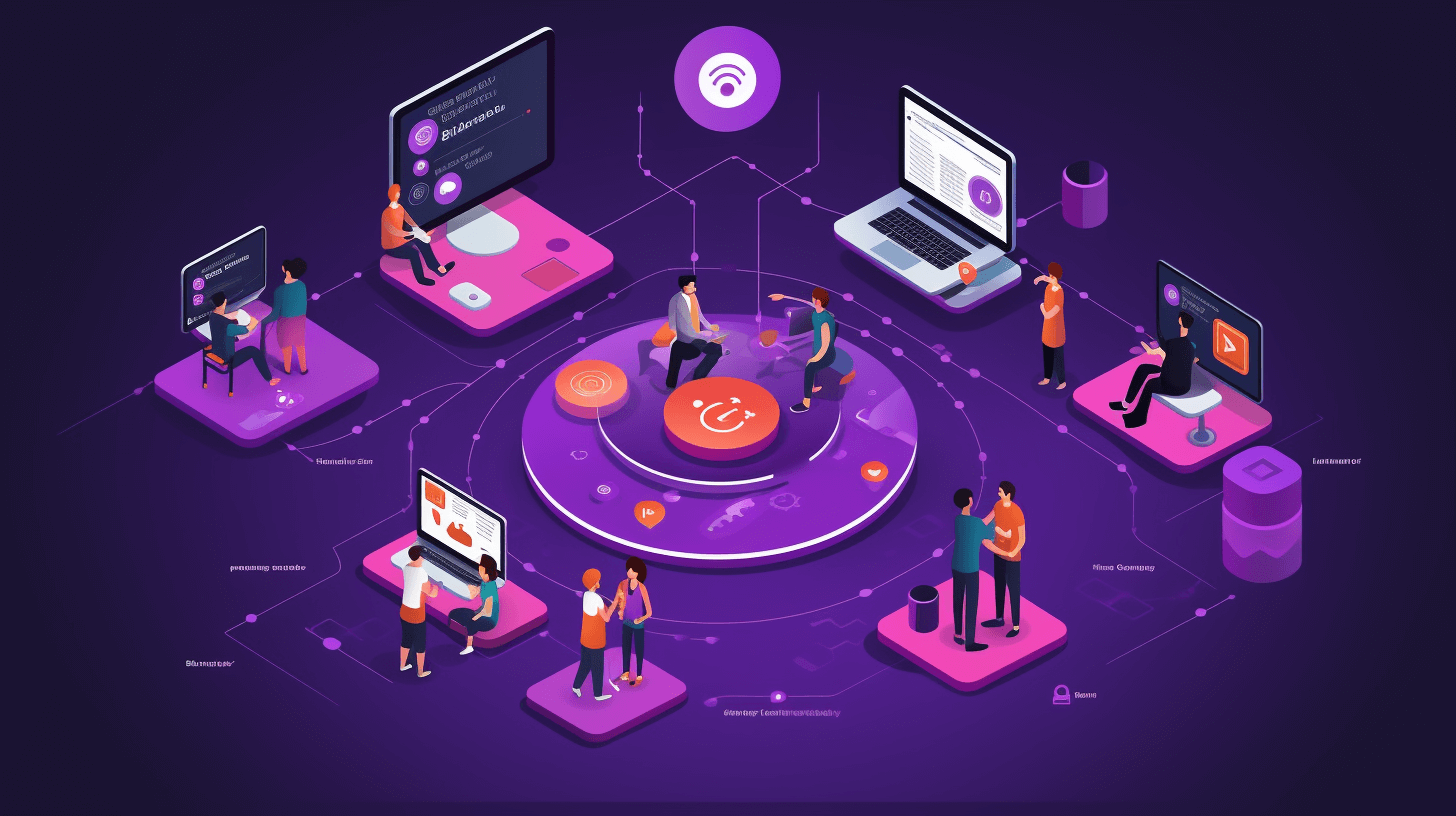
In the rapidly evolving world of web development, the combination of Next.js and headless Content Management Systems (CMS) is becoming increasingly popular. This powerful duo enables developers to build highly dynamic, scalable, and fast web applications by leveraging the best of both worlds: the server-side rendering and static site generation capabilities of Next.js with the flexibility and scalability of headless CMS. This article from Kapsys provides a comprehensive guide on how to integrate Next.js with a headless CMS, covering everything from the basics to more advanced configurations.
What is Next.js?
Next.js is an open-source React framework that enables developers to build server-side rendering and static web applications using React. It's designed to offer a better user experience and performance, with features that support SEO, faster page loads, and a more efficient development process. Here are some key features and benefits of Next.js:
Key Features of Next.js:
- Server-Side Rendering (SSR): Next.js allows React components to be rendered on the server side, which means the server sends the client a page with the initial state already populated. This improves performance and is beneficial for SEO as the content is already visible to search engines upon crawling.
- Static Site Generation (SSG): With Next.js, you can pre-render pages at build time. This feature, known as Static Site Generation, means that your web application can serve static pages with the speed and reliability of a CDN.
- Incremental Static Regeneration (ISR): Next.js offers the ability to update static content after deployment without rebuilding the entire site. This makes it easier to keep your site up-to-date while maintaining fast load times.
- File-based Routing: Next.js uses a file-based routing system, making routes based on the files in the
pages
directory. This simplifies the process of adding new routes and pages to your application. - API Routes: You can easily create API endpoints as part of your Next.js application. This allows for building APIs directly within your Next.js app, simplifying development by handling both the frontend and backend in one place.
- Built-in CSS and Sass Support: Next.js comes with built-in support for CSS and Sass, allowing for modular stylesheets with components. This enhances the styling process and makes it more efficient.
- Image Optimization: The
Image
component automatically optimizes images for performance, including resizing, optimizing, and serving images in modern formats like WebP when the browser supports it. - Fast Refresh: Next.js features a fast refresh capability that provides instant feedback on edits made to your React components. This makes the development process smoother and more enjoyable.
Why Choose Next.js with Headless CMS?
Choosing Next.js in combination with a headless Content Management System (CMS) for web development projects offers a range of benefits that cater to modern development needs, aiming for performance, scalability, and flexibility. This combination is particularly powerful for creating dynamic websites and applications that are content-rich yet maintain high performance and are SEO-friendly. Here are the key reasons to choose Next.js with a headless CMS:
Enhanced Performance and SEO
- Server-Side Rendering (SSR) and Static Generation (SSG): Next.js enables SSR and SSG, significantly improving web application performance. By pre-rendering pages on each request (SSR) or at build time (SSG), Next.js ensures that the content is immediately available to users and search engines, enhancing SEO and reducing load times.
- Optimized Content Delivery: Headless CMS platforms often come with CDN support, ensuring content is delivered quickly and efficiently worldwide. When paired with Next.js's image optimization features and fast content delivery capabilities, it results in lightning-fast web experiences.
Flexibility and Scalability
- Decoupled Architecture: A headless CMS provides a decoupled architecture, meaning the content management is separate from the presentation layer. This separation allows developers to use Next.js for the front end, leveraging its rich ecosystem and React's component-based architecture for a scalable and maintainable codebase.
- Cross-Platform Content Delivery: With a headless CMS, you can manage and deliver content to any platform with an internet connection, not just a Next.js application. This makes your content strategy more versatile and future-proof.
Improved Developer Experience
- Streamlined Development Process: Next.js offers a developer-friendly environment with features like hot reloading, automatic routing, and a plugin ecosystem, which, when combined with the API-first approach of a headless CMS, streamlines the development workflow.
- Easier Content Management: Non-technical users find it easier to manage content through a headless CMS's user interface, while developers can focus on the presentation layer using their preferred tools and frameworks like Next.js.
Better Content Management and Integration
- Content Flexibility: A headless CMS allows you to structure content as you see fit, making it easier to reuse across different parts of your application or even across different applications and platforms.
- Integration Capabilities: Most headless CMS options offer extensive APIs and webhooks, making integrating with other tools and services easier. This can extend the functionality of your Next.js application with third-party services, analytics, CRM systems, and more.
Future-Proofing Your Project
- Adaptability: As new technologies emerge, the decoupled nature of Next.js with a headless CMS means you can adapt your front-end or back-end technology stack without a complete overhaul.
- Incremental Adoption: Next.js and headless CMSs support incremental adoption, allowing you to migrate or upgrade your existing projects piece by piece rather than all at once.
Getting Started with Next.js and Headless CMS Integration
Integrating Next.js with a headless CMS combines the power of a modern frontend framework with the flexibility and ease of content management that a CMS provides. This setup is ideal for building dynamic websites that require frequent updates, such as blogs, e-commerce sites, and portfolios. Here’s how you can get started with integrating Next.js and a headless CMS.
Prerequisites:
- Node.js and npm installed on your system.
- Basic knowledge of React and how to use React components.
- An account with a headless CMS (e.g., Contentful, Sanity, Strapi, or Ghost).
Step 1: Set Up Your Next.js Project
First, create a new Next.js project if you haven't already. Open your terminal and run:
npx create-next-app@latest your-project-name
cd your-project-name
This command creates a new Next.js application and moves you into the project directory.
Step 2: Choose and Set Up Your Headless CMS
Select a headless CMS that suits your project needs. Each CMS has its own set of features and setup process. For the purpose of this guide, we'll assume you have already set up your CMS and have access to its API.
Step 3: Fetch Content from Your Headless CMS
To integrate your CMS with Next.js, you need to fetch content from the CMS's API and pass it to your Next.js pages or components.
- Create an API client: Depending on your CMS, you might need to install an SDK or use the native
fetch
API in JavaScript to make requests to your CMS's API. - Fetching data: Use Next.js's data fetching methods like
getStaticProps
for static generation orgetServerSideProps
for server-side rendering to fetch data from your CMS. For example:
export async function getStaticProps() {
// Replace 'YOUR_CMS_API_ENDPOINT' with your actual CMS API endpoint
// and adjust the fetching logic based on your CMS's API
const res = await fetch('YOUR_CMS_API_ENDPOINT');
const posts = await res.json();
return {
props: {
posts,
},
};
}
Step 4: Display Content in Your Next.js Application
Once you've fetched your content, you can use it in your React components to build your UI:
function HomePage({ posts }) {
return (
<div>
<h1>My Blog Posts</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</div>
);
}
export default HomePage;
Step 5: Dynamic Routing
Next.js supports dynamic routing, which allows you to create pages that can dynamically display content based on the URL. For content like blog posts, you can use the file name [slug].js
in the pages
directory and fetch the specific post from your CMS based on the slug.
// pages/posts/[slug].js
export async function getStaticPaths() {
// Fetch the list of posts slugs from your CMS
const paths = posts.map(post => ({
params: { slug: post.slug },
}));
return { paths, fallback: false };
}
export async function getStaticProps({ params }) {
// Fetch the post data based on the slug
const post = // fetch post data using params.slug
return { props: { post } };
}
Step 6: Optimize and Deploy
Utilize Next.js features like Image Optimization and Incremental Static Regeneration to enhance your site's performance. Finally, deploy your Next.js application on platforms like Vercel or Netlify for seamless integration with your headless CMS.
Conclusion
Integrating Next.js with a headless CMS offers a flexible, efficient, and scalable solution for building modern web applications. By following the steps outlined in this guide, developers can leverage both technologies' strengths to create dynamic, content-rich, and SEO-friendly websites. Whether you're building a blog, e-commerce site, or portfolio, Next.js and a headless CMS combine to provide a robust foundation for your web development projects.