How To Build A Simple Blog With Next.js Markdown
- User Experience
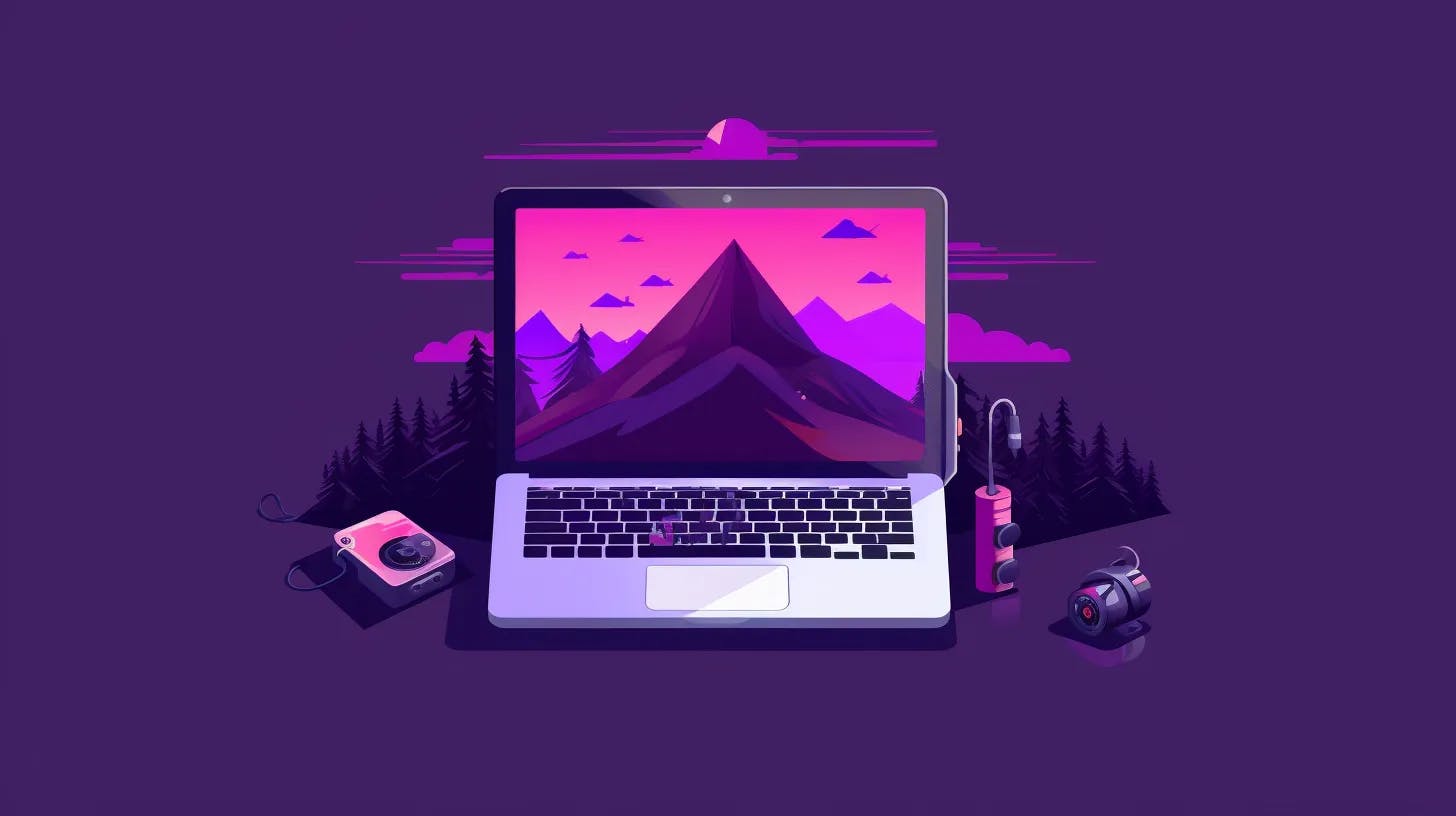
In the rapidly evolving landscape of web development, harnessing the combined power of Next.js Markdown has become a favored approach for building dynamic and efficient blogs.
With Next.js providing server-side rendering and static site generation capabilities and Markdown offering a simple yet robust markup language for content creation, developers have an arsenal of tools to craft engaging and scalable blog platforms.
Join Kapsys as we explore the intricacies of leveraging Next.js Markdown to create a seamless and customizable blogging experience.
Understanding Next.js Markdown
Before embarking on our journey to build a Next.js Markdown blog, it's essential to grasp the core concepts of both technologies.
Next.js
Next.js, a React framework, empowers developers to build web applications quickly. Its ability to handle server-side rendering, static site generation, and client-side rendering makes it a versatile choice for creating dynamic websites. Next.js Markdown simplifies the development process by providing a structured environment and optimizing performance through efficient rendering strategies.
Markdown
Markdown, a lightweight markup language, offers a simple syntax for formatting text. It allows content creators to focus on writing without the distractions of complex markup. Next.js Markdown is widely adopted for its readability and versatility, making it an ideal choice for blog posts, documentation, and other textual content.
Read: Why Choose Next.js for Your Next Web Project?
Why Use Next.js Markdown for a Blog?
Combining Next.js Markdown offers numerous benefits for building a blog:
Efficiency
Next.js's static site generation capabilities ensure fast page loads and improved SEO performance, while Markdown streamlines content creation, enabling bloggers to focus on writing compelling posts.
Flexibility
Next.js's modular architecture allows easy integration with Markdown files, offering content management and customization flexibility. Developers can leverage React components to create rich and interactive blog layouts while maintaining the simplicity of Markdown syntax.
Scalability
With Next.js's support for server-side rendering and static site generation, blogs built with Next.js Markdown are inherently scalable. As the blog grows, adding new content is as simple as creating Markdown files, ensuring seamless scalability without compromising performance.
Read: Under The Hood: How Next.js SSR Works
Before We Begin
Here are the most necessary prerequisites for building a Next.js Markdown blog:
Basic Knowledge of JavaScript: Understand variables, functions, arrays, objects, and control flow.
Understanding of React: Familiarity with React concepts like components, props, state, and JSX syntax.
Command Line Interface (CLI) Skills: Navigating directories, installing dependencies, and running commands using the command line.
HTML and CSS Knowledge: Know how to structure content with HTML and style it using CSS.
Experience with Markdown: Understanding of Markdown syntax for content creation.
Knowledge of SSG: Basic understanding of SSG concepts for optimizing performance.
Knowledge of Git and Version Control: Familiarity with Git commands for managing codebase and collaborating with others.
Text Editor or Integrated Development Environment (IDE): Use a text editor or IDE for coding, with necessary extensions for JavaScript development.
By ensuring these prerequisites are covered, you'll be ready to start building your Next.js Markdown blog efficiently.
Building a Next.js Markdown Blog: Step-by-Step
Let's dive into the step-by-step process of building a Next.js Markdown blog:
Step 1. Setting up the project
Begin by creating a new Next.js Markdown project using the command line interface. Navigate to your desired directory and execute the following command:
npx create-next-app my-blog
This will scaffold a new Next.js project named "my-blog." Next, install the necessary dependencies for managing Markdown files and blog content:
cd my-blog
npm install gray-matter remark react-markdown
These packages will facilitate parsing Markdown files and rendering Markdown content within your Next.js application.
Read: Getting Started With Next.js To Set Up A New Project
Step 2. Creating the folder structure
Organize your project's folder structure to accommodate Next.js Markdown files for blog posts. Create a dedicated directory named "posts" within your project's root directory to store Markdown files:
my-blog/
├── pages/
├── public/
├── posts/
└── ...
Define a template for rendering Markdown content within Next.js components to maintain consistency across your blog posts.
Step 3. Fetching markdown content
To retrieve Markdown content from the filesystem, utilize Next.js's data fetching methods, such as getStaticProps or getStaticPaths. In your Next.js pages or components, import the fs module to read Next.js Markdown files and the gray-matter library to parse front matter:
import fs from 'fs';
import matter from 'gray-matter';
export async function getStaticProps() {
const files = fs.readdirSync(`${process.cwd()}/posts`);
const posts = files.map((filename) => {
const markdownWithMetadata = fs.readFileSync(`posts/${filename}`).toString();
const { data, content } = matter(markdownWithMetadata);
return {
slug: filename.replace('.md', ''),
title: data.title,
date: data.date,
tags: data.tags,
content,
};
});
return {
props: {
posts,
},
};
}
This code snippet fetches Markdown content from the "posts" directory and extracts metadata such as title, date, and tags.
Step 4. Rendering markdown
Integrate Markdown content into your Next.js components using libraries like remark and react-markdown. Define a component to render Next.js Markdown content and customize the rendering process to support features such as syntax highlighting embedded media and responsive layouts:
import ReactMarkdown from 'react-markdown';
import remarkGfm from 'remark-gfm'; // For GitHub flavored Markdown
const PostPage = ({ post }) => {
return (
<div>
<h1>{post.title}</h1>
<p>Date: {post.date}</p>
<ReactMarkdown plugins={[remarkGfm]} children={post.content} />
</div>
);
};
export default PostPage;
This component renders a blog post's title, date, and content using ReactMarkdown with GitHub flavored Markdown support.
Step 5. Dynamic routing
Implement dynamic routing in Next.js to generate individual pages for each blog post. Define dynamic routes using the getStaticPaths function to generate paths for blog posts based on their slugs:
export async function getStaticPaths() {
const files = fs.readdirSync(`${process.cwd()}/posts`);
const paths = files.map((filename) => ({
params: {
slug: filename.replace('.md', ''),
},
}));
return {
paths,
fallback: false,
};
}
This code snippet generates dynamic paths for Next.js Markdown blog posts based on their filenames.
Read: How To Use Dynamic Routing For Better Scalability In Next.js
Step 6. Styling and theming
Customize the visual appearance of your blog using CSS or CSS-in-JS solutions like styled components. Define global styles and implement theming to allow users to switch between light and dark modes:
// styles/theme.js
export const lightTheme = {
body: '#FFFFFF',
text: '#000000',
};
export const darkTheme = {
body: '#333333',
text: '#FFFFFF',
};
Implement a theme context provider to manage the theme state and toggle between light and dark modes.
Step 7. Deployment
Deploy your Next.js Markdown blog to a hosting platform such as Vercel or Netlify. Configure continuous integration and deployment pipelines to automate the deployment process and ensure seamless updates to your blog. Connect your GitHub repository to your hosting platform and trigger automatic deployments on each commit.
Read: Top 10 Features Of Next js
Best Practises to Follow
When creating simple posts using Next.js Markdown, it's essential to follow best practices to ensure your blog's readability, maintainability, and scalability. Here are some key best practices to consider:
Use front matter
Incorporate front matter at the beginning of each Markdown file to store metadata such as title, date, author, and tags. This metadata can be parsed and utilized within your Next.js application to generate post listings and individual post pages dynamically.
Include images and media
Integrate images and media files into your Next.js Markdown posts using relative paths or URLs. Optimize images for the web to improve page load times and ensure a smooth user experience.
Implement syntax highlighting
If your posts contain code snippets, consider implementing syntax highlighting using libraries like react-syntax-highlighter or prism-react-renderer. This enhances readability and makes code examples more visually appealing.
Enable markdown extensions
Take advantage of Markdown extensions or plugins to enhance your content with additional features such as tables (remark-gfm), footnotes (remark-footnotes), or task lists (remark-toc).
Provide excerpts
Include brief excerpts or summaries at the beginning of your posts to give readers a preview of the content. This helps users quickly gauge the post's relevance and decide whether to read further.
Test responsiveness
Verify that your Next.js Markdown posts display correctly and are fully responsive across various devices and screen sizes. Test your blog on desktops, laptops, tablets, and smartphones to ensure a seamless reading experience for all users.
Following these best practices, you can create simple and effective Markdown posts for your Next.js blog, providing valuable content to your audience while maintaining a well-organized and scalable blogging platform.
Read: Improving Performance: Next.js Application Optimization
Conclusion
Building a blog with Next.js Markdown offers a potent combination of efficiency, flexibility, and scalability. By harnessing the power of Next.js's rendering capabilities and Markdown's simplicity, developers can create dynamic and engaging blog platforms that cater to the needs of content creators and audiences.
Next.js Markdown blog offers a versatile solution for sharing ideas and engaging with your community. Embrace the power of Next.js and Markdown today and embark on a journey to create your own dynamic blogging experience.
Keep up with Kapsys to learn all about Next.js and more!