Next js Page Transitions: Creating Smooth Navigation And Animation
- User Experience
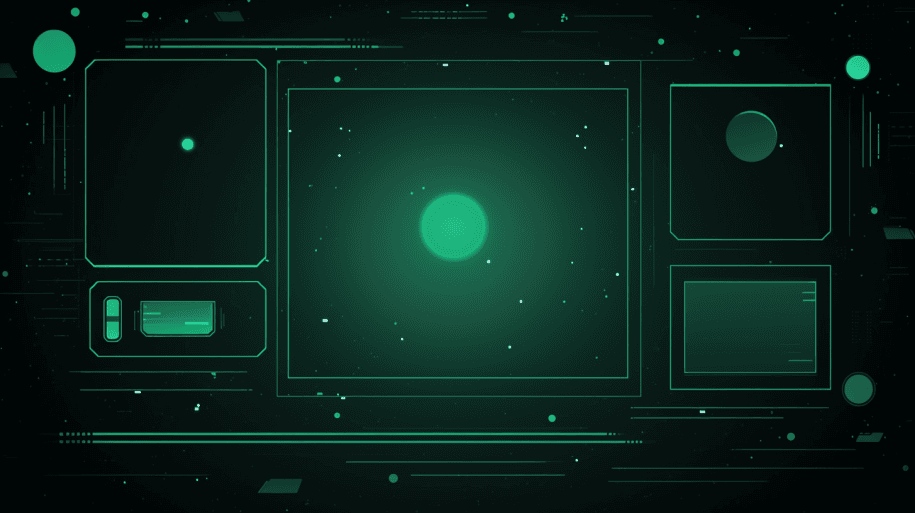
Elevating user experience is a top priority in web development, and a highly effective method for achieving this is by incorporating Next js page transitions.
Next.js, a widely acclaimed React framework, serves as an invaluable platform for crafting seamless and captivating navigation, as well as page transitions.
Join Kapsys as we explore the enchanting realm of CSS animations, coupled with the capabilities of Next.js, to elevate your website's user experience with captivating Next js page transitions.
What Is Next.js?
Next.js is a popular open-source JavaScript framework for building web applications, particularly single-page and server-rendered applications.
Developed by Vercel (formerly Zeit), Next.js is built on top of React, another widely used JavaScript library for building user interfaces.
Next.js provides several key features and advantages that make it a powerful choice for web development, including Next js page transitions:
Server-Side Rendering (SSR): Next.js offers built-in support for server-side rendering, improving performance and SEO.
Routing: Next.js provides an intuitive routing system for setting up and navigating between pages.
Automatic Code Splitting: Next.js automatically code-splits your application to reduce initial page load times.
Static Site Generation (SSG): It supports static site generation for pre-building pages, making it suitable for content that only changes sometimes.
Vercel Integration: Next.js seamlessly integrates with the Vercel hosting platform for easy deployment and management.
Developers and companies widely adopt Next.js for building a wide range of web applications, including e-commerce sites, blogs, marketing websites, and complex web applications.
Why Next js Page Transitions Matter
Before delving into the technical aspects of implementing Next js page transitions, it's crucial to understand why they matter. Here are a few compelling reasons:
1. Improved user engagement
Next js page transitions, such as content animations, add an element of delight to your website, making interactions more engaging and enjoyable for users. The subtlety of well-designed animations can keep visitors glued to your site.
2. Seamless navigation
Smooth transitions between pages create a sense of continuity and seamlessness, reducing the perception of loading times. Users appreciate it when they can effortlessly navigate your site with Next js page transitions.
3. Storytelling
Next js page transitions can guide users through your content, telling a visual story. Whether scrolling, fading, sliding, or other effects, Next js page transitions can make your website's narrative more compelling.
Read: Building a Static Site with Next.js: Explore the Advantages of SSG
Before We Begin
To work effectively with Next s transitions, you will need the following prerequisites:
JavaScript Knowledge: A strong understanding of JavaScript, as Next.js is built on React, and JavaScript is the primary language for both the framework and animations.
React Basics: Familiarity with React components, state, and props.
Node.js and npm/Yarn: Node.js, npm, or Yarn are essential for managing dependencies and running Next.js projects.
CSS and CSS Animations: Good knowledge of CSS, including CSS animations, keyframes, and transitions for creating transitions.
These foundational skills and tools will enable you to get started with Next.js and create Next js page transitions effectively.
Getting Started with Next.js
If you're unfamiliar with Next.js, it's a React framework that allows easy server-side rendering, routing, and efficient performance optimization. To use Next.js for your Next js page transitions, you'll need to set up a Next.js project.
You can create a new Next.js application by running the following command:
npx create-next-app my-nextjs-app
Once your project is set up, you can implement Next js page transitions.
Read: Getting Started With Next.js To Set Up A New Project
CSS Animate Fade-In Transition
One of the most common Next js page transitions is the fade-in effect. This simple yet effective animation adds a touch of elegance to your website. We'll guide you through creating a CSS animate fade-in transition in Next.js.
1. Create a CSS File
First, create a CSS file for your animations. In your Next.js project, create a new CSS file, e.g., animations.css.
2. Define the CSS Animation
In your animations.css file, define the CSS animation for the fade-in effect. Here's an example:
.fade-in {
opacity: 0;
animation: fadeIn 1s ease-in-out forwards;
}
@keyframes fadeIn {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
This CSS code sets the initial opacity to 0 and gradually increases it to 1, creating a smooth fade-in effect with Next js page transitions.
3. Import CSS in Next.js
Next, import your animations.css file into your Next.js component, where you want to use the fade-in animation with Next js page transitions.
import React from 'react';
import '../styles/animations.css'; // Adjust the path as needed
function MyComponent() {
return (
<div className="fade-in">
{/* Your content goes here */}
</div>
);
}
export default MyComponent;
By applying the fade-in class to an element, you trigger the CSS animation when that element is rendered with Next js page transitions.
Read: How To Implement Content Previews in Next.js
Next js Page Transitions
Now, let's take a step further and explore how to create Next js page transitions.
1. Create a layout component
In Next.js, you can create a layout component that wraps your pages and controls the page transitions. Here's an example of a simple layout component:
// components/Layout.js
import { useRouter } from 'next/router';
function Layout({ children }) {
const router = useRouter();
return (
<div className="page-transition">
{children}
</div>
);
}
export default Layout;
2. Define page transitions
In your layout component, you can define page transitions using CSS animations. For instance, you can create a slide-in effect when navigating to a new page:
/* animations.css */
.page-transition {
animation: slideIn 0.5s ease-in-out both;
}
@keyframes slideIn {
from {
transform: translateX(100%);
}
to:
transform: translateX(0);
}
}
In this example, the slide animation slides in the content from the right when transitioning between pages with Next js page transitions.
3. Apply layout component
To apply the layout component to your pages, wrap your pages with it:
// pages/_app.js
import Layout from '../components/Layout';
function MyApp({ Component, pageProps }) {
return (
<Layout>
<Component {...pageProps} />
</Layout>
);
}
export default MyApp;
With this setup, all your pages will use the defined page transitions automatically with Next js page transitions.
Read: Choosing An Architecture Pattern for Next.js Applications
Enhancing Navigation with Next.js
Next.js offers various tools to enhance navigation with Next js page transitions. Here are a few techniques to consider:
1. Link component
Next.js provides the <Link> component for client-side navigation with Next js page transitions. It pre-fetches linked pages for a smoother transition. Here's how to use it:
import Link from 'next/link';
function MyComponent() {
return (
<Link href="/other-page">
<a>Go to Other Page</a>
</Link>
);
}
2. Route change events
You can also leverage route change events in Next.js to add custom animations or loading indicators during navigation with Next js page transitions. For instance, you can display a loading spinner when the user clicks on a link:
import { useRouter } from 'next/router';
function MyComponent() {
const router = useRouter();
const handleLinkClick = () => {
router.events.on('routeChangeStart', () => {
// Display loading indicator
});
router.events.on('routeChangeComplete', () => {
// Hide loading indicator
});
router.events.on('routeChangeError', () => {
// Handle error
});
};
return (
<a href="/other-page" onClick={handleLinkClick}>
Go to Other Page
</a>
);
}
By utilizing these route change events, you can add dynamic animation transitions and loading indicators to improve user experience with Next js page transitions.
Read: How To Build A Multilingual Site With Next.js
Best Practices for Next js page transitions
To make the most of Next js page transitions, consider the following best practices:
1. Keep animations subtle
While animations can enhance user experience, excessive or distracting animations may annoy users. Keep your animations subtle and purposeful with Next js page transitions.
2. Optimize for performance
Heavy animations can slow down your website. Ensure your animations are optimized for performance to maintain fast loading times with Next js page transitions.
3. Test on multiple devices
Test your animations on various devices and browsers to ensure they work smoothly everywhere with Next js page transitions.
4. Provide fallbacks
In case animations are not supported in specific browsers or for users with accessibility needs, make sure your content remains accessible and functional with Next js page transitions.
5. A/B Testing
Consider running A/B tests to determine which animations resonate best with your audience and drive engagement with Next js page transitions.
By following these best practices, you can create Next js page transitions that not only captivate your audience but also provide a seamless and enjoyable user experience while ensuring accessibility and performance are optimized.
Read: Choosing The Best Next.js CMS in 2023
Conclusion
Next js page transitions can transform your website from ordinary to extraordinary.
By adding subtle yet captivating animations to your pages and implementing seamless navigation with page transitions, you can significantly improve user engagement and the overall user experience with Next js page transitions.
Remember to keep your animations purposeful, optimize for performance, and test thoroughly to ensure they work well on all devices. With the power of Next.js and CSS animations, you can create a website that stands out from the crowd and keeps visitors coming back for more.
Keep up with Kapsys to take your development process to the next level!