How To Build A Multilingual Site With Next.js
- User Experience
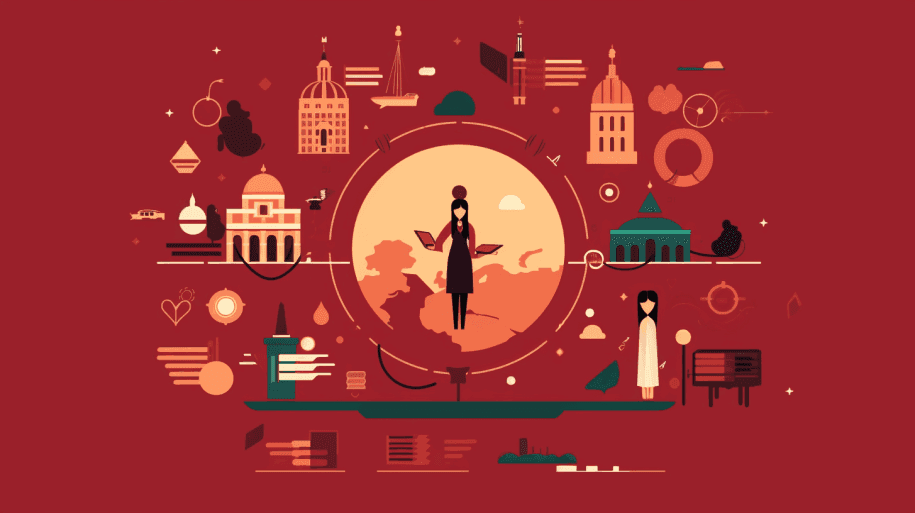
Whether you're a business owner looking to expand your online presence, a content creator aiming for a global audience, or a developer looking to enhance your skills, building a multilingual site can be a valuable asset.
In today's interconnected world, the demand for multilingual sites is rising. So, join Kapsys in this comprehensive guide as we explore how to create a multilingual site using Next.js as your primary framework.
Why Choose Next.js for a Multilingual Site?
Before diving into the technical details, it's essential to understand why Next.js is an excellent choice for building a multilingual site.
Next.js is a popular React framework that offers a range of features that make it ideal for this purpose:
SEO-Friendly: Next.js is known for its excellent SEO capabilities, which are crucial for a global audience. Search engines love Next.js applications, making ranking higher in search results more accessible for your site.
Server-Side Rendering (SSR): SSR is a significant advantage when dealing with multilingual content. Next.js allows you to render pages on the server, improving performance and enabling efficient content delivery to users worldwide.
Code Splitting: Next.js automatically generates optimized bundles for different pages, ensuring your site loads quickly, regardless of the user's location.
Internationalization (i18n) Support: Next.js strongly supports internationalization, making it easier to manage and serve content in multiple languages.
Large and Active Community: With a large community and continuous development, you can find plenty of resources, plugins, and solutions for any multilingual challenges.
Now that you know why Next.js is an excellent choice, let's dive into the prerequisites to build a multilingual site.
Prerequisites for Building a Multilingual Site
To build a multilingual site with Next.js, you need the following essential prerequisites:
Basic Knowledge of JavaScript: A solid understanding of JavaScript is necessary, as Next.js is built on React, and you'll be writing JavaScript code.
Node.js and npm: Node.js is required to run the development server and manage project dependencies. npm is used to install and manage packages for your Next.js project.
Next.js Installed: You should have Next.js installed on your development machine. You can create a new project using the Next.js CLI or npm.
Text Editor or IDE: You'll need a code editor or IDE for writing and editing your code, such as Visual Studio Code.
Basic Command-Line Knowledge: Familiarity with basic command-line operations is essential for navigating your project directory, running scripts, and managing dependencies.
These are the most necessary prerequisites for building a multilingual site using Next.js. While optional, a basic understanding of HTML, CSS, and SEO principles can also be helpful.
Step 1: Setting Up Your Next.js Project
To build a multilingual site, set up a Next.js project. You can do this by using the official Next.js documentation as a guide. Once you have your Next.js project up and running, you're ready to proceed to the next steps.
Read: Getting Started With Next.js To Set Up A New Project
Step 2: Choose the Right Multilingual Strategy
Before you start coding, decide on your multilingual strategy for building your multilingual site. There are primarily two approaches:
1. Multi-Page Approach:
In this method, each language is treated as a separate standalone website. Each language version of your site resides in a different directory. For example:
English: https://yoursite.com/en
Spanish: https://yoursite.com/es
French: https://yoursite.com/fr
This approach is beneficial for SEO as each language version is a separate website, making it easier for search engines to index your content. However, it can be challenging to maintain as you must duplicate pages and assets for each language.
Read: SEO Best Practices In Next.js Applications: Search-Engine Friendly
2. Single-Page Approach:
In this approach, you keep all languages on a single page, and the content is dynamically loaded based on the user's language preference. For example:
https://yoursite.com with language options available for users to select.
This approach is easier to maintain but may require more complex code for language handling. We will focus on the single-page strategy for our guide on building a multilingual site.
Step 3: Integrate Internationalization (i18n)
To make your Next.js project into a multilingual site, you'll need to integrate internationalization (i18n) support. A popular library for this purpose is next-i18next. Here's how to set it up:
Install next-i18next:
npm install next-i18next
Create a Configuration File: In your project's root directory, create a next-i18next.js configuration file with the following content:
// next-i18next.js
module.exports = {
i18n: {
locales: ['en', 'es', 'fr'], // Add your supported languages here
defaultLocale: 'en', // Set your default language
},
// Add any additional configuration options here
};
Initialize next-i18next: In your Next.js pages/_app.js file, initialize next-i18next with your configuration:
// pages/_app.js
import { appWithTranslation } from 'next-i18next';
import { i18n } from '../i18n'; // Replace with your i18n configuration path
function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />;
}
export default appWithTranslation(MyApp);
This sets up the foundation for handling multilingual content.
Step 4: Create Language Files
Now, for your multilingual site, you must provide your content translations. For each language you support, create a JSON file with translations. For example, create en.json, es.json, and fr.json for English, Spanish, and French, respectively.
en.json:
{
"welcome": "Welcome to our website!",
"aboutUs": "About Us",
"contact": "Contact Us"
}
es.json:
{
"welcome": "¡Bienvenido a nuestro sitio web!",
"aboutUs": "Sobre Nosotros",
"contact": "Contáctenos"
}
fr.json:
{
"welcome": "Bienvenue sur notre site Web !",
"aboutUs": "À propos de nous",
"contact": "Contactez-nous"
}
These JSON files contain key-value pairs for all the content you want to translate.
Step 5: Implement Language Switching
To allow users to switch between languages, you must create a language switcher component in your multilingual site. This component should be available on all pages to enable users to change their language preferences.
Create a new component, LanguageSwitcher.js, with the following code:
import { useTranslation } from 'next-i18next';
const LanguageSwitcher = () => {
const { i18n } = useTranslation();
const changeLanguage = (locale) => {
i18n.changeLanguage(locale);
};
return (
<div>
<button onClick={() => changeLanguage('en')}>English</button>
<button onClick={() => changeLanguage('es')}>Español</button>
<button onClick={() => changeLanguage('fr')}>Français</button>
</div>
);
};
export default LanguageSwitcher;
Now, import and include this component in your multilingual site layout or navigation.
Read: Serverless Functions in Next.js: A Practical Guide
Step 6: Translate Your Content
With the language files in place and the language switcher component ready, it's time to translate your content for a multilingual site. To do this, use the useTranslation hook from next-i18next. Here's an example of how to use it in your components:
import { useTranslation } from 'next-i18next';
const Home = () => {
const { t } = useTranslation('common'); // 'common' is the translation namespace
return (
<div>
<h1>{t('welcome')}</h1>
<p>{t('aboutUs')}</p>
<p>{t('contact')}</p>
</div>
);
};
export default Home;
By calling useTranslation('common'), you indicate that you want to use the translations from the 'common' namespace. This allows you to keep translations organized.
Repeat this process for all your pages and components to ensure all content is translated correctly for a multilingual site.
Step 7: Handle Dynamic Routes
If your multilingual site has dynamic routes, you must ensure that these routes also support multiple languages. For example, if you have a blog with posts, each post should be accessible in every supported language.
You can achieve this by creating a dynamic route for the post and using the getStaticPaths and getStaticProps functions. Here's an example of how to set up multilingual dynamic routes:
// pages/blog/[slug].js
import { useTranslation } from 'next-i18next';
// Add your dynamic route component logic here
Step 8: SEO Considerations
Optimizing your multilingual site for SEO is crucial to reaching a global audience effectively.
Here are some tips:
Hreflang Tags: Use hreflang tags to inform search engines about your pages' language and regional targeting. Include these tags in the <head> section of your HTML document.
<link rel="alternate" hreflang="en" href="https://yoursite.com/en/page" />
<link rel="alternate" hreflang="es" href="https://yoursite.com/es/page" />
<link rel="alternate" hreflang="fr" href="https://yoursite.com/fr/page" />
Sitemap: Create a sitemap for each language version of your site. Submit these sitemaps to search engines to ensure proper indexing.
Localized Content: Provide localized metadata, such as titles and descriptions, for each language version of your pages.
Structured Data: Use structured data markup (e.g., JSON-LD) for multilingual sites to help search engines understand your content.
Step 9: Testing and Quality Assurance
Before launching your multilingual site, thoroughly test it to ensure all translations are accurate and the language switcher works as expected. Also, check for any visual or functional issues that might arise from text length differences between languages.
Consider conducting user testing to gather feedback from individuals who are native speakers of the languages you support. This will help you identify any cultural or linguistic nuances you may have missed.
Step 10: Deployment and Monitoring
Once you've tested and verified that your multilingual site works as intended, it's time to deploy it to your hosting platform. Whether you host it on Vercel, Netlify, or another service, ensure that the server configuration supports internationalization.
After deployment, regularly monitor your site's performance and user interactions. Use analytics tools to understand user behavior and gather feedback to make continuous improvements.
Common Problems and Troubleshooting
Troubleshooting is a standard part of the development process, and when working on a multilingual site with Next.js, you may encounter various issues.
Here are some troubleshooting tips to help you address common challenges:
Missing translations
Sometimes, translations may need to be completed for specific languages.
Solution: Ensure all translations are provided for each supported language. You can create a system to handle missing translations gracefully, showing the default language text if a translation is missing.
Text overflow and styling issues
Text in different languages may have varying lengths, causing text-overflow and styling problems.
Solution: Use CSS techniques like ellipsis or responsive design to handle varying text lengths. Test with authentic content to ensure that it displays correctly in all languages.
SEO challenges
SEO can be tricky with multilingual sites. Hreflang tags, duplicate content issues, and regional targeting can be challenging to manage.
Solution: Implement hreflang tags, canonical URLs, and structured data correctly. Use SEO tools and check for indexing issues. Monitor SEO metrics to address problems proactively.
Dynamic content and routing
Handling dynamic content and routes for different languages can be complex.
Solution: Utilize Next.js's getStaticPaths and getStaticProps for dynamic routes and follow best practices for localized routing. Ensure the same content is available in all languages.
Optimizing performance
Multilingual sites can need faster performance due to the need to load content in multiple languages.
Solution: Implement server-side rendering (SSR) and code splitting to optimize performance. Use a content delivery network (CDN) for assets to reduce latency.
Remember that building and maintaining a multilingual site is an ongoing process that requires attention to detail, continuous improvement, and a user-centric approach.
Conclusion
Building a multilingual site with Next.js empowers you to connect with a global audience efficiently. With the robust features of Next.js and the support of the next-i18next library, you can create a seamless multilingual experience.
Prioritize SEO, testing, and monitoring to maximize your multilingual site's potential. Remember that success hinges on delivering culturally relevant content to your international visitors.
By leveraging Next.js, you can make this a reality. Whether you're a developer, business owner, or content creator, embarking on your multilingual journey with Next.js is a wise choice.
Keep up with Kapsys to learn all about software development!