Integrating with External APIs in Next.js: A Comprehensive Guide
- User Experience
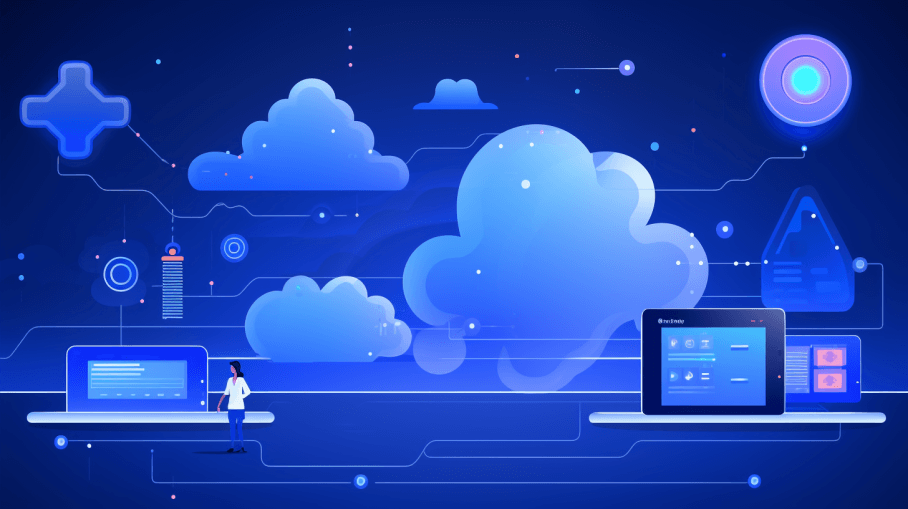
Integrating external APIs in Next.js is essential for building dynamic and data-driven applications. Next.js, the primary framework for many developers, offers powerful features like server-side rendering, static site generation, and a robust ecosystem for feature-rich web applications.
Join Kapys in this guide as we explore the process of integrating external APIs in your Next.js projects.
Understanding the Importance of External APIs
Before diving into the technical aspects of incorporating external APIs in Next.js, let's take a moment to understand why this is essential.
External APIs, or Application Programming Interfaces, are gateways to accessing data and functionality from other platforms and services. These APIs allow your application to interact with external systems, such as social media platforms, databases, payment gateways, etc.
Here are some reasons why integrating with external APIs is crucial in modern web development:
Access to Rich Data: External APIs offer access to a wealth of data that can enrich your application. You can fetch weather forecasts, stock market data, user profiles, and more.
Reduced Development Time: Why reinvent the wheel when you can leverage existing APIs to provide the necessary functionality? This can significantly reduce development time and costs.
Real-time Updates: External APIs can provide real-time updates, ensuring that your application always displays the most current information to users.
Expanded Functionality: By integrating with external APIs, you can add new features to your application without building everything from scratch.
Now that we've established the importance of external APIs, let's get into the specifics of integrating them into your Next.js project.
Read: Choosing The Best Next.js CMS in 2023
Getting Started with External API Integration in Next.js
Integrating external APIs in Next.js primarily involves making HTTP requests to the API endpoints, receiving responses, and processing the data as needed. To start, follow these steps:
1. Create a new Next.js project
If you haven't already, create a new Next.js project using the following command:
npx create-next-app my-next-app
This command creates a new Next.js project with the necessary files and dependencies.
2. Fetching data from an external API
Next, you'll need to fetch data from an external API. You can use built-in JavaScript functions like fetch or libraries like axios for this purpose. Let's explore both options:
Using the fetch function
import React, { useState, useEffect } from 'react';
function ExternalApiFetch() {
const [data, setData] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch('https://api.example.com/data');
if (response.ok) {
const json = await response.json();
setData(json);
} else {
console.error('Failed to fetch data');
}
} catch (error) {
console.error('Error:', error);
}
};
fetchData();
}, []);
if (!data) return <div>Loading...</div>;
return (
<div>
{/* Display the data */}
</div>
);
}
export default ExternalApiFetch;
In this example, we use the fetch function to make an asynchronous request to an external API and then process the response.
Using Axios
npm install axios
import React, { useState, useEffect } from 'react';
import axios from 'axios';
function ExternalApiAxios() {
const [data, setData] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await axios.get('https://api.example.com/data');
setData(response.data);
} catch (error) {
console.error('Error:', error);
}
};
fetchData();
}, []);
if (!data) return <div>Loading...</div>;
return (
<div>
{/* Display the data */}
</div>
);
}
export default ExternalApiAxios;
The code above demonstrates how to use the Axios library to fetch data from an external API. It simplifies making HTTP requests and handling responses.
Read: Serverless Functions in Next.js: A Practical Guide
3. Display the data
Once you've successfully fetched data from an external API, you can display it in your Next.js application. You can render the data using JSX, creating components and templates that suit your project's design.
You can lay the ground for your actions using these three easy steps. Now, as we begin with our process of integrating external API, let's move to the next section of this guide.
Handling External API Authentication and Authorization
Many external APIs require authentication to access their data or services. Here are the standard methods for handling authentication in Next.js:
API keys
Some APIs require an API key. You can store this key in a secure environment variable and access it in your Next.js application. For example, you can use the dotenv package to manage environment variables.
npm install dotenv
Create a .env file in the root of your project and add your API key:
API_KEY=your_api_key_here
Then, in your Next.js code, access the API key like this:
const apiKey = process.env.API_KEY;
OAuth and tokens
You'll need to implement the authentication flow for APIs that use OAuth or token-based authentication. This typically involves sending a request to an authentication endpoint, receiving a token, and using that token in subsequent API requests.
Next.js provides a straightforward way to handle authentication by using middleware. You can create middleware functions to check authentication before allowing access to specific routes or components. A standard package used for this purpose is next-auth.
npm install next-auth
After installing next-auth, you can configure it to handle authentication with your chosen external API.
// pages/api/auth/[...nextauth].js
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.YourChosenProvider({
clientId: process.env.CLIENT_ID,
clientSecret: process.env.CLIENT_SECRET,
}),
],
session: {
jwt: true,
},
});
Remember to replace YourChosenProvider, CLIENT_ID, and CLIENT_SECRET with the actual provider and credentials you are using.
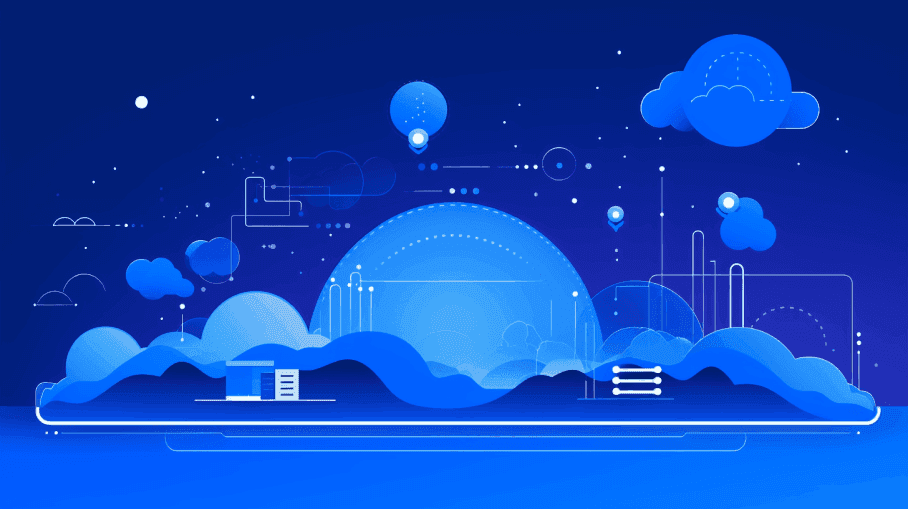
Error Handling and Data Validation
Handling errors and validating data from external APIs are critical aspects of integration. You must anticipate and gracefully handle errors when working with external data sources, ensuring a smooth user experience.
Here are some best practices for error handling and data validation:
Status Codes: Check the HTTP status code in the API response to determine if the request was successful or encountered an error. Typically, status codes in the 4xx range indicate client errors, and status codes in the 5xx range indicate server errors.
Error Messages: Most APIs provide error messages in the response data. Parse these messages to display meaningful error information to users.
Data Validation: Validate the data you receive from external APIs. Ensure the data structure and content match your expectations before using it in your application. This can help prevent issues like null pointer errors or unexpected behavior.
Fallback Data: In case of API failures or errors, have fallback data or error messages to display to users. This ensures your application remains functional even when external data sources are unavailable.
You must anticipate and gracefully handle errors when working with external data sources, ensuring a smooth user experience. With these practices, you’ll be ready to face any challenge.
Caching Data from External APIs
Caching external API responses can improve the performance and reliability of your Next.js application. Caching involves storing API responses for a specified period, so you don't have to fetch the same data repeatedly. It can reduce the load on both your server and the external API server.
Next.js provides several options for caching data, including:
Client-Side Caching: Using local or session storage technologies, you can cache data on the client side. This allows you to store data on the user's device for a limited time.
Server-Side Caching: Next.js supports server-side caching using mechanisms like memoization or caching libraries. By caching API responses on the server, you can serve the same data to multiple users without making redundant API requests.
Content Delivery Networks (CDNs): You can leverage CDNs to cache and serve API responses. CDNs are highly effective at reducing latency and speeding up user data delivery.
To implement caching effectively, you'll need to consider factors such as cache expiration, cache validation, and cache clearing when the data on the external API changes.
Read: Optimizing Your Next.js Site On Vercel With CDN And Cache
Rate Limiting and Security
When integrating with external APIs, it's essential to be mindful of rate limiting and security considerations.
Many APIs have rate limits to prevent abuse and protect their resources. Violating these limits can result in temporary or permanent suspension of access.
Here are some tips for dealing with rate limits and enhancing security when working with external APIs:
Understand Rate Limits: Study the API's rate limit documentation to know how many requests you can make within a specific time frame. Comply with these limits to ensure continued access.
API Key Security: If you use an API key for authentication, keep it secure. Never expose your API key in client-side code or public repositories. Use environment variables to hide sensitive information.
Retrying Failed Requests: Implement retry mechanisms for failed API requests. This can help avoid disruptions in your application due to transient network issues or occasional API downtime.
CORS (Cross-Origin Resource Sharing): Be aware of CORS policies and restrictions when making API requests from the client side. You may need to configure the API server to allow requests from your domain.
Data Privacy: Ensure you handle user data securely and adhere to data protection regulations. If you're dealing with user data, encrypt it during transmission and storage.
Integrating with Specific External APIs
The process of merging with external APIs can vary depending on the API you're working with. Different APIs have their endpoints, authentication methods, and data formats. It's crucial to consult the API's documentation for specific integration instructions.
To give you an idea of how this works in practice, let's explore integrating with a few common external APIs:
RESTful APIs
REST (Representational State Transfer) is a popular architectural style for designing networked applications. Most RESTful APIs use standard HTTP methods like GET, POST, PUT, and DELETE to interact with resources. As demonstrated earlier, you can interact with a RESTful API using the fetch function.
GraphQL APIs
GraphQL is an alternative to REST that allows you to query only the data you need. To integrate with a GraphQL API in Next.js, you can use the graphql-request library or Apollo Client. These libraries provide tools for sending GraphQL queries and mutations to the API.
Read: Strapi's GraphQL Plugin: Setting Up And Querying Data With GraphQL
Social media APIs
Many social media platforms, such as Facebook, Twitter, and Instagram, offer APIs to access user data and perform actions like posting updates. Integrating these APIs often involves OAuth authentication and specific API requests to read or update data.
Payment gateway APIs
Payment gateway APIs, like Stripe or PayPal, enable you to process payments securely. To integrate with payment gateways, you'll typically need to set up webhooks, handle payment processing, and ensure data security.
Each of these APIs has its specific integration requirements, and it's crucial to consult their documentation and follow best practices to ensure a smooth integration.
Conclusion
Integrating external APIs is essential for modern web applications. Next.js provides a strong foundation for dynamic, data-driven projects, making it a top choice for developers.
Following this guide's steps, you'll confidently integrate external APIs into your Next.js applications. Remember to prioritize error handling, data validation, caching, rate limiting, and security for smooth and secure operations.
Exploring and integrating different external APIs will enhance your skills, enabling the creation of versatile and feature-rich web applications. Consult the API documentation for specific integrations and embrace innovation with the data you access.
Check out Kapsys blog for more actionable insights!