How To Use Dynamic Routing For Better Scalability In Next.js
- User Experience
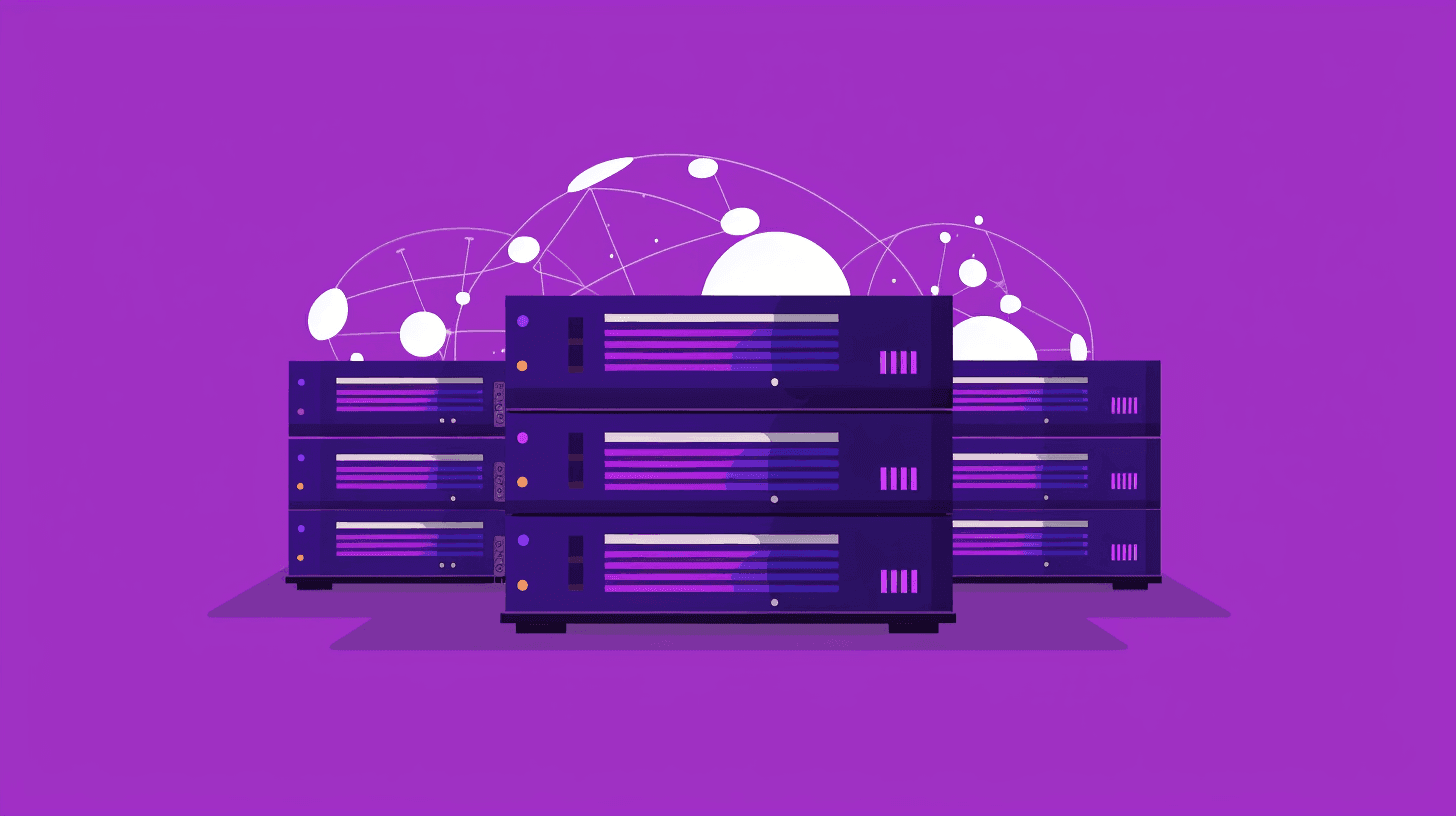
The journey to building scalable and efficient web applications often involves various challenges, including efficient routing. Routing is the mechanism that helps navigate between different parts of an application. Next.js, a popular React framework, offers a feature-rich routing system that includes static and dynamic routing. In this blog post, Kapsys will delve deep into Next.js dynamic routing. We’ll explore dynamic routing, how it can lead to better scalability, and the steps to implement it in your Next.js application.
What Is Dynamic Routing?
In contrast to static routing, dynamic routing allows us to generate routes dynamically based on the data received or the pattern matched. Instead of having a fixed route for every page, dynamic routes adjust based on variables, like user IDs or post titles, to serve different content. This enables your application to scale effectively without manually creating routes for every new page or entity.
What Is Dynamic Router?
The dynamic router is a component in Next.js that interprets the variable segments in the URL and renders the appropriate content based on those variables. This simplifies the routing mechanism, enabling Next.js to handle various simple and complex use-cases.
Why Dynamic Routing?
Here are some compelling reasons to consider using dynamic routing in Next.js:
Scalability
As your application grows, managing individual routes can become cumbersome. Dynamic routing offers a more scalable approach by reusing the same page layout and logic for multiple routes.
Flexibility
Dynamic routes are not tied to a specific URL structure. This means you can easily adapt your application's routes as requirements evolve without significantly changing the codebase.
Improved User Experience
With dynamic routing, you can create SEO-friendly URLs, personalize page content, and improve overall user experience without sacrificing performance.
How To Implement Dynamic Routing In Next.js
Dynamic routing in Next.js enables you to create routes dynamically based on the data or parameters you pass to the URL. This allows you to build highly interactive and data-driven websites. Here's a simple example to demonstrate how you can implement dynamic routing in a Next.js application.
File and Folder Naming Conventions
Next.js uses a file-system-based routing mechanism. To create a dynamic route, you simply name your file or folder using square brackets ([]
).
For example, to create a dynamic route for user profiles based on user IDs, you could create a file named userId.js
inside a folder named users
like so:
- pages
- users
- [userId].js
Query Parameters
The dynamic segment (userId
in the example) becomes a query parameter you can access in your component to render dynamic content.
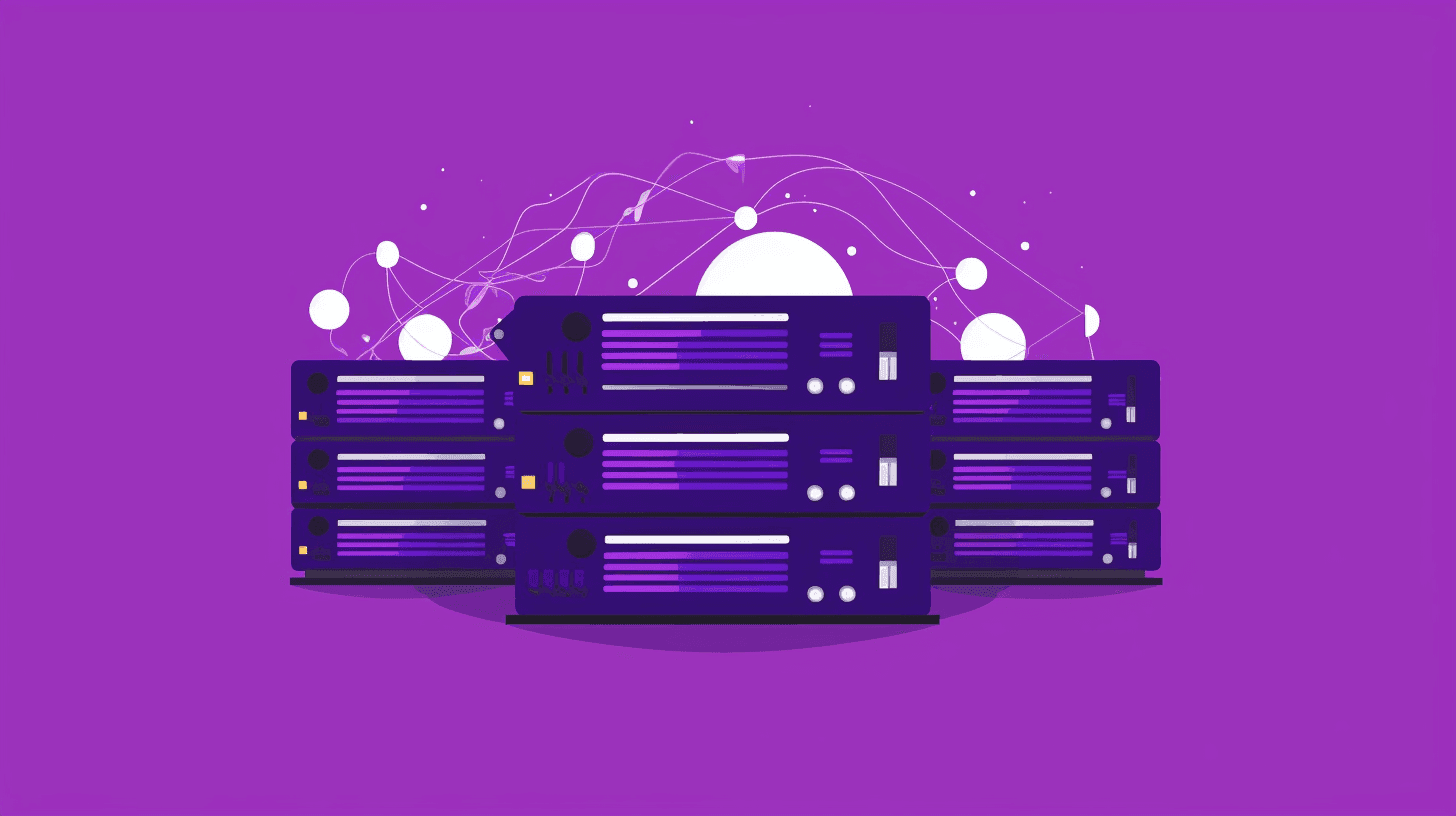
Fetching Data for Dynamic Routes
Once the file and folder structure is set up, you can fetch data based on the dynamic segment to render the appropriate content. You can achieve this using Next.js' getServerSideProps
, getStaticProps
, or getInitialProps
.
Using getServerSideProps
export async function getServerSideProps(context) {
const { userId } = context.query;
const userData = await fetch(`https://api.example.com/users/${userId}`);
const data = await userData.json();
return { props: { data } };
}
Using getStaticProps
and getStaticPaths
export async function getStaticPaths() {
return {
paths: [
{ params: { userId: '1' } },
{ params: { userId: '2' } }
],
fallback: true,
};
}
export async function getStaticProps({ params }) {
const userData = await fetch(`https://api.example.com/users/${params.userId}`);
const data = await userData.json();
return { props: { data } };
}
Accessing Dynamic Route Parameters
Within your Next.js page component, you can access the dynamic route parameter using useRouter
from next/router
:
import { useRouter } from 'next/router';
const UserProfile = ({ data }) => {
const router = useRouter();
const { userId } = router.query;
return (
<div>
<h1>User Profile for {userId}</h1>
<p>Name: {data.name}</p>
<p>Email: {data.email}</p>
</div>
);
};
Conclusion
Dynamic routing in Next.js offers an elegant, scalable solution for handling various routing scenarios in your web application. By understanding what dynamic routing is and how to implement it, you can create more flexible, maintainable, and user-friendly applications. This guide aimed to cover the essentials of Next.js dynamic routes, providing you with the knowledge to start implementing dynamic routing in your Next.js projects effectively.