Strapi v4: A Comprehensive Guide To Extending And Building Custom Resolvers
- User Experience
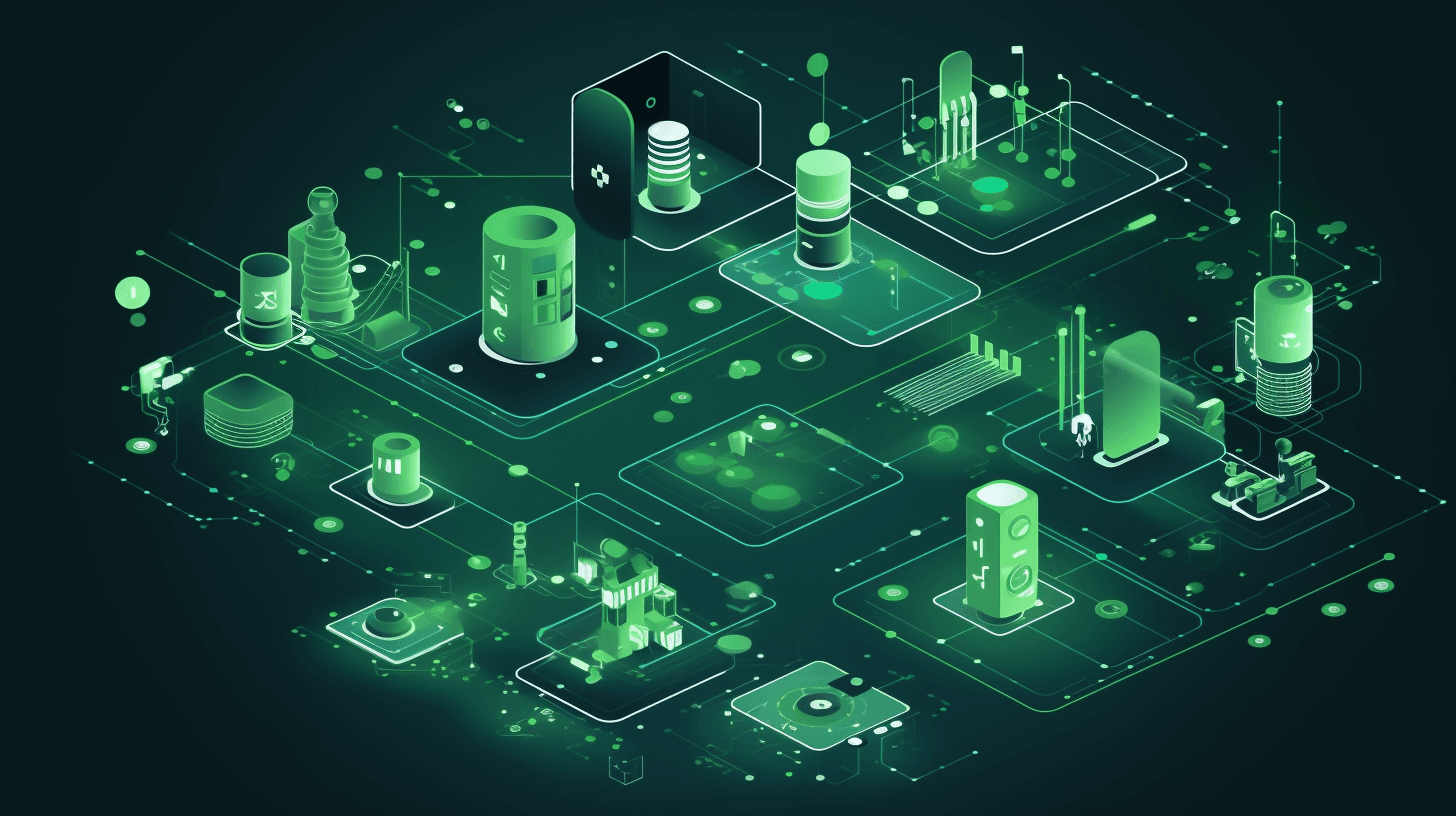
A robust content management system (CMS) is crucial for delivering dynamic and engaging digital experiences. Strapi, a headless CMS, has become a go-to choice for developers seeking flexibility and scalability. With the release of Strapi v4, a wealth of features has been introduced, including the ability to extend and build custom resolvers.
Join Kapsys in this comprehensive guide as we explore the heart of Strapi v4, exploring the intricacies of resolvers, defining resolver functions, and leveraging GraphQL typedefs to create a customized and powerful data-handling layer for your application.
What is a Headless CMS?
A headless CMS like Strapi v4 is a content management system that provides a back-end infrastructure for managing and delivering content. Still, it doesn't dictate how the content is presented on the front end:
A headless CMS decouples these components, unlike traditional CMS architectures, where the back and front end are tightly integrated.
In this model, the back end stores and manages content, while the front end fetches and displays it through an API (Application Programming Interface).
Advantages of headless CMS
The essential advantage of a headless CMS lies in its flexibility. Developers are not constrained by a specific technology stack for the front end; they can use their preferred frameworks and languages.
This flexibility benefits projects with diverse front-end requirements, such as websites, mobile apps, IoT devices, or applications needing content on various platforms.
What is Strapi v4?
Strapi v4 is the latest iteration of Strapi, an open-source headless CMS known for its flexibility, user-friendliness, and extensibility.
This version builds upon the strengths of its predecessors, introducing new features and improvements to enhance the development experience for users.
Critical features of Strapi v4
Let's take a look at what makes Strapi v4 special:
Custom Resolvers: Strapi v4 empowers developers to extend and build custom resolvers, providing precise control over the data-handling logic.
GraphQL: The introduction of GraphQL typedefs allows developers to define the structure and types of their GraphQL schema, offering a clear blueprint for data representation.
Extensibility: Highly extensible, Strapi v4 enables developers to customize and extend its functionality to meet the specific requirements of their projects.
Middleware Support: With middleware functions, developers can implement actions before or after the execution of a resolver, enhancing flexibility and control over the application's logic.
Authentication Mechanisms: Strapi v4 simplifies the implementation of authentication checks within resolvers, ensuring secure access to sensitive data and operations.
API-First Approach: Strapi v4 follows an API-first approach, allowing developers to focus on defining the data structure and API endpoints before building the front end.
Strapi v4 is designed to provide developers with a powerful and customizable headless CMS solution. Its rich feature set makes it suitable for various projects, from small websites to complex enterprise applications.
Read: What Is Strapi, And Why Is It A Leading Headless CMS?
Understanding Resolvers
A resolver, in essence, is a set of functions that define how data should be fetched, transformed, or manipulated in response to GraphQL queries or mutations. Each resolver corresponds to a specific field in your GraphQL schema, and its primary responsibility is to resolve the value for that field.
In Strapi v4, resolver functions are defined within the api directory of your project, making it easy to organize and manage your application's logic. A typical resolver might look like this:
// api/resolvers/customResolver.js
module.exports = {
Query: {
customQuery: async (_, __, context) => {
// Custom logic to fetch and return data
},
},
Mutation: {
customMutation: async (_, args, context) => {
// Custom logic to mutate and update data
},
},
};
In the above example, customQuery and customMutation are resolver functions for handling GraphQL queries and mutations. These functions can be as straightforward or complex as your application requires, providing a high degree of customization.
Read: Strapi's GraphQL Plugin: Setting Up And Querying Data With GraphQL
Leveraging GraphQL Typedefs
In Strapi v4, GraphQL typedefs play a crucial role in defining the structure and types of your GraphQL schema. By understanding and manipulating typedefs, you gain fine-grained control over how your data is represented and queried.
Defining GraphQL typedefs
Typedefs are typically organized within the api directory under the typedefs subdirectory. Here's an example of how you might define a type and its corresponding query in a Strapi v4 project:
# api/typedefs/customTypeDef.graphql
type CustomType {
id: ID!
name: String!
description: String
}
type Query {
getCustomData(id: ID!): CustomType
}
In this example, we've defined a CustomType with fields id, name, and description. Additionally, a Query type has been defined with a getCustomData resolver, which retrieves a CustomType based on the provided ID.
These typedefs serve as blueprints for your GraphQL schema, guiding the client and server on querying or mutating data structures.
Extending Resolvers in Strapi v4
Now that we have a foundational understanding of resolvers and GraphQL typedefs let's explore how to extend and customize these components to meet the specific requirements of your Strapi v4 project.
Creating custom queries
Extending resolvers to incorporate custom queries is a common requirement in many projects.
Whether fetching data from external APIs, aggregating data from multiple sources, or implementing complex business logic, custom queries empower you to tailor your data-fetching process.
Let's say we want to extend our previous example in Strapi v4 to include a custom query that fetches a list of custom data based on a specific condition. We can achieve this by modifying our resolver:
// api/resolvers/customResolver.js
module.exports = {
Query: {
customQuery: async (_, __, context) => {
// Custom logic to fetch and return data
},
customListQuery: async (_, { condition }, context) => {
// Custom logic to fetch a list of data based on the provided condition
},
},
Mutation: {
customMutation: async (_, args, context) => {
// Custom logic to mutate and update data
},
},
};
We have added a customListQuery resolver to our Query type, allowing us to fetch a list of custom data based on a specified condition.
Implementing custom mutations
In addition to custom queries, you might need to implement custom mutations for handling specific data manipulation tasks in Strapi v4.
Whether updating multiple records, triggering external processes, or implementing complex validation, custom mutations give you the flexibility to tailor your data manipulation logic.
Let's extend our resolver to include a custom mutation that updates the description of a custom data entry:
// api/resolvers/customResolver.js
module.exports = {
Query: {
customQuery: async (_, __, context) => {
// Custom logic to fetch and return data
},
customListQuery: async (_, { condition }, context) => {
// Custom logic to fetch a list of data based on the provided condition
},
},
Mutation: {
customMutation: async (_, args, context) => {
// Custom logic to mutate and update data
},
updateCustomDescription: async (_, { id, description }, context) => {
// Custom logic to update the description of a custom data entry
},
},
};
Now, with the addition of the updateCustomDescription resolver, we can easily update the description of a custom data entry by providing the ID and the new description as arguments.
Read: Understanding and Using Relations in Strapi
Advanced Techniques: Middleware and Authentication
As your Strapi v4 project grows, you may encounter scenarios where you must implement advanced techniques, such as middleware and authentication, within your custom resolvers.
Middleware in resolvers
Middleware functions in Strapi v4 allow you to perform actions before or after the execution of a resolver. This can be immensely useful for logging, data transformation, or authorization checks.
// api/resolvers/customResolver.js
module.exports = {
Query: {
customQuery: async (_, __, context) => {
// Custom logic to fetch and return data
},
customListQuery: async (_, { condition }, context) => {
// Custom logic to fetch a list of data based on the provided condition
},
},
Mutation: {
customMutation: async (_, args, context) => {
// Custom logic to mutate and update data
},
updateCustomDescription: async (_, { id, description }, context) => {
// Custom logic to update the description of a custom data entry
},
},
// Middleware example
// This middleware logs the input arguments before executing any resolver
async * beforeResolver(next, source, args, context) {
console.log('Resolver Arguments:', args);
yield next();
},
};
In this example, the beforeResolver middleware logs the input arguments before executing any resolver function. This can be invaluable for debugging and gaining insights into the data flow within your application.
Authentication in resolvers
Ensuring only authorized users can access or manipulate specific data is critical to application security. Strapi v4 makes it straightforward to implement authentication checks within your resolvers.
// api/resolvers/customResolver.js
module.exports = {
Query: {
customQuery: async (_, __, context) => {
// Custom logic to fetch and return data
},
customListQuery: async (_, { condition }, context) => {
// Custom logic to fetch a list of data based on the provided condition
},
},
Mutation: {
customMutation: async (_, args, context) => {
// Custom logic to mutate and update data
},
updateCustomDescription: async (_, { id, description }, context) => {
// Custom logic to update the description of a custom data entry
},
},
// Authentication example
// This middleware checks if the user is authenticated before allowing access to the resolver
async * beforeResolver(next, source, args, context) {
if (!context.state.user) {
throw new Error('Unauthorized: User must be authenticated');
}
yield next();
},
};
In this example, the beforeResolver middleware checks whether the user is authenticated before allowing access to any resolver function. This simple yet powerful authentication mechanism protects sensitive data and operations.
Read: Custom Controllers and Services in Strapi: Enhancing the Default Behavior
Conclusion
Strapi v4 empowers developers to build flexible and scalable applications with its extensible architecture and powerful features.
By mastering custom resolvers, you can take complete control of your data-handling logic, shaping it to suit the unique requirements of your project.
Whether you're building a small blog or a complex enterprise solution, the knowledge gained from this guide will serve as a solid foundation for harnessing the true power of Strapi v4.
Start building with Strapi v4, and witness the transformation of your web development experience. Stay tuned with Kapsys for more!