How To Integrate State Management in Next.js Using Libraries like Redux
- User Experience
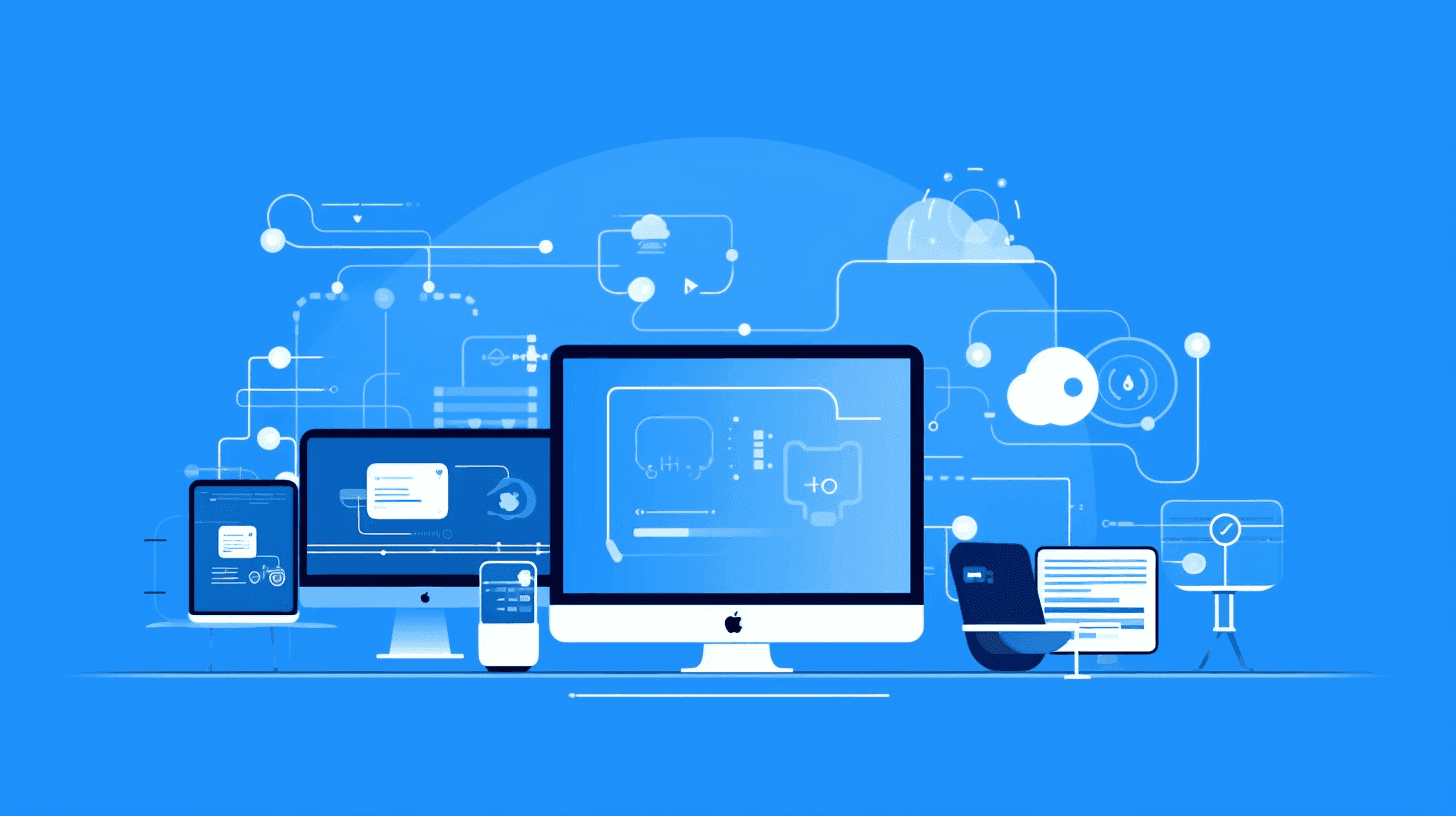
State management is an integral part of any web application. With Next.js gaining immense popularity, developers often wonder how to integrate state management systems, particularly Redux, into their projects. In this guide, Kapsys will highlight the steps to integrate redux with Next.js, ensuring efficient application state management.
What is Redux?
Redux is a predictable state container for JavaScript apps. It helps manage the state of your application in a centralized manner, making it easier to trace changes and maintain data consistency.
Why Use Redux with Next.js?
Next.js is a powerful React framework that offers SSR (Server-Side Rendering) capabilities. When merging redux next js projects, you benefit from:
- Consistency: A single source of truth for your application state.
- Debugging Capabilities: Easily trace back to the root cause of any changes.
- Efficient Data Flow: Unidirectional data flow ensures a more predictable application behavior.
Integrating Redux Next.js
When building applications with Next.js, managing state efficiently becomes paramount, especially as your project scales. Redux, a popular state management tool, ensures predictable state updates and offers an extensive ecosystem of middleware and developer tools. While straightforward, integrating Redux into a Next.js project requires a methodical approach. Below, we'll guide you through a step-by-step process to seamlessly integrate Redux with Next.js, harnessing the full power of both tools.
Step 1: Setting Up Your Next.js App
Before you integrate redux with next js, ensure you have a Next.js app set up. If you're starting from scratch, create a new app:
npx create-next-app your-app-name
Step 2: Installing Required Packages
Install the Redux and its required packages:
npm install redux react-redux next-redux-wrapper
This ensures you have the necessary tools to integrate Redux Next.js effectively.
Step 3: Setting up the Redux Store
Create a folder named store
in your project root. Inside this, add a reducer.js
file with your root reducer, and another file named store.js
:
store/reducer.js
const rootReducer = (state = {}, action) => {
switch (action.type) {
// Your reducers here
default:
return state;
}
};
export default rootReducer;
store/store.js
import { createStore } from 'redux';
import rootReducer from './reducer';
const store = createStore(rootReducer);
export default store;
Step 4: Integrating Redux with Next.js
To make Redux work seamlessly with server-side rendering offered by Next.js, use the next-redux-wrapper
:
store/store.js (Updated)
import { createStore } from 'redux';
import { createWrapper } from 'next-redux-wrapper';
import rootReducer from './reducer';
export const makeStore = (context) => createStore(rootReducer);
// Export the wrapper & createStore
export const wrapper = createWrapper(makeStore, { debug: true });
Step 5: Wrap Your Next.js App
Update your _app.js
to wrap your application using the Redux wrapper:
pages/_app.js
import { wrapper } from '../store/store';
function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />;
}
export default wrapper.withRedux(MyApp);
Using Redux in Next.js Components
With the setup complete, you can now harness the power of Redux in your components.
Connecting Components to the Redux Store
Using the connect
function, you can map state and dispatch to the props of your component:
import { connect } from 'react-redux';
function MyComponent({ data, dispatch }) {
// Your component logic here
}
const mapStateToProps = (state) => ({
data: state.data
});
export default connect(mapStateToProps)(MyComponent);
Conclusion
State management is a crucial aspect of web application development. Integrating Redux in Next.js projects ensures that you harness the best of both worlds: the server-side rendering of Next.js and Redux's centralized, predictable state management. By following the above steps, you'll have a robust setup to manage state seamlessly in your Next.js applications.
Remember, as with any tool, the effectiveness of redux with next js depends on how well you understand and utilize it. Always keep refining your skills and stay updated with changes to both Next.js and Redux for optimal development experience.