Next.js SSG: Best Practices And Implementation Strategy
Efficiency, performance, and scalability are paramount in the rapidly evolving web development landscape. As developers seek optimal solutions, static site generation (SSG) has emerged as a game-changer, particularly with frameworks like Next.js. Join Kapsys as we explore the essence of Next.js SSG, its benefits, and how it transforms the development experience.
Thank you
We’ve received your message. Someone from our team will contact you soon by email
ContinueBack to main pageSign up to our blog to stay tuned about the latest industry news.
Understanding Static Site Generation (SSG)
Before delving into Next.js SSG, let's clarify how static site generation operates.
Static site generation creates static HTML files for a website during the build phase. This approach enables developers to generate pages in advance based on the content and structure defined in their codebase.
Consequently, users receive pre-rendered HTML files when they visit the site, enhancing performance and reducing server-side processing.
Introducing Next.js
Next.js is a popular open-source React framework used for building web applications. Vercel created it and has gained widespread adoption in the web development community due to its ease of use, powerful features, and performance optimizations.
Key features of Next.js include:
Server-side Rendering (SSR): Next.js enables server-side rendering for faster page loads and improved SEO.
Static Site Generation (Next.js SSG): It supports pre-rendering pages at build time, enhancing performance and SEO.
Routing: Next.js offers intuitive file-based routing for easy navigation and code organization.
API Routes: Seamless integration of serverless functions and backend logic.
Automatic Code Splitting: Optimizes performance by splitting code into smaller bundles.
Hot Module Replacement (HMR): Real-time updates without full page refresh.
TypeScript Support: Built-in TypeScript support for type-safe code.
CSS and Sass Support: Directly import stylesheets into components.
Read: Top 10 Features Of Next js
Benefits of Next.js SSG
As stated, Next.js supports static site generation, making it an ideal choice for building performant web applications. Here's how you can leverage Next.js SSG to its fullest potential:
Seamless integration
Next.js integrates static site generation into its framework, allowing developers to choose between static generation, server-side rendering (SSR), or a hybrid approach based on their project requirements.
This flexibility empowers developers to optimize performance without sacrificing dynamic functionality.
Improved performance
By pre-rendering pages at build time, Next.js SSG significantly enhances website performance. Users receive static HTML files, resulting in faster load times, improved SEO, and a smoother browsing experience.
Additionally, with incremental static regeneration, Next.js can update stale content in the background, ensuring the site remains fresh without compromising speed.
Dynamic content support
While static site generation is often associated with purely static content, Next.js SSG enables developers to incorporate dynamic data seamlessly.
Through data fetching methods like getStaticProps and getStaticPaths, developers can retrieve data at build time and generate static pages dynamically. This capability is particularly useful for blogs, e-commerce sites, and content-heavy applications.
Scalability and cost-efficiency
Static sites generated with Next.js are inherently scalable and cost-effective. Since they consist of static files, they can be served from content delivery networks (CDNs), reducing server load and distribution latency.
Moreover, automatic static optimization in Next.js eliminates unnecessary JavaScript from static pages, enhancing performance and reducing hosting costs.
Developer experience
Next.js SSG streamlines the development process, offering a delightful developer experience. Developers can iterate rapidly and quickly build complex applications with features like hot module replacement (HMR), TypeScript support, and built-in routing.
Additionally, the Next.js ecosystem provides a plethora of plugins and optimizations, enabling developers to customize and extend functionality effortlessly.
Getting Started with Next.js SSG
Ready to harness the power of Next.js SSG? Follow these steps to kickstart your project:
Step 1. Setting up the Next.js project
Begin by creating a new Next.js project using create-next-app. This command creates a new Next.js project with the necessary dependencies and folder structure.
npx create-next-app my-nextjs-project
cd my-nextjs-project
The create-next-app command initializes a new Next.js project in the directory my-nextjs-project. It installs all the required dependencies and sets up the folder structure for your project.
Step 2. Creating pages
Next.js uses the pages directory to determine the routes of your application. Create your pages inside this directory to define the structure of your website.
// pages/index.js
import React from 'react';
const HomePage = () => {
return (
<div>
<h1>Welcome to Next.js SSG!</h1>
<p>This is a static site generated by Next.js.</p>
</div>
);
};
export default HomePage;
In this example, we create a simple homepage for our Next.js application.
Step 3. Generating static pages
Next.js SSG allows you to pre-render pages at build time. Use getStaticProps to fetch data needed for static pages.
// pages/index.js
export async function getStaticProps() {
const data = {
title: "Welcome to Next.js SSG!",
description: "This is a static site generated by Next.js."
};
return {
props: {
data
}
};
}
The getStaticProps function is used to fetch data required for static pages. In this example, we're returning some static data for the homepage.
Read: Under The Hood: How Next.js SSR Works
Step 4. Building and running your application
Once your pages are set up and data fetching is implemented, build your Next.js SSG application and start the development server to preview your site locally.
npm run build
npm run start
These commands build your Next.js application and start a development server. You can then access your site locally to test and preview your changes.
Step 5. Deployment
Deploy your Next.js SSG application to a hosting provider to make it accessible on the internet. Popular options include Vercel, Netlify, and Heroku.
Deploying your Next.js application allows others to access your website on the internet. Follow the instructions provided by your chosen hosting provider to deploy your application successfully.
Read: How To Build A Simple Blog With Next.js Markdown
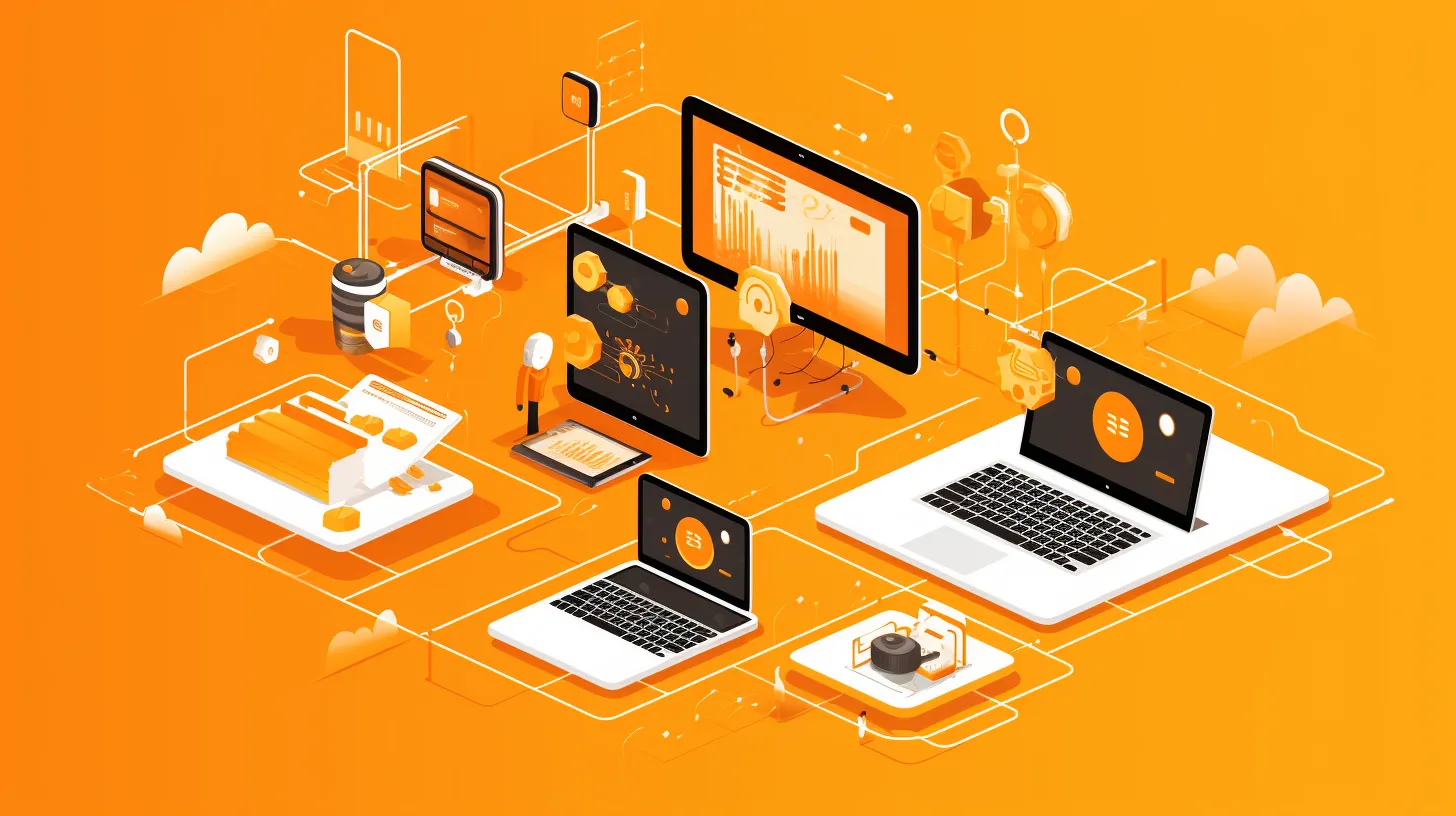
Additional Best Practices
Here are some additional best practices for implementing Next.js SSG effectively:
Data fetching optimization
Fetching data during build time using getStaticProps ensures that content is pre-rendered and readily available when users visit the page, improving performance. Implementing caching strategies helps optimize data fetching, while the revalidate option in getStaticProps allows for updating stale data without impacting user experience.
Route-based code splitting
Dynamic imports enable route-based code splitting, loading only the necessary JavaScript for each page. This reduces the initial bundle size and speeds up page load times, enhancing overall performance.
SEO optimization
Managing metadata with Next.js's Head component allows better control over titles, descriptions, and canonical URLs, improving search engine visibility. Additionally, generating a sitemap.xml file facilitates search engine indexing, making it easier for search engines to discover and crawl your site.
Image optimization
Optimizing images for the web by reducing file size and implementing lazy loading helps improve page load times, especially for pages with multiple images. By deferring the loading of images until they are needed, lazy loading enhances users' initial page load experience with Next.js SSG.
Error handling
Wrapping data-fetching components with error boundaries ensures that errors are gracefully handled, preventing the entire page from crashing if an error occurs. This improves user experience by providing a smoother browsing experience and reducing frustration.
Analytics and monitoring
Integrating analytics tools allows you to track important metrics such as page views and user interactions, providing valuable insights into user behavior. Setting up error monitoring helps identify and fix issues quickly, ensuring a seamless user experience and minimizing downtime of your Next.js SSG.
Accessibility
Conducting regular accessibility audits using tools like Lighthouse or Axe ensures that your static site is accessible to all users, including those with disabilities. Addressing accessibility issues promptly improves user experience and demonstrates a commitment to inclusivity.
Read: Improving Performance: Next.js Application Optimization
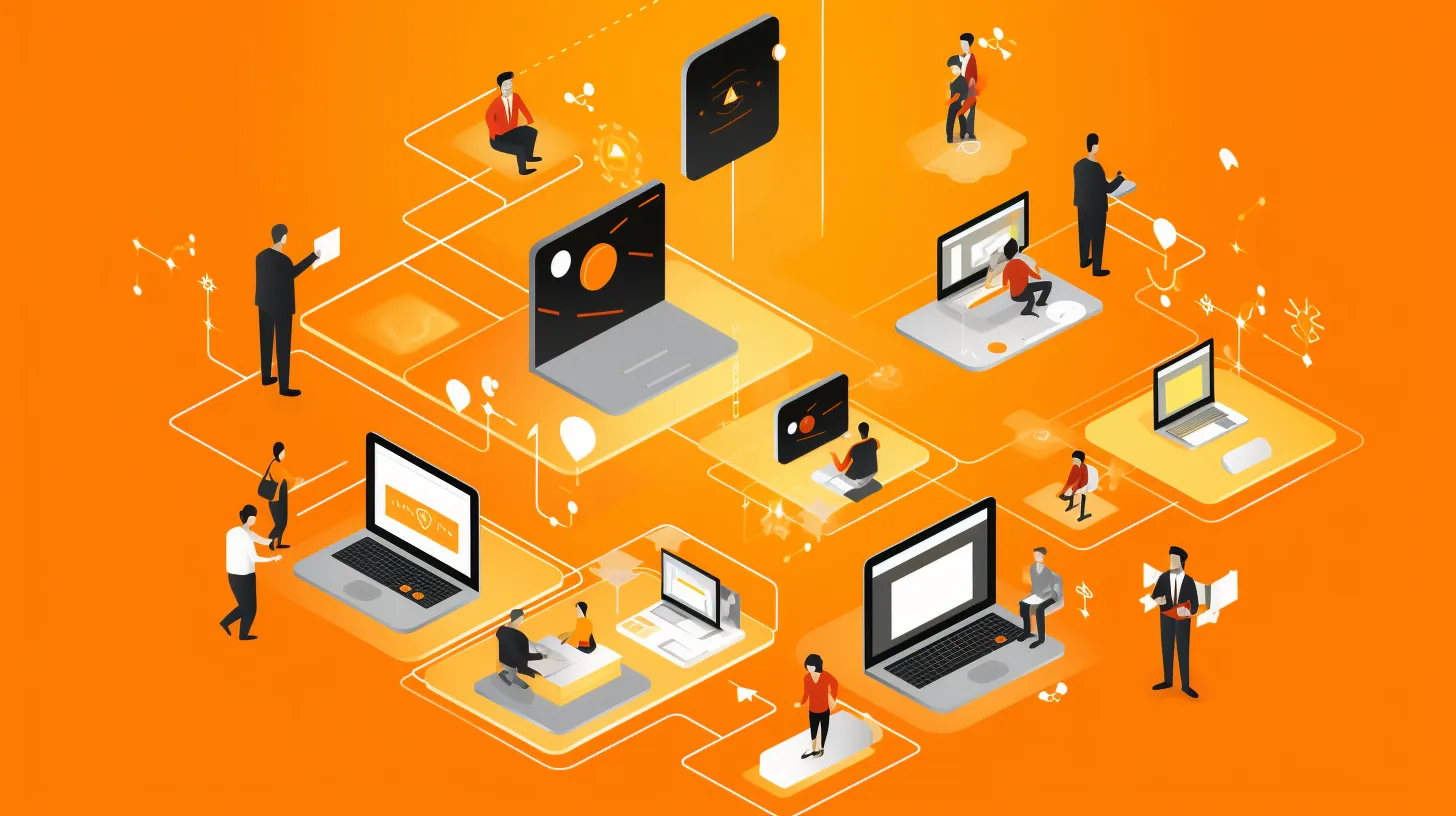
Conclusion
Next.js SSG empowers developers to build high-performance, scalable web applications with ease. By combining the flexibility of React with the efficiency of static site generation, Next.js offers a comprehensive solution for modern web development challenges.
Whether you're building a personal blog, an e-commerce platform, or a corporate website, Next.js SSG provides the tools and capabilities to elevate your project to new heights. Embrace the power of Next.js SSG and unlock limitless possibilities for your web development endeavors.
Learn all about Next.js and so much more with Kapsys!