Optimizing Your Next.js Site On Vercel With CDN And Cache
- User Experience
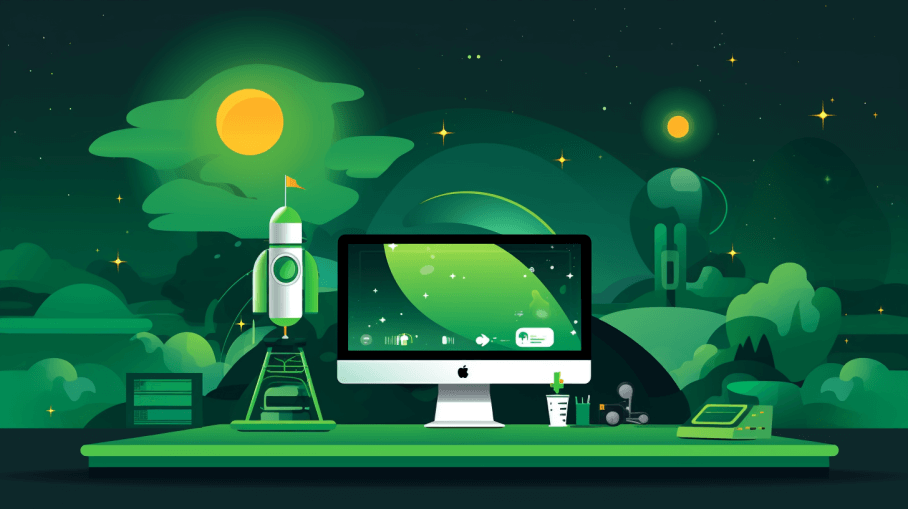
If you're using Next.js site on Vercel, you're already on the right track, but there are additional steps you can take to supercharge your site's performance.
Optimizing your website's performance in today's fast-paced digital landscape is more crucial than ever. User expectations are high, and the slightest delay in page loading can result in lost visitors and potential customers.
Join Kapsys as we delve into the world of Next.js site optimization on Vercel, focusing on the best practices and solutions, particularly leveraging Content Delivery Networks (CDN) and caching effectively to deliver lightning-fast web experiences.
Understanding Next.js
Before we dive into the optimization techniques, let's start with a brief overview of Next.js site and why it's a popular choice for building web applications. Next.js is a React framework that offers several benefits:
Server-Side Rendering (SSR): Next.js supports SSR out of the box, meaning your web pages are generated on the server and delivered as fully-rendered HTML to the client. This significantly improves page load times and SEO rankings.
Automatic Code Splitting: Next.js optimizes your application by splitting your JavaScript code into smaller chunks, loading only what's needed. This leads to faster initial page loads and smoother user experiences.
Efficient Client-Side Navigation: With its client-side routing, Next.js allows for lightning-fast transitions between pages, making your web application feel like a native app.
Developer Experience: It provides a fantastic developer experience with features like hot module reloading, automatic routing, and a rich ecosystem of plugins and extensions.
Now that we've established the benefits of Next.js, let's explore the best practices and solutions to optimize your Next.js site hosted on Vercel further.
Read: Serverless Functions in Next.js: A Practical Guide
Utilizing Content Delivery Networks (CDN)
A Content Delivery Network (CDN) is a distributed network of servers strategically placed worldwide to deliver web content to users quickly and efficiently. By integrating a CDN into your Next.js site hosted on Vercel, you can achieve the following advantages:
Reduced Latency: CDNs cache your site's assets and serve them from the server closest to the user. This minimizes the physical distance data needs to travel, resulting in lower latency and faster loading times.
Scalability: CDNs can handle traffic spikes and distribute the load, ensuring your site remains responsive even during high-demand periods.
Enhanced Security: CDNs often have built-in security features that protect your site from DDoS attacks, bot traffic, and other malicious activities.
To enable a CDN for your Next.js site on Vercel, add the following code to your project's configuration:
const withPlugins = require("next-compose-plugins");
const withOptimizedImages = require("next-optimized-images");
const withCDN = require("next-cdn");
module.exports = withPlugins(
[withOptimizedImages, withCDN],
// Your other Next.js configurations here
);
This code snippet ensures that your Next.js site is optimized with a CDN, improving content delivery to users across the globe.
Read: Getting Started With Next.js To Set Up A New Project
Effective Caching Strategies
Caching is a crucial aspect of optimizing your Next.js site. Properly configured caching can significantly reduce server load and improve page load times. Here are some caching strategies to consider:
Page Caching: Caching entire pages on the server or at the CDN level effectively reduces server load and improves load times. However, be cautious with dynamic content that needs to be updated frequently.
Client-Side Caching: Leverage browser caching to store assets like images, stylesheets, and scripts on the user's device. This minimizes the need to re-download these assets on subsequent visits, leading to faster page loads.
Cache-Control Headers: Use the Cache-Control HTTP header to specify caching directives for specific resources. You can set rules for how long assets should be cached, helping strike a balance between load times and content freshness.
Here's how to implement effective caching strategies for your Next.js site:
module.exports = {
async headers() {
return [
{
source: "/(.*)",
headers: [
{
key: "Cache-Control",
value: "public, max-age=31536000, must-revalidate",
},
],
},
];
},
// Other Next.js configurations
};
Adding these Cache-Control headers to your Next.js configuration will ensure that your site's assets are cached efficiently, reducing the need for repetitive server requests.
Cache Invalidation: Implement cache invalidation strategies to ensure that when you update your content, the changes are reflected immediately. This is particularly important for dynamic range or frequently changing data.
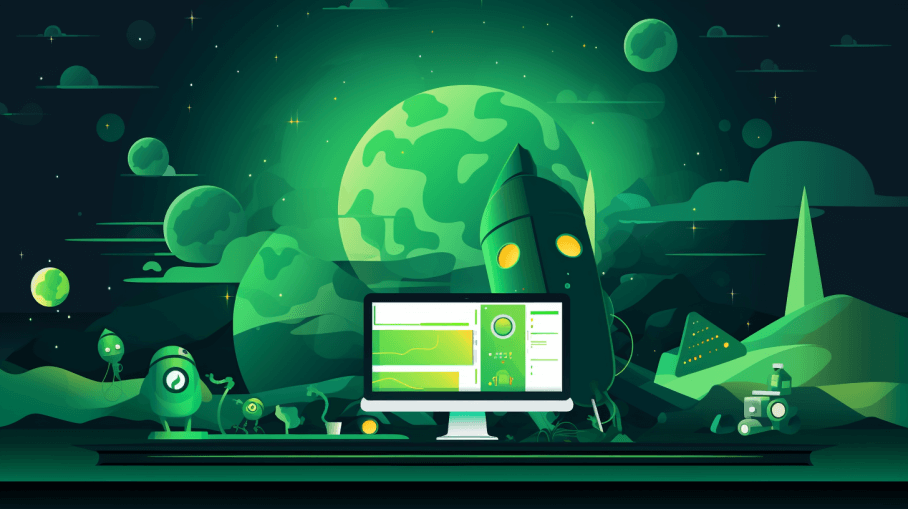
Code Splitting and Lazy Loading
Next.js sites are well-known for automatic code splitting, but you can take it further by implementing lazy loading. Lazy loading allows you to load JavaScript code and assets only when needed, reducing the initial page load time. Here's how you can do it:
Dynamic Imports: Use dynamic imports to load modules on demand. This way, you can load only the JavaScript code necessary for the current page, improving the user's experience.
For dynamic imports, you can use the following code:
// Dynamic import example
const MyComponent = dynamic(() => import("../components/MyComponent"));
React Suspense and React.lazy: Take advantage of React's Suspense API and the React.lazy function to load components lazily. This can help reduce the initial bundle size and improve page load times.
- Prefetching: Implement prefetching for links that users are likely to click. Next.js supports automatic prefetching by adding a simple attribute to your links. This way, resources are preloaded in the background, ensuring a smooth transition to the next page.
Image Optimization
Images are often the heaviest assets on a webpage. Optimizing images is crucial for a fast-loading site. Here are some strategies for image optimization in Next.js site:
Use the 'next/image' Component: Next.js provides the 'next/image' component, automatically optimizing images. It offers lazy loading, responsive image sizes, and automatic format conversion, reducing the load time and improving the user experience.
Here's how to use it:
import Image from "next/image";
// ...
<Image
src="/your-image.jpg"
alt="Your Image"
width={500}
height={300}
/>
Choose the Right Image Format: Select the appropriate image format for different use cases. For photographs, use JPEG; for transparent images, use PNG or WebP. WebP is an excellent choice for modern browsers as it offers a better compression rate.
Compress Images: Before uploading images to your site, compress them to reduce file sizes. Tools like ImageOptim and TinyPNG can help you achieve significant savings in image size without compromising quality.
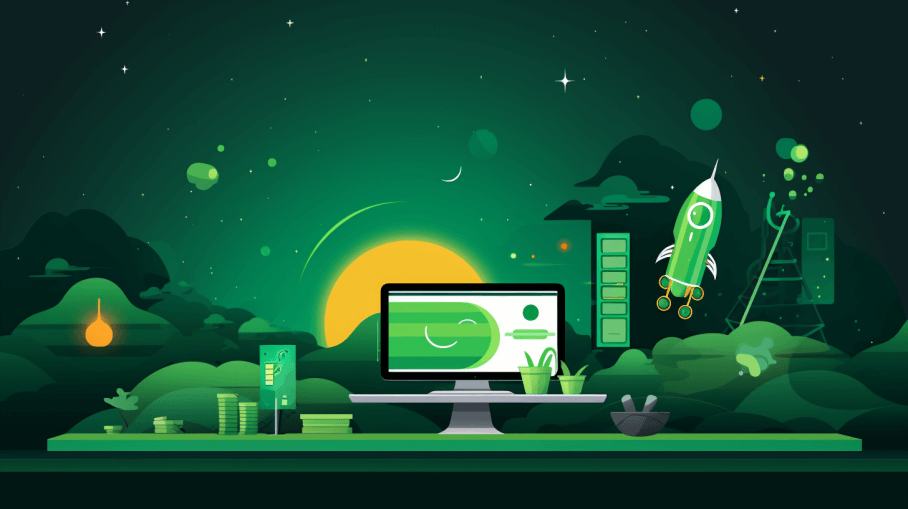
Database and API Caching
If your Next.js site relies on databases or APIs, consider implementing caching for these data sources. Caching can dramatically reduce the response time of your API calls and database queries. Here's how:
In-Memory Caching: Utilize in-memory caching solutions like Redis to store frequently accessed data in memory. This reduces the need to query the database for the same data repeatedly.
Here's how you can implement it:
const redis = require("redis");
const client = redis.createClient();
// ...
client.get("your-key", (err, data) => {
if (err) throw err;
if (data !== null) {
// Data found in cache, use it
res.send(JSON.parse(data));
} else {
// Data not in cache, query the database
// Store the result in cache for future use
client.setex("your-key", 3600, JSON.stringify(data));
// ...
}
});
Response Caching: Implement response caching for API endpoints. You can use HTTP caching headers to specify how long responses should be cached by the client or intermediary caches.
- Query Optimizations: Optimize your database queries by using indexes, avoiding unnecessary JOIN operations, and selecting only the needed data. Well-optimized questions are faster and put less strain on your database server.
Serverless Functions
Leveraging serverless functions can help offload server tasks, reducing the load on your primary server and improving overall site performance. With Vercel, you can easily create and deploy serverless functions using their integrated solution.
Here are some use cases for serverless functions:
API Endpoints: Move API endpoints to serverless functions to reduce the load on your primary server. These functions can handle authentication, data processing, or server-side logic.
Server-Side Rendering (SSR): You can use serverless functions to implement server-side rendering on specific pages, enabling dynamic content delivery while maintaining fast load times.
Scheduled Tasks: Use serverless functions for scheduled tasks, such as generating and caching data, sending emails, or other background processes.
Here's a simple example of creating a serverless function:
module.exports = (req, res) => {
res.status(200).json({ message: "Hello from the serverless function!" });
};
By implementing serverless functions, you can offload server tasks, reducing the load on your primary server and improving overall site performance.
Minification and Compression
Minifying and compressing your assets, including JavaScript, CSS, and HTML, is a straightforward way to reduce load times. Minification removes unnecessary characters and whitespace, while compression reduces file sizes for faster transmission.
Here's how to apply minification and compression to your Next.js site:
module.exports = {
// ...
experimental: {
optimizeCss: true,
optimizeImages: true,
},
};
By enabling automatic minification and compression during deployment, your Next.js site's assets will have smaller file sizes, resulting in faster page loads.
Monitoring and Continuous Optimization
Once you've implemented the optimization techniques mentioned above, monitoring your Next.js site's performance is essential. Optimization is an ongoing process, and there are always ways to make your site even faster. Here's what you can do to ensure continuous improvement:
Performance Metrics: Regularly measure and analyze key performance metrics, such as page load times, page speed scores, and server response times.
Error Tracking: Monitor for errors and issues impacting your site's performance. Implement error-tracking solutions to identify and address problems as they arise.
A/B Testing: Use A/B testing to experiment with different optimization strategies and choose the most effective ones based on actual user data.
User Feedback: Consider user feedback and comments regarding your site's performance. Users often notice issues that can be missed through automated monitoring.
Vercel Analytics: Take advantage of Vercel's analytics features to gain insights into how your site is performing and where improvements can be made.
Read: Best Practices For Safety And Privacy Vercel Deployment
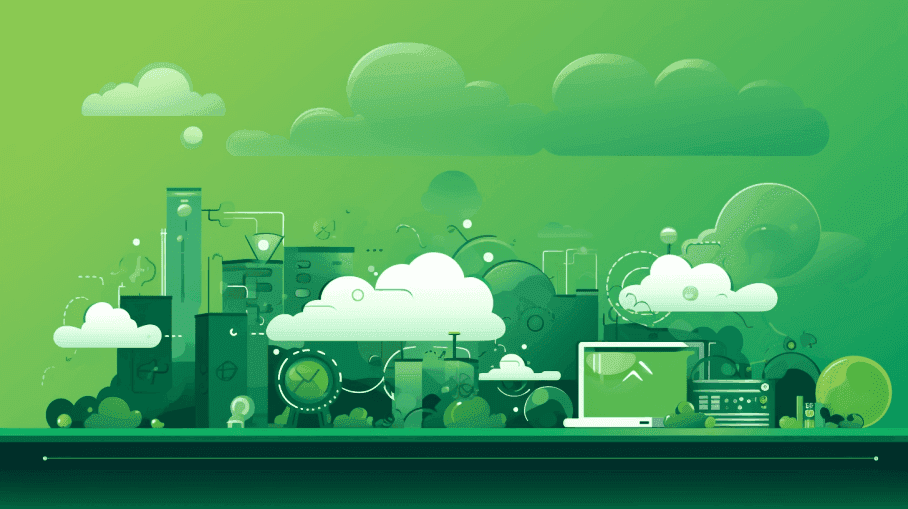
Conclusion
Optimizing your Next.js site on Vercel with CDN and caching is crucial for a responsive user experience. You'll create a faster, more efficient website by following best practices like utilizing CDNs, efficient caching, code splitting, and image optimization.
Continuous optimization is critical. Monitor your site's performance, gather feedback, and adapt your strategies to exceed visitor expectations. These techniques equip your Next.js site to deliver lightning-fast experiences, setting you apart in the competitive online world.
A faster site benefits users and boosts search engine rankings and conversions. Invest in optimization for lasting rewards.
Check out Kapsys blog to learn how to improve your website capabilities!