Next.js PWA: The Ultimate Guide to Building Progressive Web Apps
- User Experience
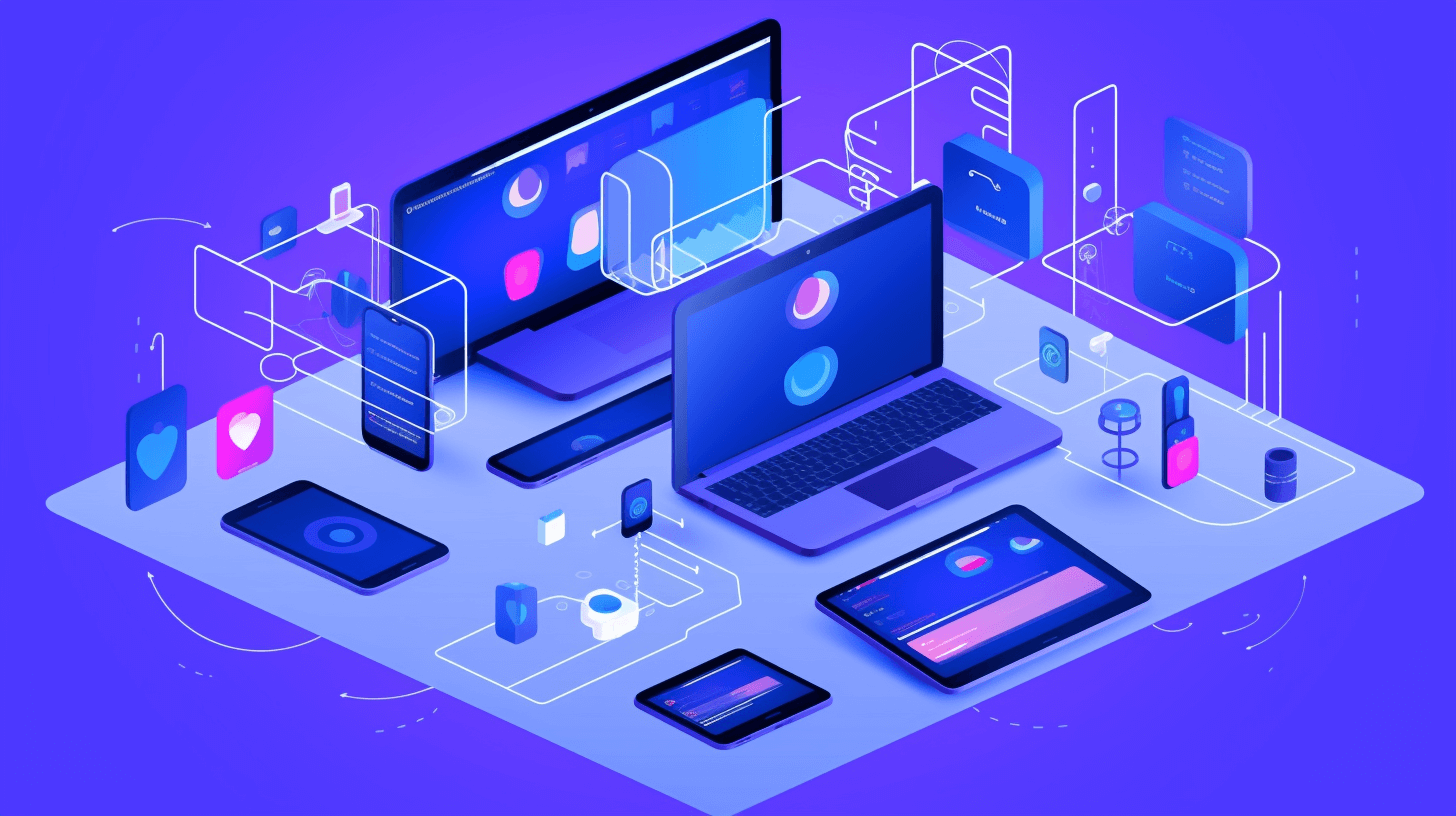
Progressive Web Apps (PWAs) are revolutionizing how we experience the web. Combining the best web and mobile app features, PWAs offer users a seamless, installable, and highly responsive experience. With the rise of frameworks like Next.js, creating PWAs has become more accessible and robust. In this ultimate guide, Kapsys walks you through building a Next.js PWA, ensuring you harness the full potential of this powerful combo.
Understanding the Basics of a Next.js PWA
Progressive Web Apps are not just buzzwords; they represent a fundamental shift in web development, providing functionality and seamless user experience akin to native apps. When these capabilities are combined with Next.js, a premier framework for React, you create a robust Next.js PWA that sets the standard for modern web applications. Here, we’ll uncover what a Next.js PWA entails and why it's the smart choice for developers looking to deliver exceptional web experiences.
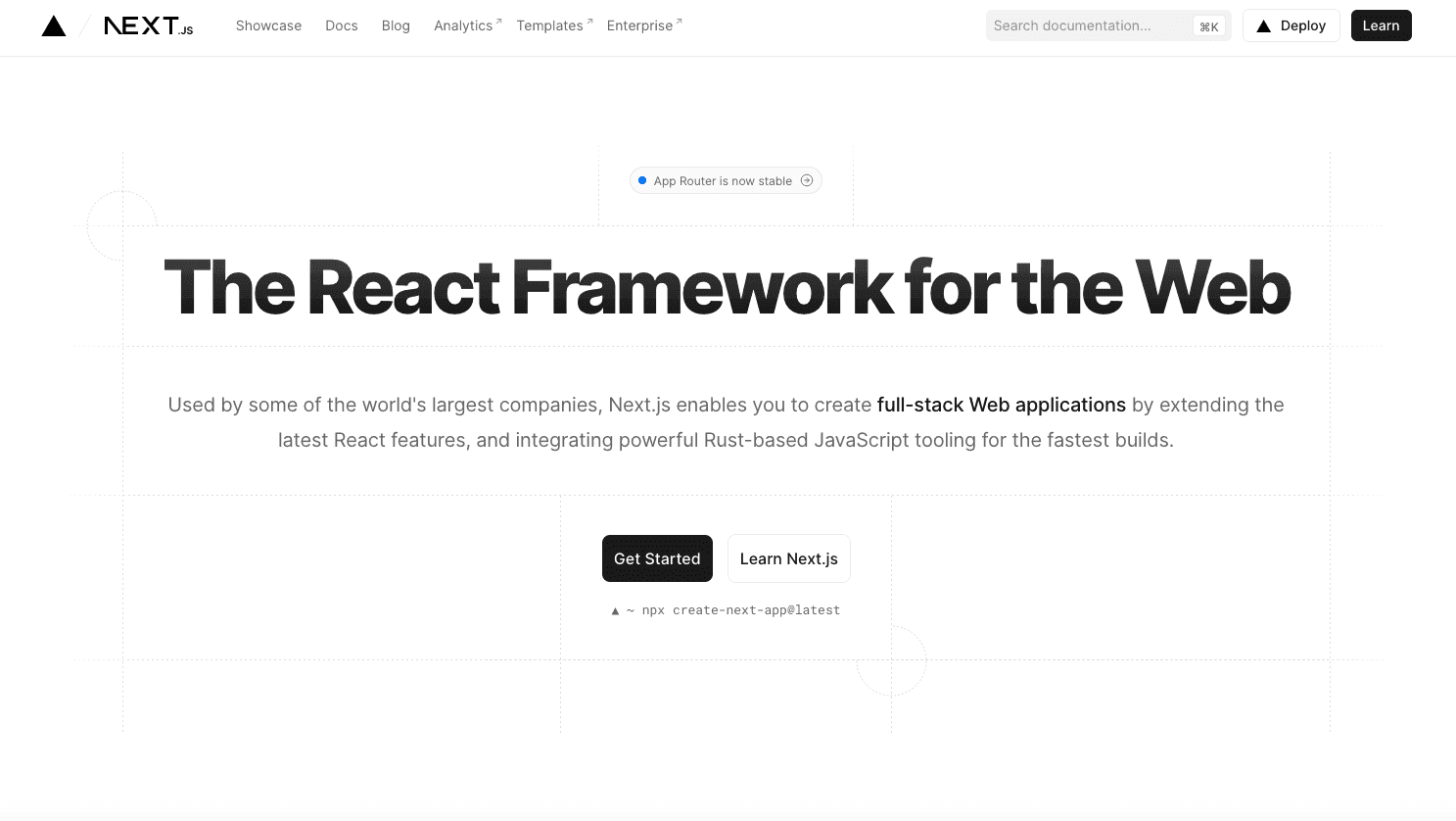
What is a Next.js PWA?
A Next.js PWA (Progressive Web App) is a web application built using the Next.js framework incorporating features typically associated with native applications. It combines the broad reach of the web with the rich user experience of a mobile app. PWAs are designed to work on any platform with a standards-compliant browser, including desktop and mobile devices.
Next.js is a React-based framework that offers server-side rendering, static site generation, and many other features that make web development more efficient. When you use Next.js to build a PWA, you’re leveraging its capabilities to create a web application that can:
- Load quickly and run smoothly, enhancing the user experience.
- Work offline or on low-quality networks, thanks to service workers.
- Act like a native app on mobile devices, with features like full-screen experiences and the ability to be added to the home screen.
- Send push notifications to users, engaging them even when they’re not actively using your app.
- Be discoverable by search engines, which can help with SEO and ensure a wider reach.
In essence, a Next.js PWA is a fast, reliable, and engaging web app that offers a user experience akin to a native app while still being discoverable and linkable like a web app. This makes Next.js PWAs a compelling choice for developers looking to build web applications that stand out in performance and user engagement.
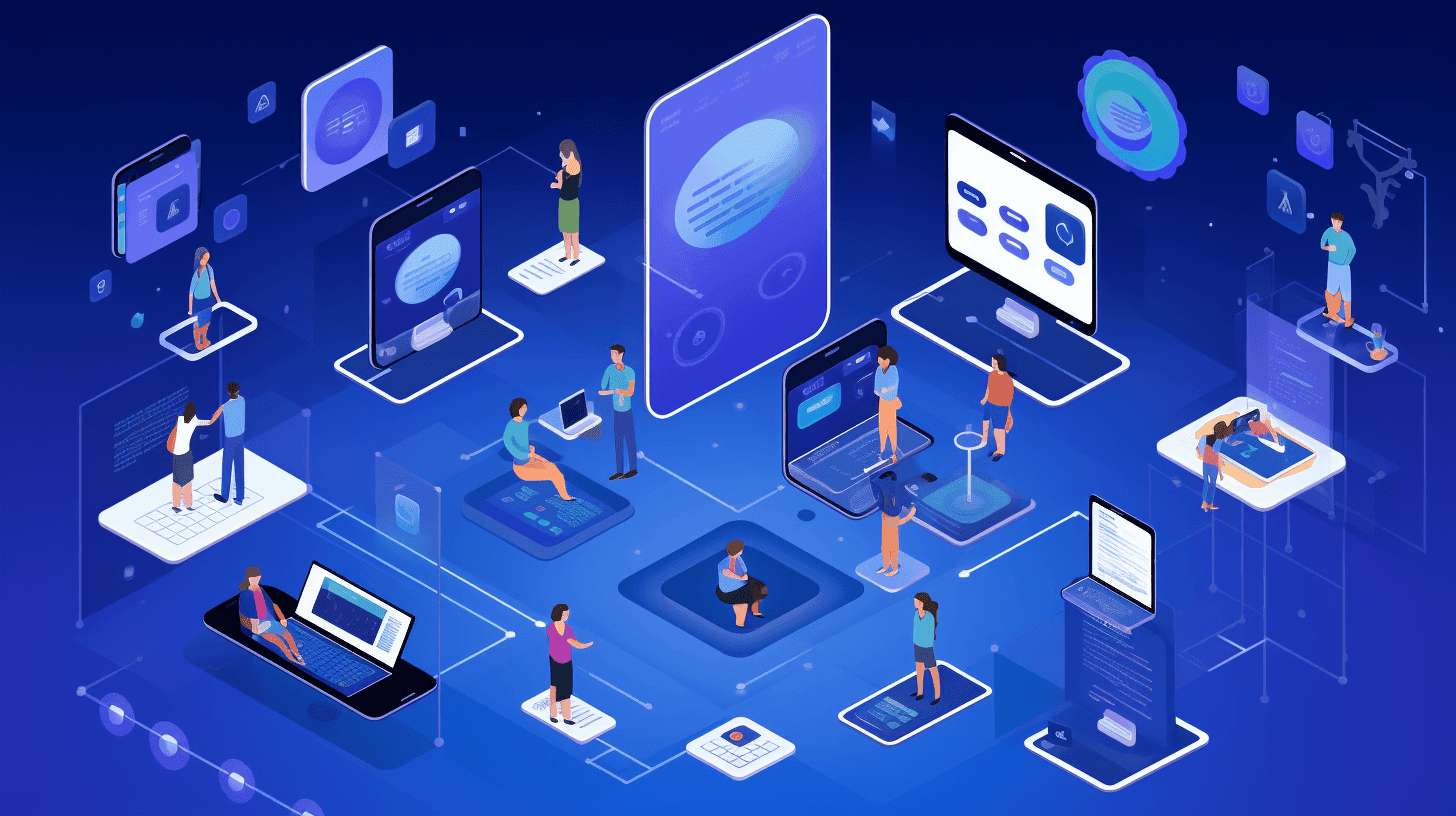
Key Features of a Next.js PWA
A Next.js Progressive Web App (PWA) encompasses features that elevate it from a standard web application to a more performant and user-centric experience. Here are the key features that typically characterize a Next.js PWA:
Service Workers
Service workers act as a proxy between the web application and the network. They enable your Next.js PWA to handle offline requests, cache content, and perform background tasks even when the web page is not open. This feature enhances performance, especially in network-constrained environments, enabling push notifications and background data synchronization.
Web App Manifest
This is a simple JSON file that controls how your Next.js PWA appears to the user in areas where they would expect to see apps (for example, the home screen of a device). It allows you to define the name of your app, the starting URL, icons, and even the screen orientation. The manifest ensures that when your PWA is installed on a device, it offers a full-screen, app-like experience without the browser Chrome.
Responsive and Adaptive UI
Next.js is built on top of React, which is conducive to creating responsive and adaptive user interfaces. This ensures that your PWA looks great and functions well on any device, from desktops to tablets and smartphones, maintaining a consistent user experience across different screen sizes and resolutions.
Reliable Performance
Next.js strongly focuses on performance, with features like automatic code-splitting, which loads only the necessary code for the page being viewed, and hybrid static & server rendering, which ensures that pages load quickly. This reliability, even in uncertain network conditions, is a defining trait of a PWA.
Push Notifications
PWAs built with Next.js can use web push notifications to engage users with timely, personalized content. This feature helps to re-engage users with customized content, fostering a deeper user connection to the app.
Security
Next.js enforces best practices for security, such as automatic output escaping to prevent cross-site scripting (XSS) attacks. Additionally, PWAs require HTTPS to ensure secure connections, which Next.js supports out of the box, especially when deployed on platforms like Vercel or Netlify.
Accessibility
Next.js encourages building accessible web applications, and this extends to PWAs as well. Accessibility is a cornerstone, ensuring that the app can be used by as many people as possible, including those with disabilities.
SEO-Friendly
Thanks to server-side rendering and static generation features, Next.js PWAs are SEO-friendly out of the box. This means that the content of the PWA can be indexed by search engines, which is not always the case with single-page applications (SPAs).
Easy Deployment and Scalability
With Next.js, deploying a PWA is straightforward, especially when using Vercel, the company behind Next.js. The framework is designed to scale seamlessly, managing both static and dynamic content efficiently.
Setting Up Your Next.js PWA Environment
Setting up the environment for a Next.js PWA is the first crucial step in the development process. This setup includes installing the necessary tools, configuring the development environment, and initializing a new Next.js project. Here’s a step-by-step guide to getting started:
Prerequisites
Before you begin, ensure that you have the following prerequisites installed on your system:
- Node.js: Next.js requires Node.js, which is a JavaScript runtime that allows you to run JavaScript on the server.
- npm or Yarn: These are package managers that you will use to install and manage dependencies in your Next.js project.
You can download Node.js, which includes npm, from the official Node.js website.
Step 1: Creating a New Next.js Project
To create a new Next.js project, open your terminal and run the following command:
npx create-next-app@latest my-next-pwa
This command creates a new folder named my-next-pwa
with a starter Next.js project. If you prefer using Yarn, you can replace npx
with yarn create
.
Step 2: Installing PWA Dependencies
To turn your Next.js application into a PWA, you must add specific dependencies that enable service workers and manifest functionality. In your project directory, install the following packages:
npm install next-pwa
The next-pwa
package is a zero-config PWA plugin for Next.js that simplifies the integration of PWA features.
Step 3: Configuring next.config.js for PWA
In the root of your Next.js project, create or modify the next.config.js
file to include the next-pwa
plugin:
const withPWA = require('next-pwa');
module.exports = withPWA({
pwa: {
dest: 'public',
register: true,
skipWaiting: true,
},
// your other Next.js config here
});
This configuration tells Next.js to generate service workers for your application and defines the basic settings for the PWA.
Step 4: Setting Up the Manifest and Service Worker
Next, you'll need to set up your web app manifest and customize the service worker. Create a manifest.json
file in the public
directory with the following content:
{
"name": "My Next.js PWA",
"short_name": "NextPWA",
"start_url": "/",
"display": "standalone",
"background_color": "#ffffff",
"description": "An example of a Next.js PWA",
"icons": [
// add icons in various sizes
]
}
Add icons to the manifest to represent your app on devices, ensuring you include various sizes for different devices.
Step 5: Adding Service Worker Support
In your pages/_app.js
file, add the following code to register the service worker using the next-pwa
plugin:
import { useEffect } from 'react';
const MyApp = ({ Component, pageProps }) => {
useEffect(() => {
if ('serviceWorker' in navigator) {
navigator.serviceWorker
.register('/sw.js')
.then(() => {
console.log('Service worker registered!');
})
.catch((err) => {
console.log('Service worker registration failed', err);
});
}
}, []);
return <Component {...pageProps} />;
};
export default MyApp;
With these steps completed, you have successfully set up the environment for a Next.js PWA. The next phase is to develop your application and integrate additional PWA features, like offline support and push notifications, to enhance the user experience.
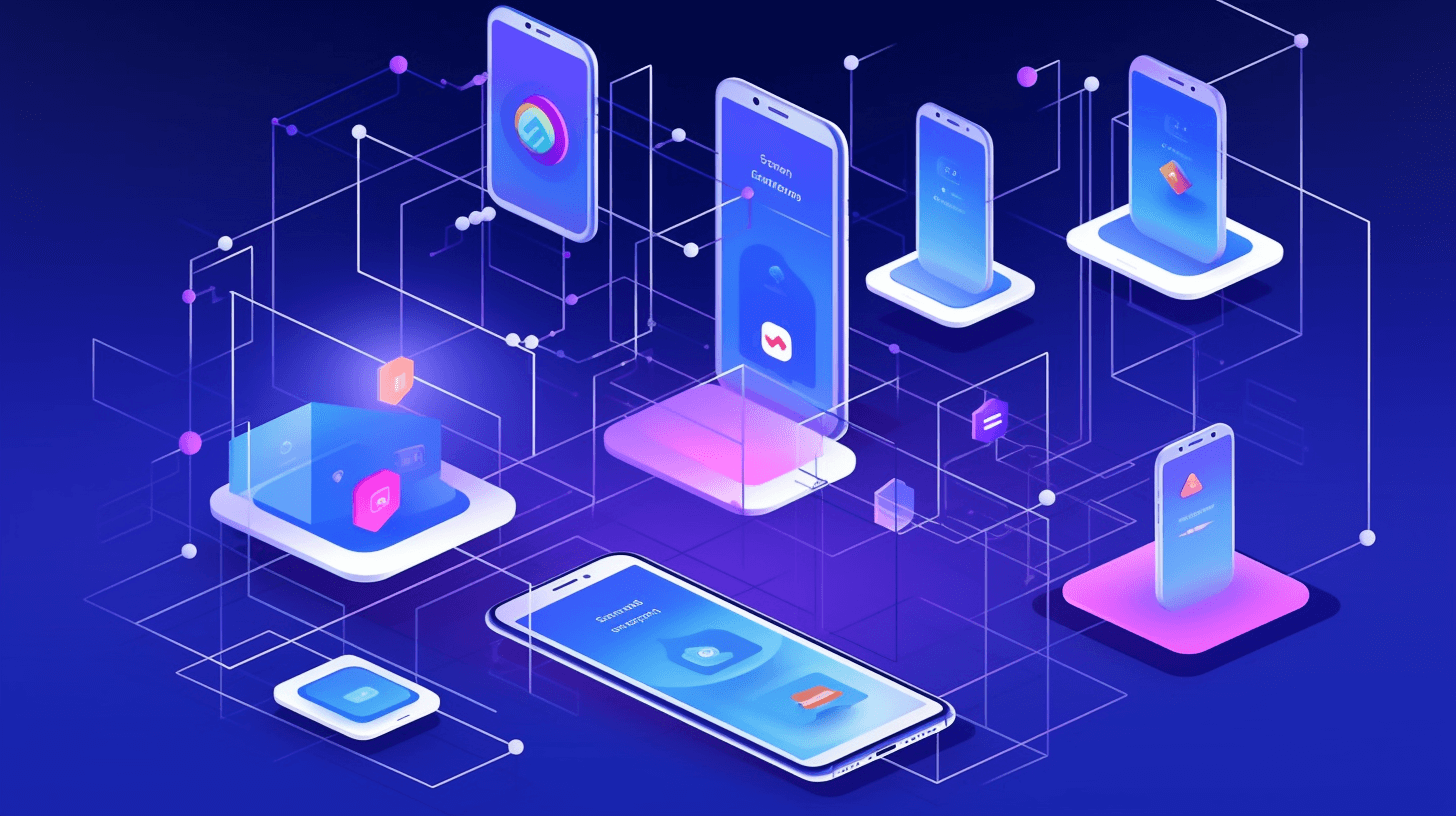
Architecting Your Next.js PWA
Architecting your Next.js PWA is about creating a solid foundation that not only harnesses the power of Next.js and its server-side rendering capabilities but also integrates the progressive web app qualities effectively. A well-architected Next.js PWA is robust, scalable, and maintains high performance while providing an app-like experience.
Designing a Scalable Directory Structure
When planning the directory structure of your Next.js PWA, it’s important to consider the scale of your application and how it might grow over time. A modular directory structure, where components, pages, and modules are organized logically, will make it easier to maintain and update your app.
- Pages: In Next.js, each page is associated with a route based on its file name. Keeping your pages directory clean and only for page components helps manage the routes effectively.
- Components: Reusable UI components should be placed in a separate
components
directory. You might want to further organize this directory by UI functionality or page-specific components. - Public: Static assets such as images, manifest, and icons are placed in the
public
directory. This directory is served at the root level of your app. - Styles: Even if you use a CSS-in-JS library, you may have global styles or themes. Keep these in a specific
styles
orthemes
directory. - Libraries/Helpers: Utility functions and custom hooks can be stored in a
lib
orutils
directory for better accessibility throughout your application. - Store: Using state management libraries like Redux or Zustand, keep your store, actions, reducers, or atoms within a dedicated store directory.
Implementing Efficient Data Fetching
Next.js offers several data fetching methods like getStaticProps
, getServerSideProps
, and getInitialProps
. Choosing the right one is essential for performance:
- getStaticProps: Use this for generating static pages at build time. It’s great for pages that fetch data from headless CMS or static files.
- getServerSideProps: This is used for server-side rendering on a per-request basis. It suits scenarios where data changes frequently, and you must present the most up-to-date content.
- getStaticPaths: In combination with
getStaticProps
, this method allows for dynamic routing and is ideal for static generation of pages with dynamic routes, like blog posts or product pages.
Optimizing for Performance
Performance is a hallmark of PWAs. Next.js has built-in performance optimizations, but you should still:
- Code Splitting: Next.js automatically splits your code at the page level, but you can also use dynamic imports to further split your code and load it only when needed.
- Caching: Leverage browser caching for static assets and implement a caching strategy with service workers for dynamic content.
- Image Optimization: Use the built-in Image component from Next.js for optimized image delivery.
Ensuring Offline Capability
Service workers are the backbone of offline functionality in PWAs. You can implement a caching strategy to cache the shell of your application and the data required to display pages:
- Caching Strategies: Choose the right caching strategy for your needs, whether it’s cache-first, network-first, or stale-while-revalidate.
- Cache Management: Implement cache versioning to remove outdated caches and ensure users receive the most up-to-date content online.
Integrating PWA Features
Next.js PWAs should feel like native apps, which means integrating features such as:
- Web App Manifest: This includes configuring the look and feel of your PWA when installed on a device.
- Push Notifications: Use service workers to manage push notifications, keeping users engaged even when they’re not on your site.
Accessibility and SEO
Lastly, ensure your Next.js PWA is accessible to all users and easily discoverable:
- Accessibility: Follow the Web Content Accessibility Guidelines (WCAG) to ensure your app is usable by as many people as possible.
- SEO: Utilize Next.js’s SSR and pre-rendering features to ensure search engines can crawl and index your site effectively.
Enhancing Your Application for PWA
Enhancing a Next.js application to function effectively as a Progressive Web App (PWA) involves implementing various best practices and technologies to create a user experience that rivals native apps. Here's how to elevate your Next.js application to PWA status:
Improve Loading Performance
- Optimize Assets: Compress images and use modern formats like WebP. Utilize the
next/image
component for automatic image optimization. - Minimize Bundle Size: Analyze your bundle size with tools like
webpack-bundle-analyzer
and remove unnecessary libraries or functions. - Server-Side Rendering (SSR): Use SSR for initial page loads to serve content to users and search engines faster.
- Static Generation: Pre-render pages at build time using
getStaticProps
orgetStaticPaths
when the data doesn't change often.
Implement an Offline Experience
- Service Workers: Create a service worker script to cache assets and API responses, allowing your application to work without a network connection.
- Cache Strategy: Define a caching strategy (e.g., cache-first, network-first) for different types of resources.
- Background Sync: Use the Background Sync API to queue actions performed offline and execute them when the connection is restored.
Create an App-Like Experience
- Web Manifest: Add a
manifest.json
file to define the installable aspects of your PWA, such as the home screen icons, splash screen, and theme colors. - Home Screen Installation: Prompt users to add your PWA to their home screen, providing full-screen and standalone navigation.
- Smooth Animations and Transitions: Implement performant animations and transitions to enhance the feeling of a native app.
Enhance Interactivity and Engagement
- Push Notifications: Integrate push notifications to re-engage users with your app by sending timely updates or reminders.
- Background Fetch: Use the Background Fetch API to periodically fetch data in the background, keeping the app content up-to-date.
Maintain Security and Privacy
- HTTPS: Ensure your application is served over HTTPS to secure data transfer between the client and server.
- Data Protection: Implement proper security measures to protect user data, including using web security headers and sanitizing user input.
Optimize for SEO
- Meta Tags: Use descriptive and keyword-rich meta tags for better visibility in search engine results.
- Structured Data: Implement structured data using JSON-LD to help search engines understand the content of your pages.
Monitor and Analyze Performance
- Lighthouse: Use Google Lighthouse to audit your PWA and identify areas for improvement.
- Real User Monitoring (RUM): Collect performance data from real users to understand the actual user experience.
Accessibility Considerations
- Semantic HTML: Use semantic HTML elements for better accessibility and SEO.
- ARIA Attributes: Include ARIA attributes where necessary to enhance the accessibility of dynamic content and complex UI components.
- Keyboard Navigation: Ensure that all interactive elements are accessible via keyboard navigation.
Prepare for Scalability
- State Management: Implement efficient state management using tools like React Context or state management libraries like Redux or Zustand.
- Error Handling: Develop a robust error handling system to gracefully manage and log errors.
Continuous Improvement
- Feedback Loop: Establish a feedback loop with your users to continually improve your app based on real-world usage.
- Performance Budget: Set a performance budget and track it throughout the development process to prevent regressions.
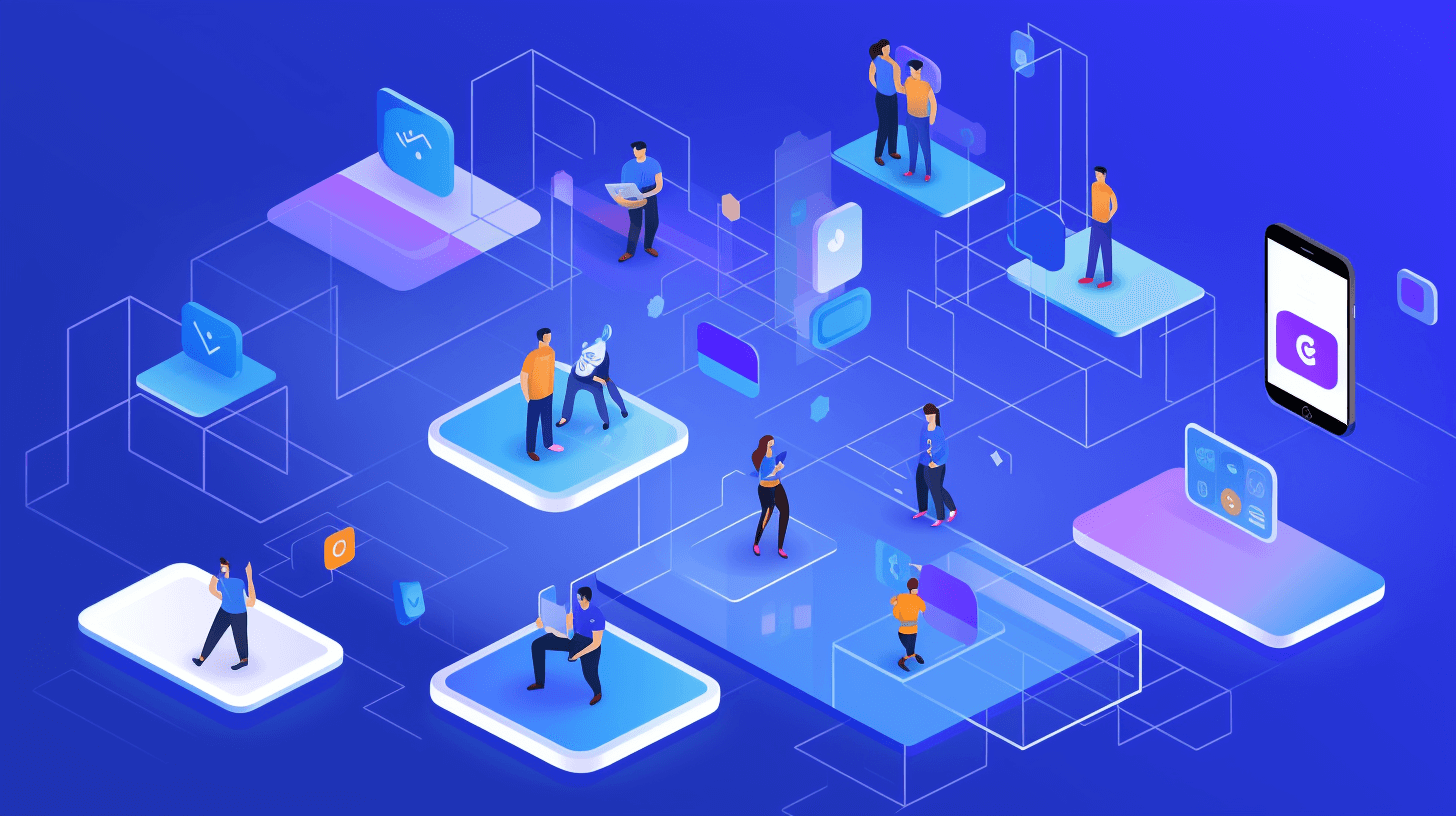
Optimizing Your Next.js PWA
Optimizing your Next.js Progressive Web App (PWA) is essential to ensure that it is not only functional but also efficient, fast, and user-friendly. Here are several strategies for optimizing your Next.js PWA:
Optimize Load Times
1. Server-Side Rendering (SSR) & Static Generation: Next.js excels in offering SSR and static generation methods like getStaticProps
and getServerSideProps
. Use these to serve pages quickly and improve the Time to First Byte (TTFB).
2. Code-Splitting: Next.js automatically code-splits on a per-page basis, but for large components on those pages, consider dynamic imports with import()
to split your code further.
3. Optimize Images: Utilize the next/image
component, which automatically optimizes images for the viewport and device, reducing load times for image-heavy pages.
Service Worker Strategies
1. Caching Assets: Use service workers to cache important assets and API calls, ensuring your app can still function during network interruptions.
2. Update Handling: Implement a strategy for handling updates to your service worker and cached assets to make sure users are always seeing the most current version without sacrificing the cache-first benefits.
Performance Monitoring
1. Web Vitals: Leverage Next.js’s built-in support for measuring Web Vitals, which provides quality signals important to user experience, such as LCP, FID, and CLS.
2. Website Audits: Regularly perform audits with tools like Google Lighthouse to find opportunities for optimization.
SEO and Accessibility
1. Meta Tags and SEO-Friendly Content: Ensure that each page has relevant meta tags, proper use of headings, and SEO-friendly URLs.
2. Accessible Design: Follow Web Content Accessibility Guidelines (WCAG) to ensure your PWA is usable by everyone, including people with disabilities.
Build Optimization
1. Analyze Bundle Size: Use tools next/bundle-analyzer
to visualize the size of webpack output files and optimize them.
2. Tree Shaking: Remove unused code from your bundles to reduce their size. Next.js and webpack support tree-shaking out-of-the-box.
User Experience
1. Progressive Enhancement: Build your PWA with progressive enhancement in mind, starting with a solid, functional core that works on all browsers and then adding enhancements that operate on more modern browsers.
2. Responsive Design: Ensure your PWA looks and functions well across all devices by using responsive design techniques.
State Management and Data Fetching
1. Efficient State Management: Consider using the Context API for smaller apps and more robust solutions like Redux or MobX for larger, more complex applications.
2. Smart Data Fetching: Fetch data on the server to reduce client-side load and improve user experience by displaying content faster.
Security
1. Content Security Policy (CSP): Implement a CSP to protect against scripting attacks and other cross-site injections.
2. HTTPS: Always use HTTPS to secure your application and protect users' data.
Push Notifications
1. User Engagement: Use push notifications sparingly and smartly to engage users without annoying them.
2. Subscription Management: Implement a system to manage push notification subscriptions and respect user preferences.
Continuous Integration/Continuous Deployment (CI/CD)
1. Automated Testing: Incorporate automated tests into your deployment process to catch bugs early.
2. Deployment Optimization: Use platforms like Vercel or Netlify for deploying Next.js apps, which provide global CDN, serverless functions, and optimization out-of-the-box.
Testing and Debugging Your Next.js PWA
Before your PWA goes live, rigorous testing and debugging are imperative. This section provides insights into effective testing strategies for a Next.js PWA, covering unit tests, end-to-end tests, and the tools that can aid in a thorough debugging process to ensure your app is robust and error-free.
By following this guide, you'll have a clear blueprint for building a Next.js PWA that stands out in the modern web landscape. The synergy of Next.js with PWA capabilities promises a cutting-edge app experience that is fast and engaging. Whether you're a seasoned developer or new to PWAs, this comprehensive guide is your road map to mastering Next.js PWA development.